TL;DR: The Syncfusion Blazor Smart Paste Button, introduced in the 2024 Volume 3 release, brings innovative AI-driven clipboard pasting functionality. This component analyzes and pastes clipboard data intelligently, ensuring content is contextually relevant and properly formatted. This blog explains how to integrate Azure OpenAI with the Smart Paste Button to enable seamless auto-filling from your Blazor apps’ clipboards.
In today’s fast-paced development landscape, streamlining workflows and maximizing productivity is more important than ever.
Syncfusion Blazor components offer robust UI tools to help developers build modern, high-performance applications. Now, taking innovation a step further, we’re glad to introduce the Syncfusion Blazor Smart Paste Button in the 2024 volume 3 release. This advanced extension of the Syncfusion Blazor Button component leverages AI to intelligently paste clipboard content, ensuring it’s seamlessly formatted and contextually accurate.
In this blog, we’ll explore its key features, how it functions, and how to easily implement it in Blazor server projects.
Why Smart Paste Button?
Traditional paste functionality is limited to copying and pasting raw data. However, developers often need to process that data by filtering, transforming, or organizing it before it’s used in an app. The Smart Paste Button addresses this by using AI to detect data types (e.g., text, numbers, or structured formats like mail and date). It provides a smart solution for pasting content more effectively and accurately, enhancing productivity and user experience.
Key features
- Clipboard integration: Pulls data from the clipboard, reducing the need for manual typing.
- Autofill capability: Automatically populates multiple form fields with one click, saving time and effort.
- Smart data parsing: Intelligently recognizes and formats clipboard data to match the form’s required fields.
- Error correction: Detects and corrects common errors in the pasted content, such as typos and grammatical mistakes, ensuring high-quality output.
- Customizable rules: Allows developers to define custom rules for how different types of content should be handled, providing flexibility and control over the pasting process.
How it works?
The Smart Paste Button Component leverages advanced AI integration to analyze and process the content being pasted. When a user pastes text into an app using this component, the AI evaluates the content, applies the necessary formatting, and integrates it smoothly into the existing forms/input elements. It automatically detects input fields (<input>, <select>, <textarea>) within the forms and auto-fills them by analyzing associated <label> tags using AI. This process not only saves time but also enhances the consistency and quality of the final output.
Benefits
- Increased efficiency: By automating the formatting and error correction processes, the Smart Paste Component significantly reduces the time and effort required to manage pasted content.
- Improved accuracy: The AI-driven analysis ensures that the content is accurately formatted and free of common errors, enhancing the overall quality of the document.
- Customization: Developers can customize the component to meet their apps’ specific needs, ensuring the pasted content adheres to the desired standards and conventions.
APIs
The Syncfusion Blazor Smart Paste Button component fully inherits all the properties, types, and styling options of the Syncfusion Blazor Button component. This means that you can leverage the existing features of the Button component while benefiting from its enhanced functionality of the Smart Paste Button.
Getting started with the Blazor Smart Paste Button
The Smart Paste Button component seamlessly integrates into a Blazor server app. Let’s walk through the process of creating and implementing it.
Note: Syncfusion Blazor Smart Components are compatible with both OpenAI and Azure OpenAI and fully support Server Interactivity mode apps.
Prerequisites
- .NET SDK: Ensure you have installed the .NET 6, .NET 8 or .NET 9 SDK.
- Blazor app: Create a new Blazor web app with Server Interactivity or Blazor Server app.
- OpenAI /Azure OpenAI Account.
Step 1: Create a new Blazor project
You can create a Blazor server app using Visual Studio via Microsoft Templates or the Syncfusion Blazor Extension.
Step 2: Install Syncfusion Blazor Smart Components and Themes NuGet in the app
To add the Blazor Smart Paste Button component in the app, open the NuGet package manager in Visual Studio (Tools → NuGet Package Manager → Manage NuGet Packages for Solution), search and install Syncfusion.Blazor.SmartComponents and Syncfusion.Blazor.Themes.
Alternatively, you can use the following package manager command to achieve the same.
Install-Package Syncfusion.Blazor.SmartComponents -Version 27.1.48 Install-Package Syncfusion.Blazor.Themes -Version 27.1.48
Step 3: Register Syncfusion Blazor service
Open the ~/_Imports.razor file and import the Syncfusion.Blazor and Syncfusion.Blazor.SmartComponents namespaces.
