TL;DR: Learn how to display your current GPS location using the .NET MAUI Maps control! This guide covers integrating GPS features, adding location markers, customizing map visuals, and handling permissions across platforms. Get started with real-time location tracking today!
Displaying the GPS location is an essential feature for apps that require real-time location tracking.
The Syncfusion .NET MAUI Maps control provides robust support for integrating external imagery services like OpenStreetMap (OSM), Bing Maps, and Google Maps. It allows you to display satellite, aerial, and street maps without requiring shape files.
This blog will show you how to integrate GPS functionality into the .NET MAUI Maps control with OSM Maps and Microsoft device sensors to show your current GPS location.
Let’s get started!
Note: Before proceeding, refer to the .NET MAUI Maps documentation.
Set up the .NET MAUI Maps control
Follow these steps to integrate the .NET MAUI Maps control into your project and visualize the OpenStreetMap (OSM) tiles using it:
Step 1: Install the .NET MAUI Maps NuGet package
- In the Solution Explorer window, right-click on your .NET MAUI project and select the Manage NuGet Packages option.
- Search for the Syncfusion.Maui.Maps NuGet package and install the latest version.
- Ensure all necessary dependencies are installed, and the project is restored.
Step 2: Register the Syncfusion Core Handler
In the MauiProgram.cs file, register the Syncfusion core handler.
Refer to the following code example.
public static MauiApp CreateMauiApp() { var builder = MauiApp.CreateBuilder(); builder .UseMauiApp<App>() .ConfigureSyncfusionCore(); return builder.Build(); }
Step 3: Add the .NET MAUI Maps control
- In the XAML file, initialize the .NET MAUI Maps control.
- Set up the OSM tile provider using the UrlTemplate of the MapTileLayer class and add it to the Layer. Refer to the following code example.
xmlns:map="clr-namespace:Syncfusion.Maui.Maps;assembly=Syncfusion.Maui.Maps" <map:SfMaps> <map:SfMaps.Layer> <map:MapTileLayer x:Name="tileLayer" UrlTemplate="https://tile.openstreetmap.org/{z}/{x}/{y}.png"> </map:MapTileLayer> </map:SfMaps.Layer> </map:SfMaps>
By following these steps, you can successfully integrate the .NET MAUI Maps control into your project and display OSM tiles on the map.
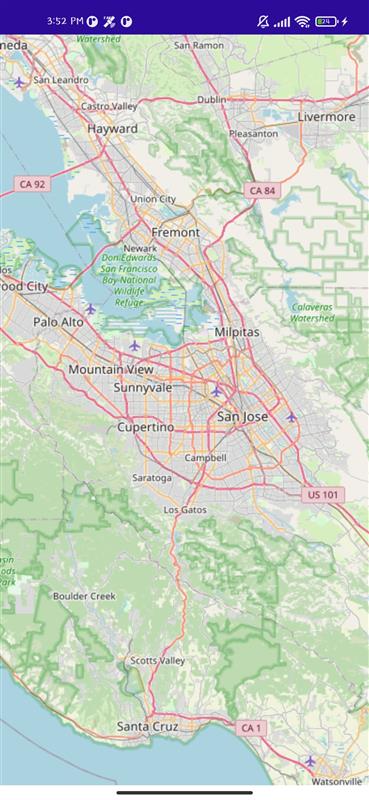
Display current GPS location in .NET MAUI Maps
Markers can be used to denote locations on the .NET MAUI Maps. Let’s follow these steps to place a map marker at the current GPS location using geocoding:
Step 1: Add a button to trigger location retrieval
In the XAML file, add a button to retrieve and display the current location. Refer to the following code example.
xmlns:buttons="clr-namespace:Syncfusion.Maui.Buttons;assembly=Syncfusion.Maui.Buttons" <buttons:SfButton x:Name="LocationButton" ImageSource="pin.png" ImageSize="25" ShowIcon="True" ImageAlignment="Default" HeightRequest="50" HorizontalOptions="Center" VerticalOptions="Center" ToolTipProperties.Text="Show my location" Background="Transparent" Style="{StaticResource SfButtonStyle}" />
Step 2: Add a marker at the current GPS location
To add a marker at a specific location, define the MapMarker class and add it to the Markers of the MapTileLayer.
