TL;DR: Learn how to build an AI-assisted mind-mapping app using OpenAI and React Diagram Library. This blog covers setting up a React project, integrating OpenAI for text-to-mind map conversion, and rendering interactive diagrams. The seamless workflow transforms simple text descriptions into structured visual maps. Let’s dive into the steps and create dynamic mind maps effortlessly!
Clear organization of ideas is key to effective brainstorming and project planning. Mind maps transform scattered thoughts into structured visuals, helping users grasp complex relationships at a glance. With OpenAI’s language intelligence and Syncfusion’s React Diagram, developers can effortlessly convert text descriptions into dynamic, interactive mind maps, streamlining the creative process.
Let’s see how to build an AI-assisted mind-mapping app, leveraging the strengths of both the OpenAI and React Diagram Library.
Prerequisites
Before you begin, ensure your environment is ready with the following:
- Node.js and npm are installed on your machine.
- Syncfusion React Diagram Library and AI SDKs are included in your project.
- A valid API key from OpenAI or an alternative AI provider such as Azure OpenAI.
Workflow
The workflow for creating an AI-assisted mind map involves the following key steps:
- User input: First, the user provides a textual description or keywords related to the concepts they wish to visualize.
- AI processing: Now, the input text is sent to the OpenAI, which processes it to generate a Mermaid structured data representing the mind map’s nodes and connections.
- Diagram rendering: The React Diagram component then uses the generated data to render the interactive mind map within the React app.
This seamless integration allows users to effortlessly transform their ideas into visually appealing mind maps.
Creating an AI-assisted mind map using OpenAI and React Diagram Library
Step 1: Set up the React project
Start by creating a new React project and installing the following dependencies:
Create the React project
npx create-react-app smart-mindmap cd smart-mindmap
Install the Syncfusion React Diagram and OpenAI libraries
npm install @syncfusion/ej2-react-diagrams --save npm install openai --save
Step 2: Configure the AI service
Configure the Azure OpenAI service to integrate AI capabilities by specifying the resource name, API key, and the AI model. The following code initializes the AI service and defines a utility function to handle the requests.
import { generateText } from "ai" import { createAzure } from '@ai-sdk/azure'; const azure = createAzure({ resourceName: 'RESOURCE_NAME', apiKey: 'API_KEY', }); const aiModel = azure('MODEL_NAME'); // Update the model here export async function getAzureChatAIRequest(options: any) { try { const result = await generateText({ model: aiModel, messages: options.messages, topP: options.topP, temperature: options.temperature, maxTokens: options.maxTokens, frequencyPenalty: options.frequencyPenalty, presencePenalty: options.presencePenalty, stopSequences: options.stopSequences }); return result.text; } catch (err) { console.error("Error occurred:", err); return null; } }
Ensure you replace the RESOURCE_NAME, API_KEY, and MODEL_NAME with your actual Azure AI service details.
Step 3: Configure the React Diagram library for visualization
The React Diagram is a feature-rich architecture diagram library for visualizing, creating, and editing interactive diagrams. Its intuitive API and rich feature set make creating, styling, and managing mind maps easy.
In the app.tsx file, define and configure the React Diagram component, which will be the core of our mind map generator. Refer to the following code example.
<DiagramComponent ref={diagramObj => diagram = diagramObj as DiagramComponent} id="diagram" width="100%" height="900px" selectionChange={selectionChange} historyChange={historyChange} onUserHandleMouseDown={onUserHandleMouseDown} tool={DiagramTools.Default} snapSettings={{ horizontalGridlines: gridlines, verticalGridlines: gridlines }} scrollSettings={{ scrollLimit: 'Infinity' }} layout={{ type: 'MindMap', horizontalSpacing: 80, verticalSpacing: 50, getBranch: function (node: Node) { if (node.addInfo) { var addInfo = node.addInfo; return (addInfo as any).orientation.toString(); } return 'Left'; } }} selectedItems={{ constraints: SelectorConstraints.UserHandle, userHandles: handle }} dataSourceSettings={{ id: 'id', parentId: 'parentId', dataSource: items, root: String(1), }} rulerSettings={{ showRulers: true }} scrollChange={function (args: IScrollChangeEventArgs) { if (args.panState !== 'Start') { let zoomCurrentValue: any = (document.getElementById("btnZoomIncrement") as any).ej2_instances[0]; zoomCurrentValue.content = Math.round(diagram.scrollSettings.currentZoom! * 100) + ' %'; } }} getNodeDefaults={getNodeDefaults} getConnectorDefaults={getConnectorDefaults} dragEnter={dragEnter} > <Inject services={[UndoRedo, DataBinding, PrintAndExport, MindMap]} /> </DiagramComponent>
This configuration creates a responsive diagram with a mind map layout, ruler settings, snap settings, scroll settings, and some event bindings.
