TL;DR: Learn how to automate flowchart creation in your React apps using Syncfusion React Diagram, eliminating the need for manual positioning. Generate flowcharts from external data, customize designs, and utilize features like zooming, panning, and serialization for enhanced interactivity. Streamline your workflows and enhance clarity with this powerful tool!
Manually assigning coordinates to flowchart elements is time-consuming and prone to errors. The Syncfusion React Diagram library provides a simplified approach with minimal configuration, enabling you to focus on your data rather than precise element positioning.
In this blog, we’ll demonstrate how to automate flowchart creation using Syncfusion’s Diagram component, eliminating manual placements by leveraging its built-in automatic layout algorithm.
What is a flowchart?
The Flowcharts are powerful tools for visually representing processes, workflows, systems, or algorithms. They use distinct symbols for various actions or steps, connected by arrows that indicate the control flow. With Syncfusion’s React Diagram, you can effortlessly generate these diagrams from external data, simplifying your workflow and enhancing clarity.
Common flowchart symbols and their meanings
Flowchart symbols are integral to visualizing different stages and operations within a process. Each symbol carries a specific meaning, representing distinct tasks, decisions, or data operations. The following table outlines the most commonly used flowchart symbols and their functions in illustrating processes.
Getting started with a simple flowchart
In this section, we’ll create a simple flowchart using the Syncfusion React Diagram library. By leveraging its built-in, high-performance layout algorithm, we can automatically arrange flowchart symbols, eliminating the need to set coordinates manually.
In addition, the diagram control supports visualizing flowcharts directly from a data source. Let’s get started
Step 1: Define the data source
To populate the flowchart in the diagram, we need to define a data source or a collection of nodes. Within the flowchart layout, you can specify the desired shape, style, labels for nodes, connectors, and decorator types for connectors directly in the data source, enhancing the UI design.
Field definitions
- name: Represents the annotation displayed on the node.
- shape: Defines the node’s shape (e.g., Terminator, Process).
- color: Specifies the fill color of the node.
- stroke: Defines the border color of the node.
- strokeWidth: Specifies the border thickness of the node.
- label: Adds annotations to the incoming connectors.
- arrowType: Determines the arrowhead type (decorator) of the incoming connector (e.g., Diamond, Fletch).
This structure allows for easy customization of the flowchart’s visual elements based on the provided data source.
The following code example defines a data source, along with custom fields that fit the flowchart requirements.
//Initializes the data source for the layout
public data = [
{
id: '1',
name: 'Start',
shape: 'Terminator',
color: '#8E44CC',
},
{
id: '2',
name: 'Open Gmail site in browser',
parentId: ['1'],
shape: 'Rectangle',
color: '#1759B7',
},
{
id: '3',
name: 'Existing \nor \n new user?',
parentId: ['2'],
shape: 'Decision',
color: '#2F95D8',
},
{
id: '4',
name: 'Create an account',
label: ['New'],
parentId: ['3'],
shape: 'Rectangle',
color: '#1759B7',
},
{
id: '5',
name: 'Sign in',
label: ['Existing'],
parentId: ['3'],
shape: 'Rectangle',
color: '#1759B7',
},
{
id: '6',
name: 'Enter username \n and password',
label: ['', 'No'],
parentId: ['5', '7'],
shape: 'Data',
color: '#70AF16',
},
{
id: '7',
name: 'Authorized?',
parentId: ['6'],
shape: 'Decision',
color: '#2F95D8',
},
{
id: '8',
name: 'Login successful!!',
label: ['Yes'],
parentId: ['7'],
shape: 'Rectangle',
color: '#1759B7',
},
{
id: '9',
name: 'Enter first name \n and last name',
parentId: ['4'],
shape: 'Data',
color: '#70AF16',
},
{
id: '10',
name: 'Enter username \n and password',
label: ['', 'Yes'],
parentId: ['9', '11'],
shape: 'Data',
color: '#70AF16',
},
{
id: '11',
name: 'Username \n already \n exists?',
parentId: ['10'],
shape: 'Decision',
color: '#2F95D8',
},
{
id: '12',
name: 'Registration Successful!!',
label: ['No'],
parentId: ['11'],
shape: 'Process',
color: '#1759B7',
},
{
id: '13',
name: 'Open inbox',
parentId: ['8', '12'],
shape: 'Process',
color: '#1759B7',
},
{
id: '14',
name: 'End',
parentId: ['13'],
shape: 'Terminator',
color: '#8E44CC',
},
];
The Parent-child relationships are essential for structuring the flowchart. Ensure each node correctly references its parent using the parentId field to maintain the logical flow.
Step 2: Binding the data source to the diagram
Once the data source is ready, bind it to the React Diagram component using DataSourceSettings. This will automatically map the nodes to the diagram and establish connections based on parent-child relationships.
Define the diagram control and bind the dataSourceSettings as follows.