Refer to the following code example to retrieve the GPS coordinates and update the marker’s Latitude and Longitude based on the current location.
this.locationButton = bindable.FindByName<SfButton>("LocationButton"); this.locationButton.Clicked += LocationButton_Clicked; private async void LocationButton_Clicked(object? sender, EventArgs e) { var status = await Permissions.RequestAsync<Permissions.LocationWhenInUse>(); if (status != PermissionStatus.Granted) { return; } Location? location = await Geolocation.GetLocationAsync(new GeolocationRequest { DesiredAccuracy = GeolocationAccuracy.High, Timeout = TimeSpan.FromSeconds(30) }); if (location != null && this.tileLayer != null) { this.tileLayer.Center = new MapLatLng(location.Latitude, location.Longitude); this.tileLayer.Markers = new MapMarkerCollection() { new MapMarker { Latitude = location.Latitude, Longitude = location.Longitude } }; } }
Step 3: Customizing markers for better visualization
You can customize the marker by using the MarkerTemplate property of the MapTileLayer, which allows you to define the template for displaying markers.
<map:MapTileLayer.MarkerTemplate> <DataTemplate> <Image Source="pin.png" Scale="1" Aspect="AspectFit" HorizontalOptions="StartAndExpand" VerticalOptions="Center" HeightRequest="35" WidthRequest="25" /> </DataTemplate> </map:MapTileLayer.MarkerTemplate>
Refer to the following image.
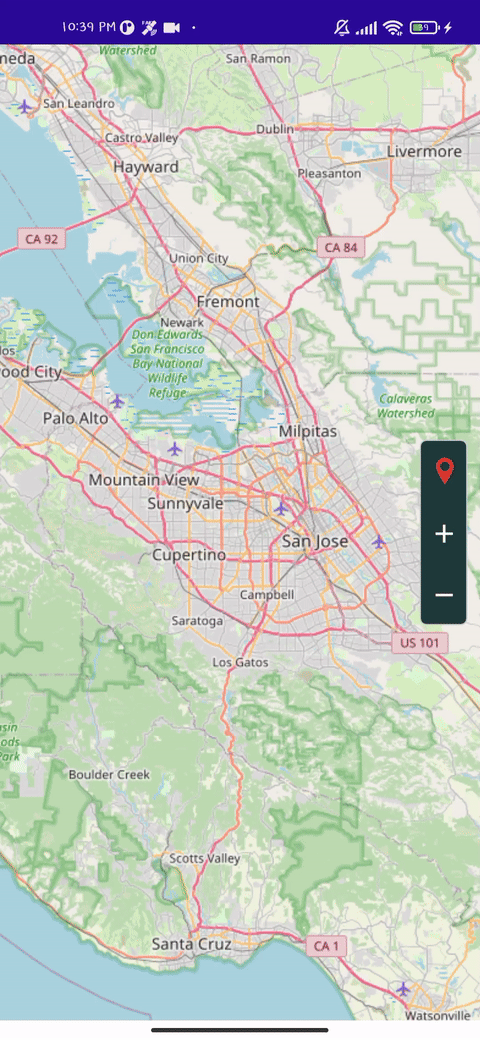
Ensuring permissions for platform-specific files
Each platform requires specific permissions to be declared.
Android (AndroidManifest.xml in Platforms/Android/)
For Android apps, add the following code inside the <manifest> tag.
