TL;DR: With PDFs central to communication, maintaining document quality is key. This guide shows how to use the Syncfusion Flutter PDF Viewer to review PDFs, detect spelling errors, and mark them with squiggly lines for quick corrections. Enhance your app and boost document accuracy in a few steps!
With the growing reliance on PDFs for communication, ensuring the accuracy of documents is crucial. Reviewing and marking PDFs for spelling errors can save time and improve document quality.
In this blog, we’ll demonstrate how to review PDF documents, identify errors, and mark them with squiggly lines using the Syncfusion Flutter PDF Viewer.
Let’s get started!
Step 1: Setting up the Flutter PDF Viewer
First, we need to install the syncfusion_flutter_pdfviewer package to display PDFs in your Flutter app. Follow these steps to set up the Flutter PDF Viewer in your project.
- Add the following lines to your pubspec.yaml file to add the Syncfusion Flutter PDF Viewer dependency.
syncfusion_flutter_pdfviewer: ^xx.x.xx # Replace xx.x.xx with the latest version
- Next, we should import the following necessary packages in our Dart file.
import 'package:syncfusion_flutter_pdf/pdf.dart'; import 'package:syncfusion_flutter_pdfviewer/pdfviewer.dart';
- Then, add the SfPdfViewer widget and load the required PDF document from the asset.
SfPdfViewer.asset( 'assets/your_PDF.pdf'),
Note: For more details, refer to the getting started with Flutter PDF Viewer documentation.
Step 2: Extracting text from PDF
To perform spell-checking on a PDF document, the first essential step is to extract the text content. Once the text is extracted, we can analyze it to detect spelling errors. In this section, we’ll demonstrate how to extract text from a PDF using the PdfTextExtractor class.
We begin by accessing the PdfDocument instance of the loaded PDF using the PdfDocumentLoadedDetails. This document instance enables us to extract text, which can then be processed word by word for spell-checking.
Refer to the following code example.
SfPdfViewer.asset( 'assets/Global warming.pdf', controller: _pdfViewerController, onDocumentLoaded: (PdfDocumentLoadedDetails details) { _document = details.document; }, ),
With the help of the PdfTextExtractor and its extractTextLines method, we can extract the text lines from the document. Each line can then be broken down into individual words for further analysis.
Refer to the following code example.
final PdfTextExtractor textExtractor = PdfTextExtractor(_document!); for (int pageIndex = 0; pageIndex < _document!.pages.count; pageIndex++) { final List textLines = textExtractor.extractTextLines(startPageIndex: pageIndex); for (final TextLine textLine in textLines) { for (final TextWord textWord in textLine.wordCollection) { final String word = textWord.text; } } } }
Step 3: Implementing spell-checking functionality
After extracting text from a PDF document, the next step is implementing spell-checking.
In this example, we’ll use a simple implementation by maintaining a default dictionary of valid words to check for spelling errors. This approach can be extended by adding more words to the dictionary as needed.
A dictionary-based spell checker is straightforward and efficient for basic spell-checking tasks. It works by comparing each word in the text against a predefined list of valid words (the dictionary). If a word is not found in the dictionary, it is flagged as a potential spelling error.
Refer to the following code example to check if a word is spelled correctly by comparing it against the dictionary.
bool _checkSpellError(String word) { bool hasError = false; if (word.isEmpty || word.length == 1 || _containsOnlyNumbers(word) || _containsOnlySymbols(word) || _containsWebsite(word)) { return hasError; } final query = word.replaceAll(RegExp(r"[^\s\w]"), '').toLowerCase(); if (!words.contains(query)) { hasError = true; } return hasError; }
Step 4: Highlighting misspelled words
After identifying misspelled words in the extracted text, the next step is visually highlighting these errors in the PDF document.
Highlighting misspelled words helps users quickly identify and correct spelling errors in a document. Visual cues like squiggly lines make it easier to spot mistakes, improving the overall readability and quality of the document. This can be done by adding squiggly annotations using the Flutter PDF Viewer.
Refer to the following example. Here, we’ll highlight misspelled words by adding squiggly annotations using the PdfViewerController.addAnnotation method.
void _markSpellError(int pageIndex, TextWord textWord) { final SquigglyAnnotation squigglyAnnotation = SquigglyAnnotation( textBoundsCollection: <PdfTextLine>[ PdfTextLine( Rect.fromLTWH( textWord.bounds.left, textWord.bounds.top, textWord.bounds.width, textWord.bounds.height, ), textWord.text, pageIndex + 1, ), ], ); _pdfViewerController.addAnnotation(squigglyAnnotation); }
Refer to the following output image.
