TL;DR: Learn to use the Syncfusion .NET MAUI PDF Viewer to zoom in on specific areas of a PDF and display them in a callout for better clarity and emphasis. This guide walks you through setting up your .NET MAUI app, importing necessary namespaces, creating a toolbar with a callout toggle switch, and implementing event handlers to manage the zoom and callout functionalities.
While using PDFs, there are many areas where we need to zoom in to understand the contexts clearly. For instance, during a presentation, you may wish to zoom in on specific sections of our document to emphasize key points. When reviewing a document with colleagues or clients, you may use the zoom feature to examine specific sections or details closely.
This article will walk you through zooming and viewing the desired text or area in a PDF document in a separate callout to enhance communication and easily understand the contexts with the help of our Syncfusion .NET MAUI PDF Viewer.
Let’s get started!
Zoom and view a PDF using .NET MAUI PDF Viewer
Follow these steps to seamlessly zoom and view a specific area or text in a PDF document using the Syncfusion .NET MAUI PDF Viewer control:
Step 1: First, create a new .NET MAUI app and add Syncfusion.Maui.PdfViewer NuGet package reference to your project from NuGet Gallery. Next, in the MauiProgram.cs file, register the Syncfusion core handler.
Refer to the following code example.
using Syncfusion.Maui.Core.Hosting;
namespace PdfZoomCallout
{
public static class MauiProgram
{
public static MauiApp CreateMauiApp()
{
var builder = MauiApp.CreateBuilder();
builder
.UseMauiApp<App>()
.ConfigureFonts(fonts =>
{
fonts.AddFont("OpenSans-Regular.ttf", "OpenSansRegular");
fonts.AddFont("OpenSans-Semibold.ttf", "OpenSansSemibold");
});
builder.ConfigureSyncfusionCore();
return builder.Build();
}
}
}
Step 2: In the MainPage.xaml.cs file, import the control namespace Syncfusion.Maui.PdfViewer to display the PDF file in the .NET MAUI PDF Viewer and access its functionalities. Then, create a toolbar and add a toggle switch button to enable or disable the callout mode and add the callout image to showcase the desired area marked in a popup-like view.
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="PdfZoomCallout.MainPage"
xmlns:syncfusion="clr-namespace:Syncfusion.Maui.PdfViewer;assembly=Syncfusion.Maui.PdfViewer"
xmlns:local="clr-namespace:PdfZoomCallout">
<ContentPage.Content>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<!--Top options toolbar-->
<Border StrokeThickness="0.5" Grid.Row="0">
<Grid>
<Label Text="Syncfusion PDF Viewer" Margin="12,0,0,0" VerticalOptions="Center"/>
<HorizontalStackLayout HorizontalOptions="End" Margin="0,0,12,0">
<Switch IsToggled="False"
Toggled="CalloutMode_Toggled" HandlerChanged="Switch_HandlerChanged"/>
<Label Margin="{OnPlatform Default='8,0,0,0'}" IsVisible="{OnPlatform Default=True, WinUI=False}" Text="Callout Mode" VerticalTextAlignment="Center" VerticalOptions="Center"/>
</HorizontalStackLayout>
</Grid>
</Border>
<Grid Grid.Row="1">
<!--PDF Viewer-->
<syncfusion:SfPdfViewer x:Name="PdfViewer" DocumentSource="{Binding PdfDocumentStream}" AnnotationAdded="PdfViewer_AnnotationAdded" DocumentLoaded="PdfViewer_DocumentLoaded">
</syncfusion:SfPdfViewer>
<!--Callout view-->
<Grid BackgroundColor="#33000000" x:Name="CalloutPopup" IsVisible="False">
<Grid.GestureRecognizers>
<TapGestureRecognizer Tapped="TapGestureRecognizer_Tapped" NumberOfTapsRequired="1" />
</Grid.GestureRecognizers>
<Image x:Name="CalloutImage" VerticalOptions="Center" HorizontalOptions="Center" Margin="{OnPlatform 40, iOS=20, Android=20}">
<Image.GestureRecognizers>
<TapGestureRecognizer NumberOfTapsRequired="1" />
</Image.GestureRecognizers>
</Image>
</Grid>
</Grid>
</Grid>
</ContentPage.Content>
</ContentPage>
Step 3: In the MainPage.xaml.cs class, we will load the desired PDF document to be viewed in the constructor by assigning the document stream to the DocumentSource property.
public MainPage()
{
InitializeComponent();
PdfViewer.DocumentSource = typeof(App).GetTypeInfo().Assembly.GetManifestResourceStream("PdfZoomCallout.Assets.Pdf_Succinctly.pdf");
}
Step 4: Next, using the following event handlers of the .NET MAUI PDFViewer and toggle switch components, we will zoom in and showcase the desired area or region in a callout.