<DiagramComponent
id="diagram"
ref={(diagram) => (diagramInstance = diagram)}
width={'100%'}
height={'750px'}
dataSourceSettings={{
id: 'id',
parentId: 'parentId',
dataSource: new DataManager(data),
}}
>
<Inject services={[FlowchartLayout, DataBinding]} />
</DiagramComponent>
Step 3: Customize the layout
You can tailor the layout to suit your design preferences. To create a flowchart, set the layoutType property to Flowchart. The layout class’s horizontalSpacing and verticalSpacing properties can control the spacing between nodes.
Use the margin property to add padding around the layout. These settings can also be bound to the user interface, enabling real-time updates for a more dynamic and flexible layout.
Refer to the following code example.
<DiagramComponent
id="diagram"
ref={(diagram) => (diagramInstance = diagram)}
width={'100%'}
height={'750px'}
layout={{
type: 'Flowchart',
verticalSpacing: 50,
horizontalSpacing: 50,
margin:{left:10,right:10,top:10,bottom:10}
}}>
<Inject services={[FlowchartLayout, DataBinding]} />
</DiagramComponent>
Once this code is executed, the flowchart will be automatically arranged, as shown in the following image.
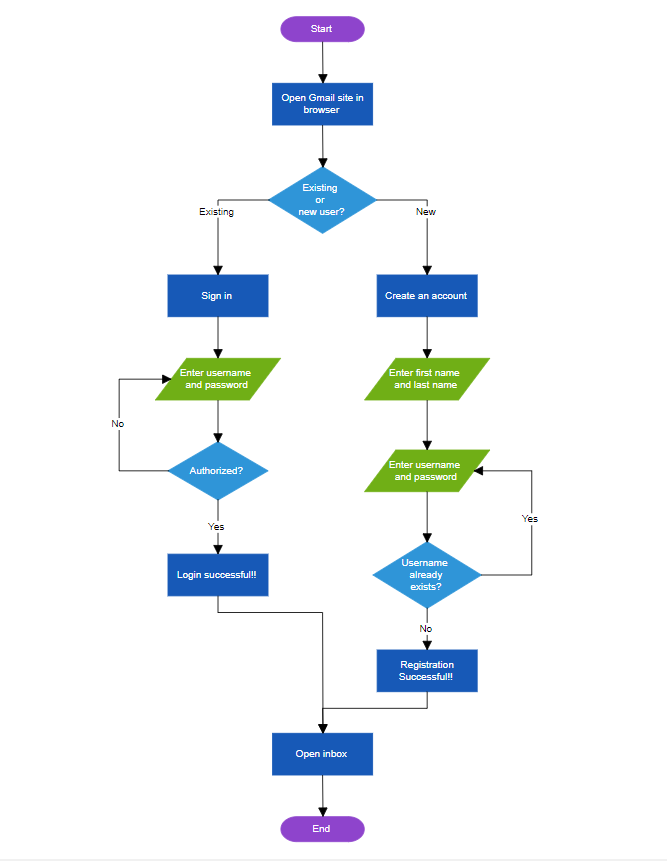
Flowchart orientation customization
The Syncfusion React Diagram library allows for easy customization of the flowchart’s orientation. Depending on your layout requirements, you can set the flow direction to vertical (TopToBottom) or horizontal (LeftToRight).
You can manage this flexibility through the orientation property of the layout class. By default, the flow direction is vertical, but you can easily change it to horizontal using the following code example.
layout={{
type: 'Flowchart',
orientation: 'TopToBottom',
}}
Refer to the following image.
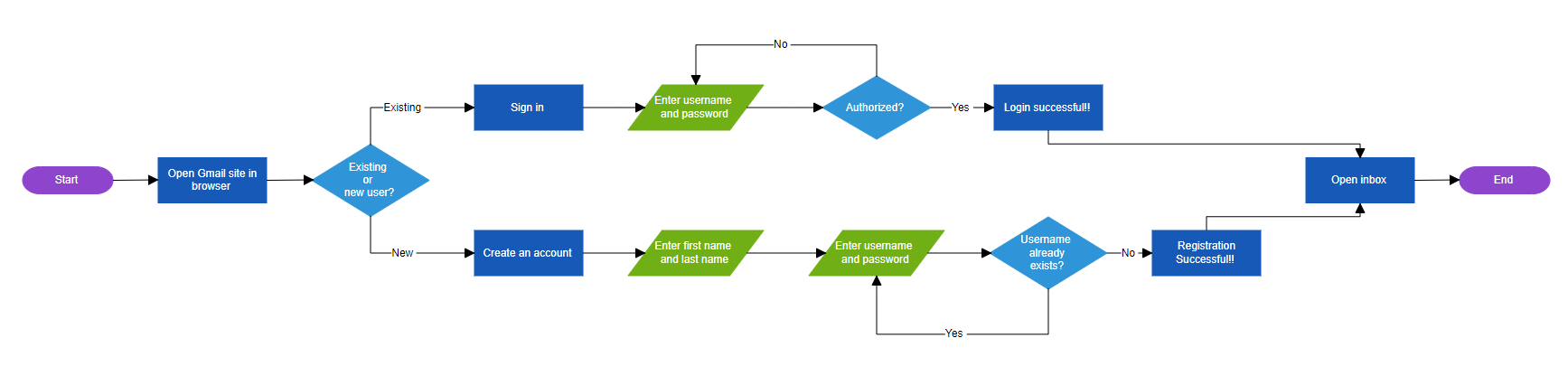
Customize the decision symbol
In flowcharts, decision symbols represent branching points where a choice is made between two paths. By default, the flowchart engine automatically determines the direction of these branches. However, with the Syncfusion React Diagram component, you can customize the direction of both the Yes and No branches to suit your needs.