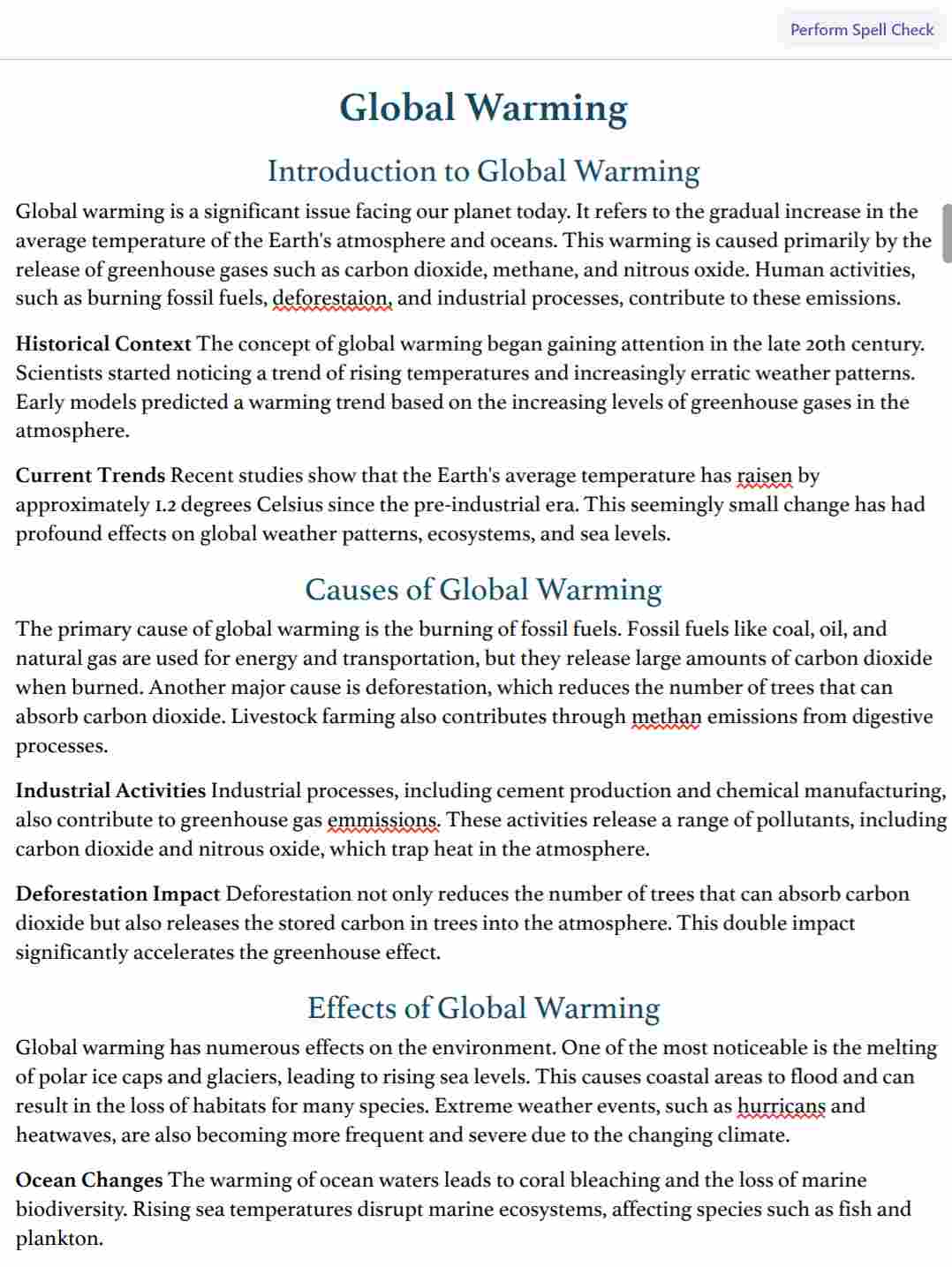
GitHub reference
Also, check out the demo for identifying and highlighting spelling errors in a PDF using Flutter PDF Viewer on GitHub.

Unlock the power of Syncfusion’s highly customizable Flutter widgets.
Conclusion
Thanks for reading! In this blog, we’ve walked through the process of creating a Flutter app that reviews PDFs, identifies spell errors, and marks them with squiggly lines using the Syncfusion Flutter PDF Viewer. By following these steps, you can enhance the quality of your documents and save time on manual proofreading.
Try out these features and share your feedback as comments on this blog. You can also reach us through our support forums, support portal, or feedback portal. We are always happy to assist you!