@using Syncfusion.Blazor @using Syncfusion.Blazor.SmartComponents
Now, register the Syncfusion Blazor service in the ~/Program.cs file of your Blazor app.
using Microsoft.AspNetCore.Components; using Microsoft.AspNetCore.Components.Web; using Syncfusion.Blazor; var builder = WebApplication.CreateBuilder(args); // Add services to the container. builder.Services.AddRazorPages(); builder.Services.AddServerSideBlazor(); builder.Services.AddSyncfusionBlazor(); var app = builder.Build(); ....
Step 4: Configure AI service
To configure the AI service, add the following settings to the ~/Program.cs file.
using Microsoft.AspNetCore.Components; using Microsoft.AspNetCore.Components.Web; using Syncfusion.Blazor; using Syncfusion.Blazor.SmartComponents; var builder = WebApplication.CreateBuilder(args); .... builder.Services.AddSyncfusionBlazor(); string apiKey = "api-key"; string deploymentName = "deployment-name"; string endpoint = "end point url"; builder.Services.AddSyncfusionSmartComponents() .ConfigureCredentials(new AIServiceCredentials(apiKey, deploymentName, endpoint)) .InjectOpenAIInference(); var app = builder.Build(); ....
In the above code example,
- apiKey: “OpenAI or Azure OpenAI API Key”;
- deploymentName: “Azure OpenAI deployment name”;
- endpoint: “Azure OpenAI deployment end point URL”;
For Azure OpenAI, first, deploy an Azure OpenAI service resource and model. Then, the values for apiKey, deploymentName, and endpoint will be provided to you after deployed.
If you are using OpenAI, create an API key, place it at apiKey, and leave the endpoint as “”. The value for deploymentName is the model you wish to use (e.g., gpt-3.5-turbo, gpt-4, etc.).
Step 5: Add stylesheet and script resources
The theme stylesheet and script can be accessed from NuGet through Static Web Assets. Reference the stylesheet and script in the <head> and <body> of the main page as follows:
- For the .NET 6 Blazor server app, include it in the ~Pages/_Layout.cshtml file.
- For the .NET 8 and .NET 9 Blazor server app, include it in the ~Components/App.razor file.
Refer to the following code example.
<head> .... <link href="_content/Syncfusion.Blazor.Themes/tailwind.css" rel="stylesheet" /> </head> …. <body> .... <script src="_content/Syncfusion.Blazor.Core/scripts/syncfusion-blazor.min.js" type="text/javascript"></script> </body>
Step 6: Add Syncfusion Blazor Smart Paste Button component
Add the Syncfusion Blazor Smart Paste Button component with form components in the ~/Pages/Index.razor file.
In this example, the Syncfusion Blazor DataForm component is used to manage the Bug Report form input fields. To get started, install the Syncfusion.Blazor.DataForm package.
@using Syncfusion.Blazor.DataForm @using System.ComponentModel.DataAnnotations @using Syncfusion.Blazor.Inputs <SfDataForm ID="MyForm" Model="@bugReportDetails" ColumnCount="3"> <FormValidator> <DataAnnotationsValidator></DataAnnotationsValidator> </FormValidator> <FormItems> <FormItem Field="@nameof(bugReportDetails.Subject)" ColumnSpan="2" Placeholder="Enter the subject" /> <FormItem Field="@nameof(bugReportDetails.Control)" ColumnSpan="1" Placeholder="Enter control name" /> <FormItem Field="@nameof(bugReportDetails.Category)" ColumnSpan="1" Placeholder="Enter category" /> <FormItem Field="@nameof(bugReportDetails.Type)" ColumnSpan="1" Placeholder="Enter bug type" /> <FormItem Field="@nameof(bugReportDetails.Priority)" ColumnSpan="1" Placeholder="Enter priority" /> <FormItem Field="@nameof(bugReportDetails.Description)" ColumnSpan="3"> <Template> <label class="e-textarea-label">Bug Description</label> <SfTextArea Placeholder="Describe the bug in detail..." /> </Template> </FormItem> <FormItem Field="@nameof(bugReportDetails.ProductVersion)" ColumnSpan="1" Placeholder="Enter product version" /> <FormItem Field="@nameof(bugReportDetails.OperatingSystem)" ColumnSpan="1" Placeholder="Enter operating system" /> <FormItem Field="@nameof(bugReportDetails.Browser)" Placeholder="Enter browser name" /> <FormItem Field="@nameof(bugReportDetails.VisualStudio)" ColumnSpan="1" Placeholder="Enter Visual Studio version" /> <FormItem Field="@nameof(bugReportDetails.BlazorProjectTemplate)" ColumnSpan="1" Placeholder="Enter