You can utilize the yesBranchDirection and noBranchDirection properties of the flowchartLayoutSettings to control the path direction for each branch.
The following code example demonstrates how to customize the Yes branch to follow the flow direction while the No branch diverges to the right.
layout={{
type: 'Flowchart',
orientation: 'TopToBottom',
}}
Refer to the following image.
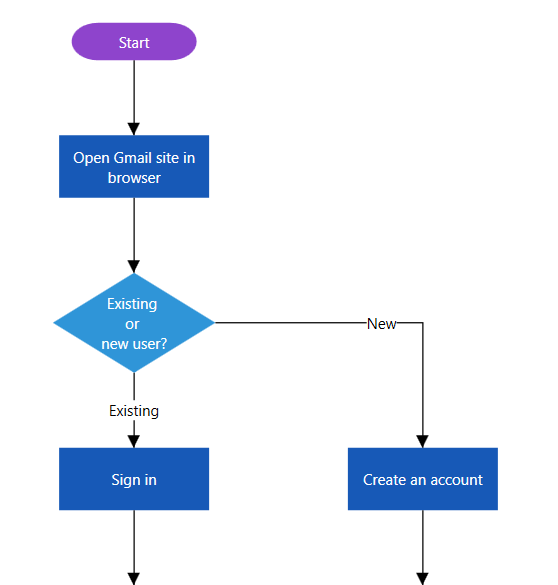
Customize the Yes and No branch line text
Decision branches are typically labeled Yes and No, but you can customize these labels to better fit your process flow better. Syncfusion’s React Diagram component allows you to define custom values for the Yes and No branches using the yesBranchValues and noBranchValues properties of the flowchartLayoutSettings.
By default, the yesBranchValues property contains Yes and True string values, while the noBranchValues property contains No and False string values.
Refer to the following code example to demonstrate how to customize the connector text to say Existing for the Yes branch and New for the No branch.
layout={{
type: 'Flowchart',
orientation: 'TopToBottom',
flowchartLayoutSettings: {
yesBranchValues: ['Existing', 'Yes'],
noBranchValues: ['New', 'No'],
},
}}
Zoom and pan
Navigating and viewing large diagrams, such as complex flowcharts, on a small screen can be quite challenging. However, the zooming and panning features simplifies this process by allowing for detailed exploration of extensive diagrams.
To enable zooming and panning, set the tool property to ZoomPan. This allows users to easily zoom in and out using the Ctrl + mouse wheel and pan by clicking and dragging the diagram.
Refer to the following code example.
<DiagramComponent
id="diagram"
ref={(diagram) => (diagramInstance = diagram)}
width={'100%'}
height={'750px'}
tool={DiagramTools.ZoomPan}
>
<Inject services={[FlowchartLayout, DataBinding]} />
</DiagramComponent>
Then, use the Ctrl + mouse wheel operation to zoom in and out of the flowchart layout.
Serialization and state persistence
Syncfusion React Diagram also provides serialization capabilities, enabling users to easily save the diagram’s state as a JSON string for later retrieval. This feature is particularly beneficial for users who need to revisit, edit, or share diagrams in the future.
A simple function call can save the diagram’s state, including nodes, connectors, and layout settings. The saveDiagram() method serializes the diagram into a JSON string, which can be stored or passed to a server for future use. Later, the loadDiagram() method restores the diagram from the saved data, allowing for seamless work continuation.
Function serialization(){
const data= diagramInstance.saveDiagram();
diagramInstance.loadDiagram(data);
}
References
For more details, refer to the Flowchart layout in React Diagram Library demos, documentation, and GitHub demo.

Explore the endless possibilities with Syncfusion’s outstanding React UI components.
Conclusion
Thanks for reading! In this blog, we have seen how to create and customize flowcharts from data without specifying coordinates using the Syncfusion React Diagram component as part of the 2024 Volume 3 release. You can also use the library to create an interactive flowchart maker application. More built-in automatic layouts, such as hierarchical and radial tree, are also available in the Diagram control.
To explore more in-depth features, refer to our help documentation on automatic layouts. Also, check out the other new updates in this 2024 volume 3 release through our Release Notes and What’s New pages.
Try out these features and share your feedback as comments on this blog. You can also reach us through our support forums, support portal, or feedback portal. We are always happy to assist you!