TL;DR: Axios and Fetch API are popular HTTP clients. Axios offers more features and easier error handling, while Fetch API is lightweight and native to browsers. Choose Axios for complex projects and Fetch for simpler ones
Two of the most common methods for handling asynchronous HTTP requests are fetch() and Axios. Despite being a common operation, the type of request you choose to work with can have a considerable influence on the usability and overall efficiency of an app. So, it is wise to thoroughly examine them and weigh their pros and cons before choosing one.
This article will compare these two widely used tools comprehensively to help you make the best choice for your project.
What is Axios?
Axios is a promise-based, third-party HTTP client commonly seen in browser (XMLHttpRequests) and Node.js (HTTP) environments. It provides a convenient API capable of intercepting requests and responses, performing request cancellations, and automatically parsing response data to JSON format. Axios also supports client-side protection against XSRF (cross-site request forgery). You can install Axios with a package manager like npm or add it to your project via a CDN.
// NPM
npm install axios
// CDN
<script src="https://cdn.jsdelivr.net/npm/axios@1.6.7/dist/axios.min.js"></script>
Advantages of Axios
- Automatic request cancellation.
- Built-in error handling, interceptors, and clean syntax.
- Compatibility with a wide range of old and modern browsers.
- Promise-based.
Disadvantages of Axios
- External dependency.
- Large bundle size and noticeable complexity.
What is fetch()?
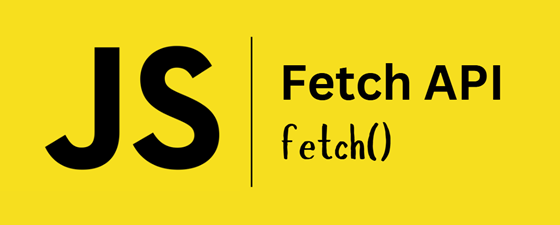
The Fetch API is also promise-based but a native JavaScript API available in all modern browsers. It is also compatible with Node.js environments and replaced the legacy XMLHttpRequests with a simpler and more contemporary approach. The Fetch API provides the fetch() method to send requests and supports multiple request and response types such as JSON, Blob, and FormData.
Advantages of fetch()
- Native browser support, so no external dependencies.
- Lightweight and more straightforward API.
- Promise-based.
- fetch() is a low-level API. So, it provides more fine-grained control.
Disadvantages of fetch()
- Limited built-in features for error handling and request time-out compared to Axios.
- Verbose code for common tasks.
Differences between Axios and fetch()
Since you now understand what Axios and fetch() are, let’s compare and contrast some key features of these two with code examples.
Basic syntax
As far as syntax is concerned, Axios delivers a more compact and developer-friendly syntax than fetch().
Simple POST request with Axios:
axios.post('https://jsonplaceholder.typicode.com/todos', {
userId: 11,
id: 201,
title: "Try Axios POST",
completed: true
})
.then(response => {
document.getElementById('output').innerHTML = `
<h2>Post Created:</h2>
<p>Title: ${response.data.title}</p>
<p>Completed status: ${response.data.completed}</p>
`;
})
.catch(error => {
console.error('Error:', error);
document.getElementById('output').innerHTML = '<p>Error creating post.</p>';
});
Similar POST request with fetch():
fetch('https://jsonplaceholder.typicode.com/todos', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ // Manually converting the object to JSON
userId: 11,
id: 202,
title: 'Try Fetch POST',
completed: true
})
})
.then(response => {
if (!response.ok) { // Manual error handling for HTTP errors
throw new Error('Error creating post');
}
return response.json(); // Manually parsing the response to JSON
});
It is quite noticeable as even though fetch() is lightweight, it still requires more manual work to perform some common tasks. For instance, Axios comes with automatic JSON parsing and can directly access the data object of the response. In contrast, in fetch(), you have to parse the response to JSON format manually. Despite both presented approaches producing similar results, you have to explicitly handle the errors, serializations, and headers in fetch().
Error handling
Handling errors is something that developers encounter almost every day. Axios, for instance, handles any HTTP call with a status code outside the 2xx range as an error by default. It gives you an explanatory object that is useful in managing and debugging your errors.
axios.get('https://jsonplaceholder.typicode.com/invalid_endpoint')
.then(response => {
console.log(response.data);
})
.catch(error => {
if (error.response) {
// Server responded with a status outside of 2xx
console.log('Error Status:', error.response.status);
console.log('Error Data:', error.response.data);
} else if (error.request) {
console.log('Error Request:', error.request);
} else {
console.log('Error Message:', error.message);
}});
fetch() does not automatically treat HTTP errors (status codes 4xx or 5xx) as errors. You have to perform conditional checks manually to identify the response status to capture the error. But you can have custom error-handling logic within your project to gather information and handle errors as Axios does.
fetch('https://jsonplaceholder.typicode.com/invalid_endpoint')
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error.message);
});
Backward compatibility with browsers
If you need compatibility with legacy dependencies, such as an older browser like Internet Explorer (IE11) or older versions of most modern browsers, your go-to solution is Axios.
fetch() is native to modern browsers and functions seamlessly with them. However, it does not support some older browser versions. You can still use it with polyfills like whatwg-fetch to get it working in browsers that do not use fetch(). It is important to note that using polyfills might increase your bundle size and affect performance, though.