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
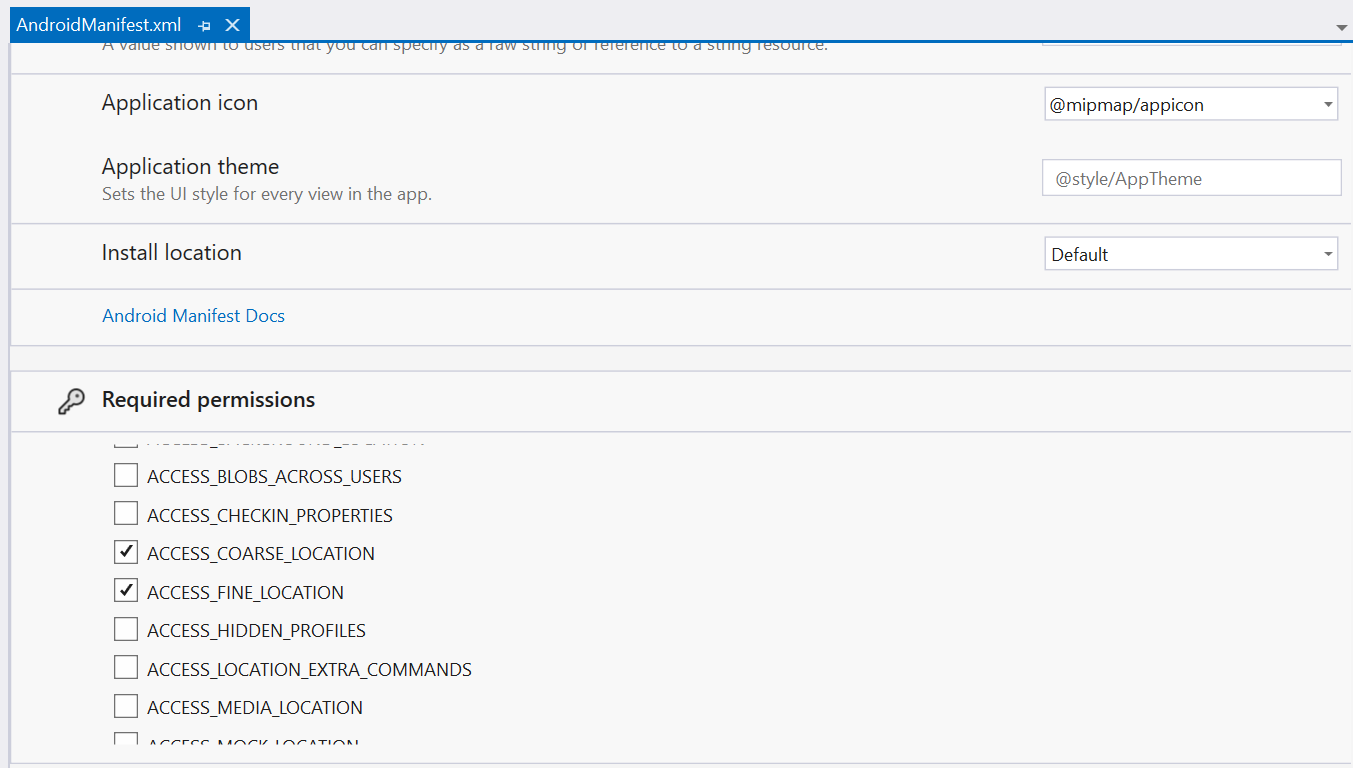
Windows (Package.appxmanifest in Platforms/Windows/)
For Windows apps, add the following code inside the <Capabilities> tag.
<DeviceCapability Name="location"/>
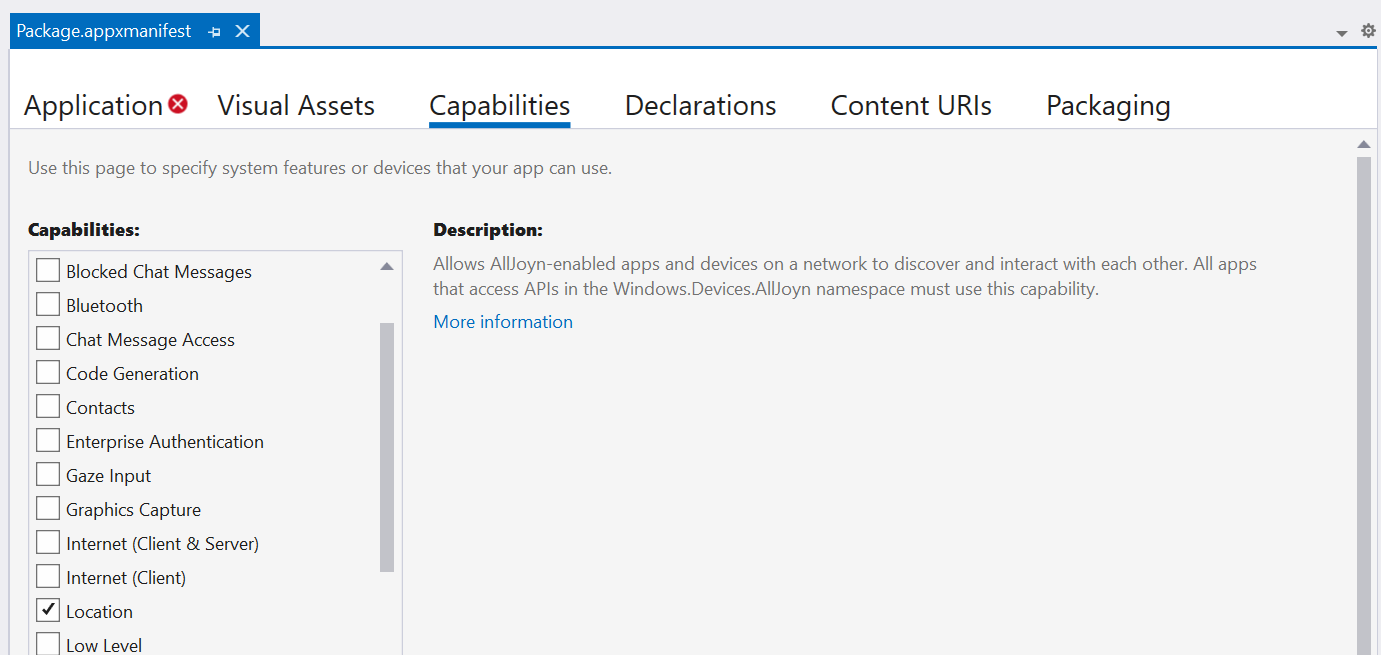
iOS (Info.plist in Platforms/iOS/)
For iOS, add the following code inside the <dict> tag.