Event handlers | Details |
PdfViewer_DocumentLoaded | This event handler method will be triggered once the document is loaded in the PDF Viewer. Here, we will initialize the PdfToImageConverter library, which is a dependent one shipped along with the SfPdfViewer. This PdfToImageConverter will help us retrieve the image of the desired marked region. |
CalloutMode_Toggled | This event handler method will be triggered when the CalloutMode toggle switch is enabled or disabled. Here, we will enable or disable the rectangle annotation mode to mark the desired region in the document. |
PdfViewer_AnnotationAdded | This event handler method will be triggered once the rectangle annotation is added. Here, we will calculate the scale factor initially to return the marked region’s image without quality loss and according to the PDF Viewer control’s size. Later, we will display the marked region in a magnified callout popup view. |
Refer to the following code example.
private void PdfViewer_DocumentLoaded(object sender, EventArgs e)
{
// Initiate the image converter for the document loaded in the PDF Viewer.
imageConverter?.Dispose();
if (PdfViewer.DocumentSource is Stream documentStream)
{
documentStream.Position = 0;
Stream stream = new MemoryStream();
documentStream.CopyTo(stream);
stream.Position = 0;
imageConverter = new PdfToImageConverter(stream);
}
}
private async void CalloutMode_Toggled(object sender, ToggledEventArgs e)
{
if (e.Value == true)
{
// Enable the rectangle drawing mode.
PdfViewer.AnnotationMode = AnnotationMode.Square;
if (showHint)
{
// Show a hint for zoom and highlight a specific portion.
showHint = await DisplayAlert("Callout mode", "Callout mode is enabled. To zoom and highlight a desired area, draw a rectangle over it.", "OK", "Don't show again");
}
}
else
{
// Disable the rectangle drawing mode.
PdfViewer.AnnotationMode = AnnotationMode.None;
// Close callout popup.
CalloutImage.Source = null;
CalloutPopup.IsVisible = false;
}
}
private void PdfViewer_AnnotationAdded(object sender, Syncfusion.Maui.PdfViewer.AnnotationEventArgs e)
{
// Get the bounds of the rectangle drawn to crop and show the specific portion.
Annotation? annotation = e.Annotation;
if (annotation != null && annotation is SquareAnnotation squareAnnotation)
{
float scaleFactor = GetScaleFactor(squareAnnotation.Bounds.Width, squareAnnotation.Bounds.Height);
Stream? imageStream = imageConverter?.Convert(annotation.PageNumber - 1,
squareAnnotation.Bounds, scaleFactor);
CalloutPopup.IsVisible = true;
CalloutImage.Source = ImageSource.FromStream(() => imageStream);
PdfViewer.RemoveAnnotation(annotation);
}
}
// Returns the calculated scale factor to show the specific portion without quality loss.
float GetScaleFactor(float selectedAreaWidth, float selectedAreaHeight)
{
// Compare the size of the selected area in the PDF and callout image popup size to achieve the best quality factor.
if (selectedAreaWidth > selectedAreaHeight)
{
return (float)(PdfViewer.Bounds.Width - CalloutImage.Margin.HorizontalThickness) / selectedAreaWidth;
}
else
{
return (float)(PdfViewer.Bounds.Height - CalloutImage.Margin.VerticalThickness) / selectedAreaHeight;
}
}
Step 5: Finally, execute the application with the above code examples, and you will get the output for the Windows platform, as shown in the following image.