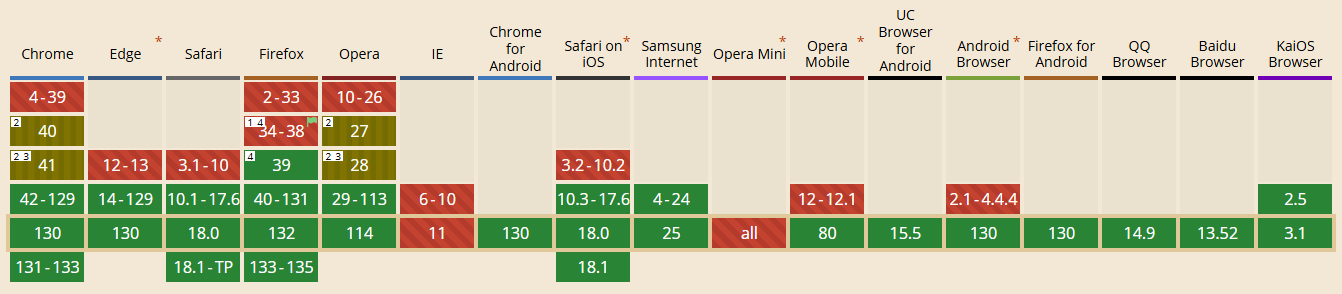
HTTP interceptors
HTTP interceptors allow the intercepting of requests and responses and come in handy when:
- Modifying requests (for example, when adding authentication to headers).
- Transforming responses (preprocessing response data).
- Handling errors globally (logging and redirecting when encountering a 401 unauthorized).
This powerful feature comes out of the box with Axios but is not natively supported by fetch().
Adding auth token to requests with Axios:
axios.interceptors.request.use(config => {
const token = 'your-token';
if (token) {
config.headers['Authorization'] = `Bearer ${token}`;
}
return config;
}, error => {
return Promise.reject(error);
});
axios.get('https://jsonplaceholder.typicode.com/todos/1')
.then(response => console.log('Data:', response.data))
.catch(error => console.error('Error:', error));
However, that does not mean fetch() cannot perform HTTP interception. You can use middleware to write a custom wrapper manually to mimic this behavior.
Adding auth token to requests with fetch() wrapper:
async function fetchWithAuth(url, options = {}) {
const token = 'your-token';
options.headers = {
...options.headers,
'Authorization': `Bearer ${token}`
};
const response = await fetch(url, options);
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
}
fetchWithAuth('https://jsonplaceholder.typicode.com/todos/1')
.then(data => console.log('Data:', data))
.catch(error => console.error('Error:', error));
Response time-out
Response time-out refers to the time a client will wait for the server to respond. If this time limit is reached, the request is counted as unsuccessful. Axios and fetch() support request time-outs, essential when dealing with unreliable or slow networks. Still, Axios takes the lead by providing a more straightforward approach to time-out management.
A request time-out with Axios:
axios.get('https://jsonplaceholder.typicode.com/todos/1', {
timeout: 3000 // (in milliseconds)
})
.then(response => {
console.log('Data:', response.data);
})
.catch(error => {
if (error.code === 'ECONNABORTED') {
console.log('Request timed out:', error.message);
} else {
console.log('Error:', error.message);
}
});
A request time-out with fetch():
const controller = new AbortController();
const signal = controller.signal;
setTimeout(() => controller.abort(), 3000);
fetch('https://jsonplaceholder.typicode.com/todos/1', { signal })
.then(response => {
if (!response.ok) {
throw new Error(HTTP error! Status: ${response.status} );
}
return response.json();
})
.then(data => {
console.log('Data:', data);
})
.catch(error => {
if (error.name === 'AbortError') {
console.log('Request timed out');
} else {
console.log('Error:', error.message);
}
});
Axios handles the time-outs more simply and gracefully with cleaner code using its time-out option. But, fetch() needs manual timeout handling with AbortController(), which gives you more control over how and when the request is aborted.
GitHub reference
For more details, refer to the complete examples for Axios vs. Fetch on the GitHub repository.
Conclusion
As with many tools, both Axios and Fetch API come with strengths and weaknesses. If you require automatic parsing of JSON, integrated error handling, and interceptors to streamline complicated processes, go with Axios. Choose fetch() if you want a pure and simple interface that is best suited for modern browser environments and does not require an external library. In short, both work well, but they are suited to different levels of complexity and functionality requirements.
Related blogs
- 5 Best Practices in Handling HTTP Errors in JavaScript
- Top 5 Chrome Extensions for Handling HTTP Requests
- How to Migrate ASP.NET HTTP Handlers and Modules to ASP.NET Core Middleware
- Efficiently Handle CRUD Actions in Syncfusion ASP.NET MVC DataGrid with Fetch Request