Blazor project template name" /> </FormItems> <FormButtons> <SfSmartPasteButton IsPrimary="true" Content="Submit Bug Report" IconCss="e-icons e-paste" /> </FormButtons> </SfDataForm> <br/> <h5 style="text-align:center;">Sample Content</h5> @* The content presented below is for demonstration purposes only and does not represent real-time data. *@ <div> Hi, my name is Alex Johnson. I'm reporting a critical issue with the DataGrid component in the Blazor Web App. The subject of the Query is "Issue with DataGrid Rendering." I have set the priority to High due to its impact on the application's usability. The bug type is categorized as a Bug Report, as it prevents users from viewing the data properly. In my description, I've noted that the DataGrid does not render data correctly when bound to a large dataset, causing the application to freeze and display an empty grid. The issue falls under the Blazor category and specifically pertains to the DataGrid control. I am currently using Product Version 27.1.48, and I developed the application in Visual Studio 2022 on a Windows 10 operating system. I tested the application in Google Chrome and utilized the Blazor Project Template called Blazor Web App. I'm eager to see this issue resolved as it significantly hinders productivity. </div> @* For Form Decoration *@ <style> #MyForm { max-width: 800px; margin: 0 auto; padding: 20px; background-color: #f9f9f9; border-radius: 8px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); } .e-input-group.e-multi-line-input.e-auto-width { margin-left: 10px; } .e-form-label { display: block; font-weight: bold; margin: 10px 10px; } .form-item { margin-bottom: 30px; } .e-textarea-label { font-size: 14px; margin-bottom: 4px; font-weight: 600; margin-left: 10px; } .form-item input, .form-item select, .description-textarea { width: calc(100% - 20px); padding: 10px; border: 1px solid #ccc; border-radius: 4px; font-size: 16px; box-sizing: border-box; } .description-textarea { height: 100px; resize: vertical; margin-left: 0; } .form-items { display: flex; flex-wrap: wrap; gap: 30px; } .e-data-form .validation-message { margin-left: 10px; } .form-item { flex: 1 1 calc(50% - 30px); } .form-buttons { text-align: center; } .e-form-item-wrapper { width: 100%; display: flex; flex-direction: column; } .e-form-item { margin: 0px 10px; } </style> @code{ private BugReport bugReportDetails = new BugReport(); public class BugReport { [Required(ErrorMessage = "Please enter the subject.")] [Display(Name = "Subject")] public string? Subject { get; set; } [Required(ErrorMessage = "Please select a priority.")] [Display(Name = "Priority")] public string? Priority { get; set; } [Required(ErrorMessage = "Please specify the type.")] [Display(Name = "Type")] public string? Type { get; set; } = "Bug report"; [Required(ErrorMessage = "Please describe the issue.")] [Display(Name = "Description")] public string? Description { get; set; } [Required(ErrorMessage = "Please select a category.")] [Display(Name = "Category")] public string? Category { get; set; } [Required(ErrorMessage = "Please specify the control.")] [Display(Name = "Control")] public string? Control { get; set; } [Required(ErrorMessage = "Please enter the product version.")] [Display(Name = "Product Version")] public string? ProductVersion { get; set; } [Required(ErrorMessage = "Please enter the Visual Studio version.")] [Display(Name = "Visual Studio")] public string? VisualStudio { get; set; } [Required(ErrorMessage = "Please enter the operating system.")] [Display(Name = "Operating System")] public string? OperatingSystem { get; set; } [Required(ErrorMessage = "Please enter the browser.")] [Display(Name = "Browser")] public string? Browser { get; set; } [Required(ErrorMessage = "Please specify the Blazor project template.")] [Display(Name = "Blazor Project Template")] public string? BlazorProjectTemplate { get; set; } } }
Press Ctrl+F5 (Windows) or ⌘+F5 (macOS) to launch the app. This will render the Syncfusion Blazor Smart Paste Button component in your default web browser. We’ll get the output that resembles the following image.
Copy the Sample Content and click on Submit Bug Report to see how the form is instantly filled.