<key>NSLocationWhenInUseUsageDescription</key> <string>We need your location to provide better services.</string> <key>NSLocationAlwaysUsageDescription</key> <string>We need your location for tracking purposes.</string>
Setting app permissions
If the issue persists, try these steps manually:
Android
Go to Settings → Apps → Select your app → Permissions → Enable Location.
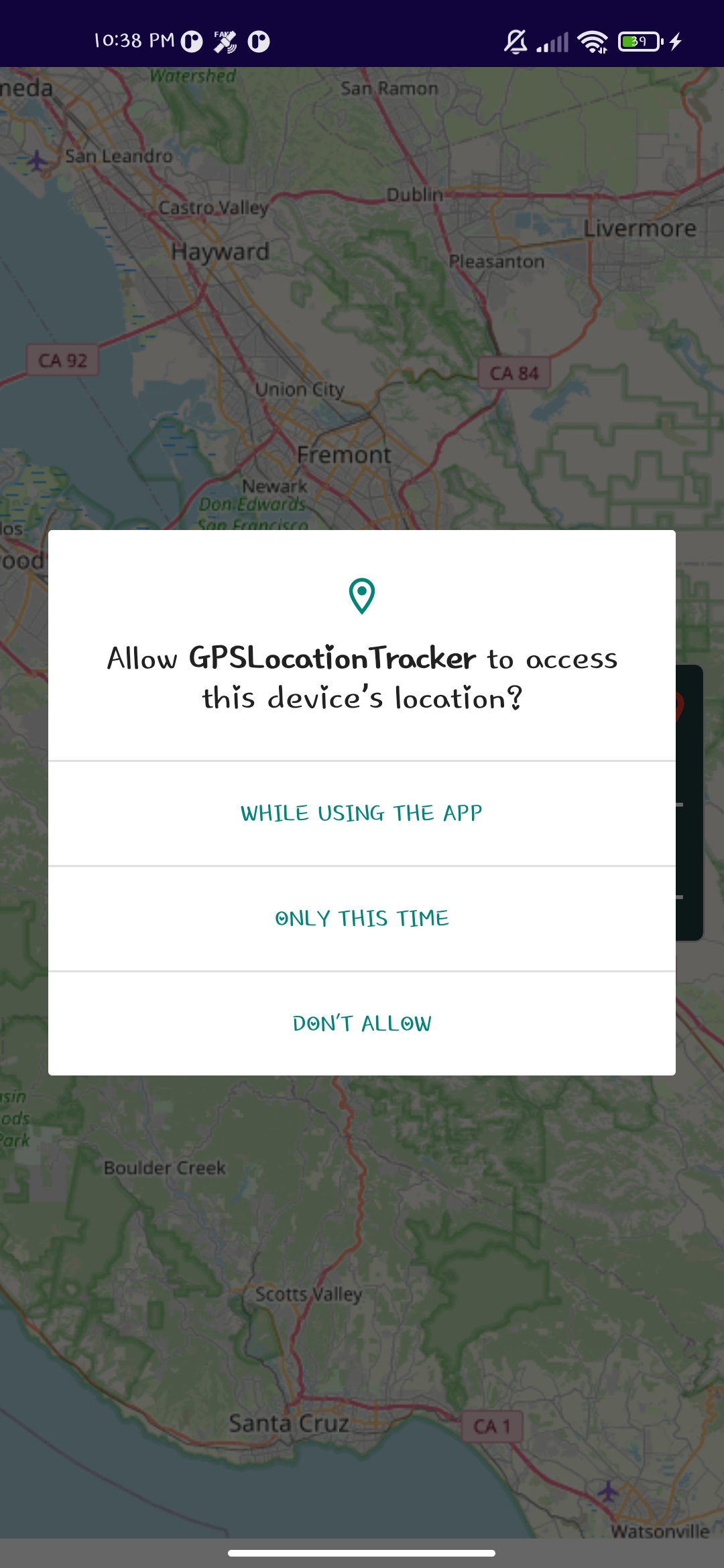
iOS
Go to Settings → Privacy & Security → Location Services → Find your app and set it to While using.
