TL;DR: Boost your WPF DataGrid’s search capabilities with semantic searching! This blog shows how to use Syncfusion’s WPF DataGrid and SmartComponents.LocalEmbeddings to perform intelligent searches by converting text into numerical embeddings. Implement smart filtering to enhance search accuracy based on contextual meaning rather than exact keywords. It is ideal for improving search features in large datasets.
The Syncfusion WPF DataGrid displays and manipulates tabular data. Its rich feature set includes functionalities like data binding, editing, sorting, filtering, and grouping. It has also been optimized to work with millions of records and handle high-frequency, real-time updates.
In this blog, we’ll see how to achieve the Semantic Searching with Syncfusion WPF DataGrid.
Note: Before proceeding, refer to the WPF DataGrid getting started documentation.
What is Semantic Searching?
Traditionally, searching relies on specific keywords that match user input. In contrast, semantic searching goes beyond keywords, connecting user input with related words and their contextual meanings.
Understanding Embedding
Embedding transforms words, phrases, sentences, and other text units into numerical representations. Essentially, natural language is converted into numerical vectors known as embeddings, which capture the deeper meaning of the text.
In this guide, we will explore how to use SmartComponents.LocalEmbeddings for semantic similarity searching. This method allows us to perform similarity searches within our local environment without relying on external AI services.
Implementation smart filtering
Let’s implement smart filtering in the WPF DataGrid using semantic searching. We’ll use a schema that includes Product ID, Product Name, Availability, and Price. We’ll focus on filtering based on the Product Name property. To achieve this, we will use the DataGrid.View.Filter functionality to filter the DataGrid rows through similarity searching.
Step 1: Add package references
Include the following NuGet packages:
- SmartComponents: This package only has preview versions, so make sure to check the IncludePrerelease checkbox when installing through NuGet Package Manager.
- Syncfusion.SFGrid.WPF
Refer to the following image.
Step 2: Creating and initializing the Embedder
We need to create a LocalEmbedder instance and a new dictionary to maintain the embedder values. Here, we’ve created the ProductEmbeddings dictionary.
Refer to the following code example.
readonly static LocalEmbedder Embedder = new LocalEmbedder(caseSensitive: false); public static Dictionary<int, embeddingf32=""> ProductEmbeddings { get; set; } = new Dictionary<int, embeddingf32="">();</int,></int,>
Then, we need to compute the embeddings for our local strings and add them to this dictionary.
foreach (var expense in viewModel.ProductDetails) { ProductEmbeddings.TryAdd(expense.ProductID, Embedder.Embed($"{expense.ProductName}")); }
Here, we used the ProductName string to convert the embedding, and ProductID was used as a key value.
Step 3: Implementing similarity comparison
To compare the similarity between the strings, we used the SmartFilterRenderer class. Here, we’ve implemented the Similarity method with Embedding type parameters.
public static class SmartFilterRenderer { public static float Similarity<TEmbedding>(TEmbedding a, TEmbedding b) where TEmbedding : IEmbedding<TEmbedding> { return a.Similarity(b); } }
Step 4: Applying filtering in the WPF DataGrid
In this process, we first need to get the embedding value for the search text. Using the DataGrid.View.Filter delegate, we passed the item values with the search text vector to the SmartFilterRenderer.Similarity() method.
Refer to the following code example.
private void textBox_KeyDown(object sender, KeyEventArgs e) { if (e.Key == Key.Enter) { if (string.IsNullOrEmpty(searchtextBox.Text)) { grid.View.Filter = null; grid.View.RefreshFilter(); return; } queryVector = Embedder.Embed(searchtextBox.Text); grid.View.Filter = FilterRecords; grid.View.RefreshFilter(); } } public bool FilterRecords(object o) { var item = o as ProductInfo; if (SmartFilterRenderer.Similarity(ProductEmbeddings[item.ProductID], queryVector) > VectorValue) return true; return false; }
In a.Similarity(b), we compare the search text and ProductName vectors and return the semantic similarity value. If this value is greater than our default vector value, we return true in the FilterRecords method.
We use a default search vector value of 0.55, with its maximum value being 1. By changing the search vector values, we can obtain different outputs.
Refer to the following output image.
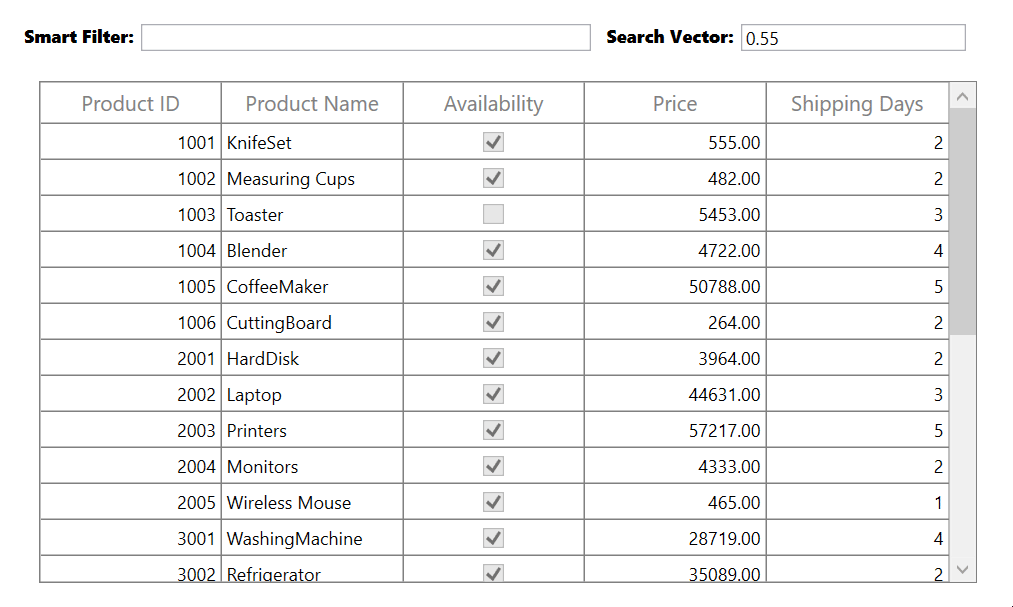
GitHub reference
For more details, refer to the semantic searching using embedding in the WPF DataGrid GitHub demo.
Conclusion
Thank you for reading! In this blog, we’ve seen how to implement semantic searching using embedding in Syncfusion WPF DataGrid. This helps you implement more advanced and meaningful search functionality in your apps. Please write in the comments section below if you have any questions or feedback.
Our WPF control examples are available in the Microsoft Store. Try them out now!
Our customers can access the latest version of Essential Studio® for WPF from the License and Downloads page. If you are not a Syncfusion customer, you can download our free evaluation to explore all our controls.
You can also contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!