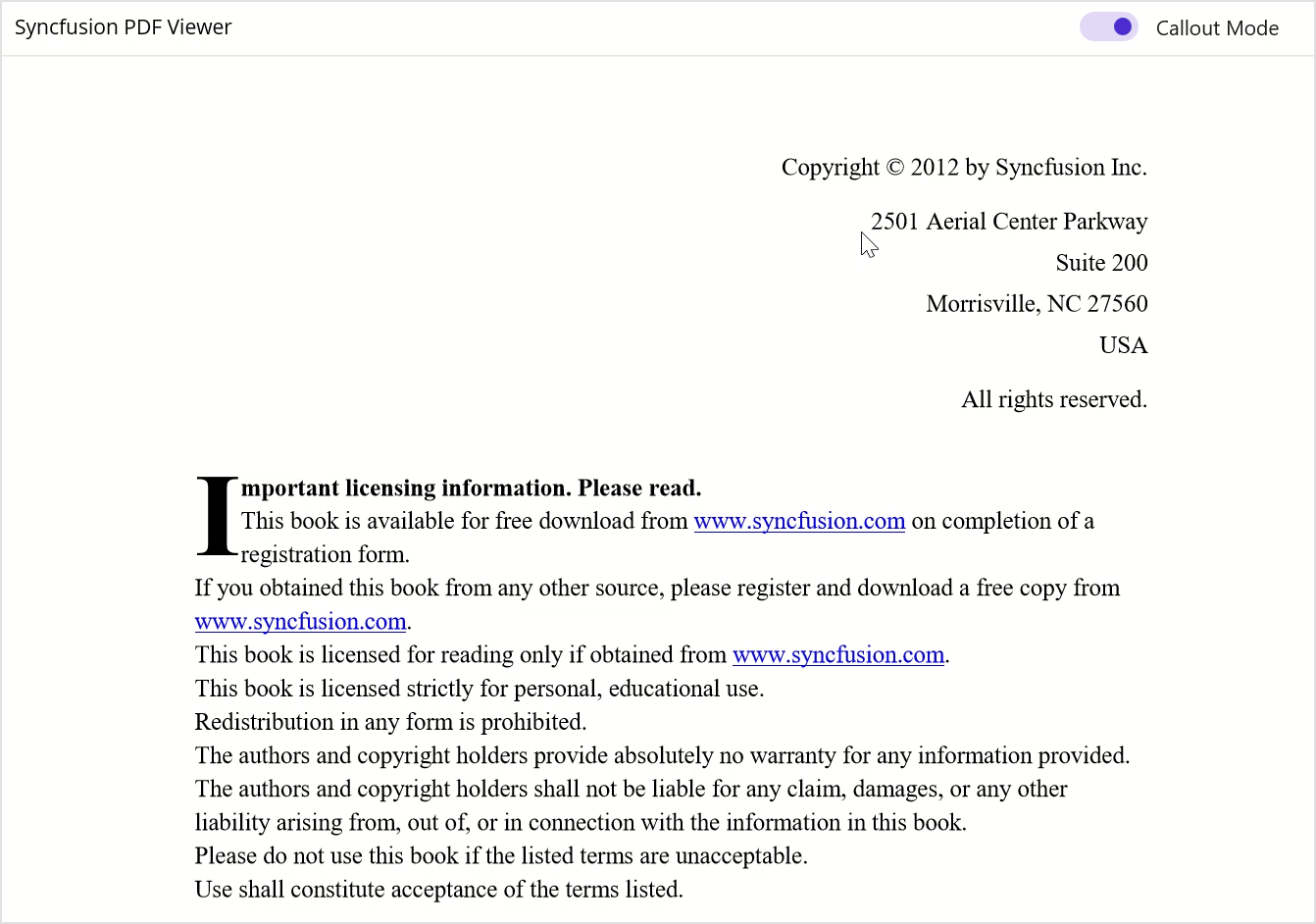
GitHub reference
For the complete working project, refer to Zoom and view the desired area in a PDF using the .NET MAUI PDF Viewer GitHub demo.

Supercharge your cross-platform apps with Syncfusion's robust .NET MAUI controls.
Conclusion
Thanks for reading! I hope you now have a clear idea about zooming and viewing the desired area in a PDF in a callout using the Syncfusion .NET MAUI PDF Viewer. Try this in your application and share your feedback in the comments below.
For current customers, the new version is available for download from the License and Downloads page. If you are not a Syncfusion customer, try our 30-day free trial to check out our newest features.
You can share your feedback and questions through the comments section below or contact us through our support forums, support portal, or feedback portal. We are always happy to assist you!