Windows
Go to Settings → Privacy & Security → Location → Enable location for your app.
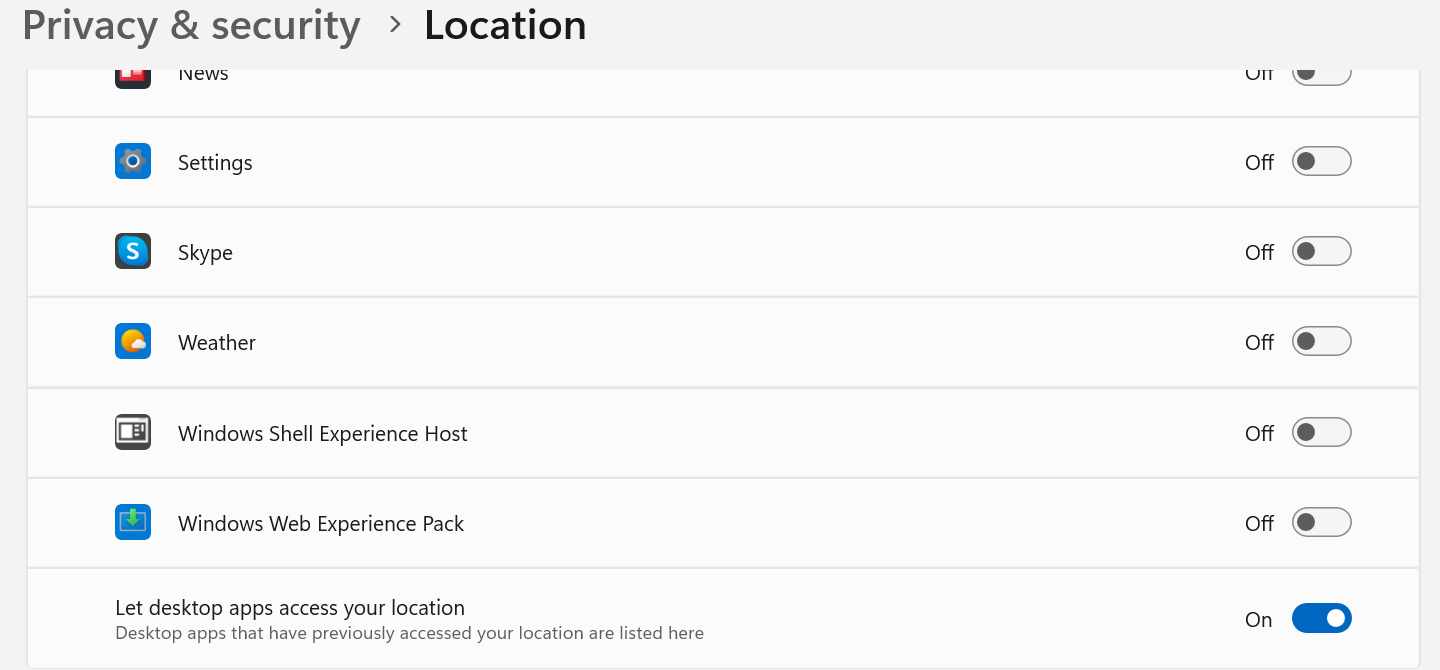
GitHub reference
For more details, download the complete example for displaying the current GPS location in the .NET MAUI Maps GitHub demo.

Supercharge your cross-platform apps with Syncfusion® robust .NET MAUI controls.
Conclusion
Thank you for reading! In this blog, we explored how to display the current GPS location in your apps using the Syncfusion .NET MAUI Maps control. Give it a try, and don’t forget to share your feedback in the comments section below!
For our customers, the latest version of Essential Studio® is available from the License and Downloads page. If you’re not a Syncfusion® customer, try our 30-day free trial to evaluate our components.
You can also contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!