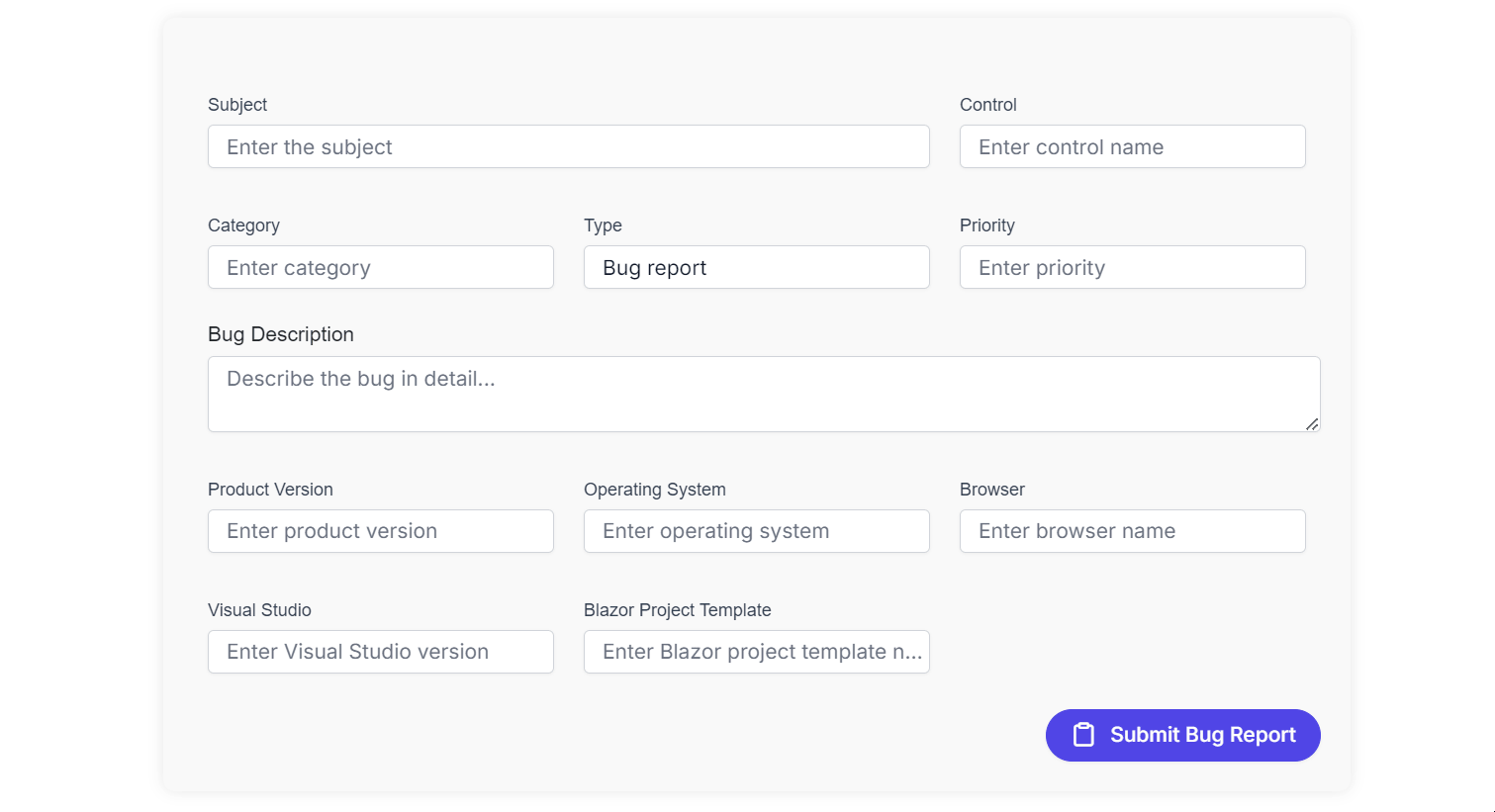
Annotation
By default, the Syncfusion Blazor Smart Paste Button analyzes form fields like <input>, <select>, and <textarea> elements, generating descriptions based on their associated <label>, name attribute, id attribute, or nearby text content. These descriptions are then sent to the back-end AI model to assist in accurately pasting the relevant data. However, for more control, you can override the default behavior by specifying custom descriptions for specific input field using the data-smartpaste-description attribute.
In the above code example, add the data-smartpaste-description attribute in the Bug Description form field.
<FormItem Field="@nameof(bugReportDetails.Description)" ColumnSpan="3"> <Template> <label class="e-textarea-label">Bug Description</label> <SfTextArea Placeholder="Describe the bug in detail..." HtmlAttributes="formatDescription" /> </Template> </FormItem> @code { Dictionary<string, object> formatDescription = new Dictionary<string, object>() { { "data-smartpaste-description", " Structure each steps in a Numbered format. " } }; }
Now run the app, copy the Sample Content, and click Submit Bug Report. Now, the date field shows as we format it using data-smartpaste-description.
The Bug Description form field has numbered content, as shown in the following output image.
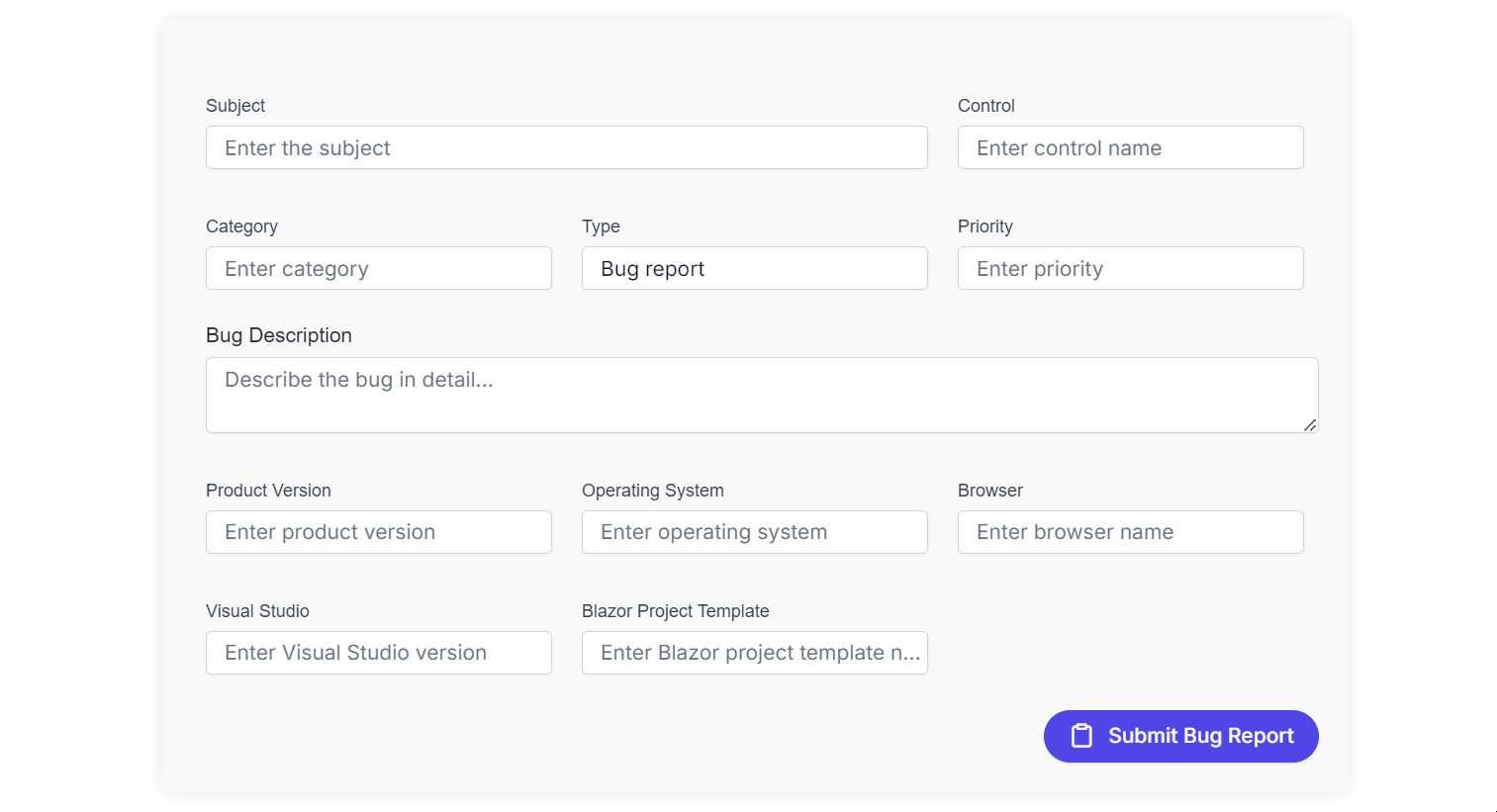
GitHub reference
For more details, refer to the Blazor Smart Paste Button component GitHub demo.

Syncfusion Blazor components can be transformed into stunning and efficient web apps.
Conclusion
Thanks for reading! The Syncfusion Blazor Smart Paste Button is a powerful tool designed to enhance user interaction by simplifying data entry. With its AI capabilities, it transforms the way users paste information, ensuring it’s accurate and contextually relevant. By implementing this component, you can significantly improve productivity and streamline workflows in your apps.
Watch this video to learn how to get started with a Blazor Smart Paste Button component, and check out here for more Syncfusion AI solutions for other supported platforms.
If you’re not a customer yet, take advantage of our 30-day free trial to explore these new features.
If you have questions, contact us through our support forum, feedback portal, or support portal. We are always happy to assist you!