Step 4: Create an AI-assist dialog for user input
Now, let’s create a dialog for AI-assisted mind map generation with suggested prompts, a textbox to get the user input, and a button to send the user input to the OpenAI.
Refer to the following code example.
<DialogComponent ref={dialogObj => dialog = dialogObj as DialogComponent} id='dialog' header='<span class="e-icons e-aiassist-chat" style="color: black;width:20px; font-size: 16px;"></span> AI Assist' showCloseIcon={true} isModal={true} content={dialogContent} target={document.getElementById('container') as HTMLElement} width='540px' visible={false} height='310px' /> const dialogContent = () => { return ( <> <p style={{ marginBottom: '10px', fontWeight: 'bold' }}>Suggested Prompts</p> <ButtonComponent id="btn1" style={{ flex: 1, overflow: 'visible', borderRadius: '8px', marginBottom: '10px' }} onClick={() => { dialog.hide(); convertTextToMindMap("Mindmap for top tourist places in the world", diagram); }} >Mindmap for top tourist places in the world</ButtonComponent> <ButtonComponent id="btn2" style={{ flex: 1, overflow: 'visible', borderRadius: '8px', marginBottom: '10px' }} onClick={() => { dialog.hide(); convertTextToMindMap("Mindmap for categories of topics in science", diagram); }} >Mindmap for categories of topics in science</ButtonComponent> <ButtonComponent id="btn3" style={{ flex: 1, overflow: 'visible', borderRadius: '8px', marginBottom: '10px' }} onClick={() => { dialog.hide(); convertTextToMindMap("Mindmap for different components in syncfusion", diagram); }} >Mindmap for different components in syncfusion</ButtonComponent> <div style={{ display: 'flex', alignItems: 'center', marginTop: '20px' }}> <TextBoxComponent type="text" id="textBox" className="db-openai-textbox" style={{ flex: 1 }} ref={textBoxObj => textBox = textBoxObj as TextBoxComponent} placeholder='Please enter your prompt here...' width={450} input={onTextBoxChange} /> <ButtonComponent id="db-send" ref={sendButtonObj => sendButton = sendButtonObj as ButtonComponent} onClick={() => { dialog.hide(); convertTextToMindMap(textBox.value, diagram) }} iconCss='e-icons e-send' isPrimary={true} disabled={true} style={{ marginLeft: '5px', height: '32px', width: '32px' }}</ButtonComponent> </div> </> ); };
This configuration in the app.tsx file sets up event listeners and handlers to manage user interactions, such as entering a prompt and triggering the conversion of text to a mind map.
Step 5: Integrating OpenAI for text-to-mind map conversion
Finally, implement the convertTextToMindMap function to transform AI-generated text into a mind map using the React Diagram library’s API.
Refer to the following code example.
export async function convertTextToMindMap(inputText: string, diagram: Diagram) { const options = { messages: [ { role: 'system', content: 'You are an assistant tasked with generating mermaid mindmap diagram data source based on user queries with space indentation' }, { role: 'user', content: `Generate only the Mermaid mindmap code for the subject titled "${inputText}". Use the format provided in the example below, but adjust the steps, shapes, and indentation according to the new title: **Example Title:** Organizational Research **Example Steps and Mermaid Code:** mindmap root(Mobile Banking Registration) User(User) PersonalInfo(Personal Information) Name(Name) DOB(Date of Birth) Address(Address) ContactInfo))Contact Information(( Email(Email) Phone(Phone Number) Account[Account] AccountType[Account Type] Savings[Savings] Checking[Checking] AccountDetails(Account Details) AccountNumber(Account Number) SortCode(Sort Code) Security{{Security}} Authentication(Authentication) Password(Password) Biometrics(Biometrics) Fingerprint(Fingerprint) FaceID(Face ID) Verification)Verification( OTP)OTP( SecurityQuestions)Security Questions( Terms(Terms & Conditions) AcceptTerms(Accept Terms) PrivacyPolicy(Privacy Policy) Note: Please ensure the generated code matches the title "${inputText}" and follows the format given above. Provide only the Mermaid mindmap code, without any additional explanations, comments, or text. ` } ], } try { const jsonResponse = await getAzureChatAIRequest(options); diagram.loadDiagramFromMermaid(jsonResponse as string); diagram.clearHistory(); } catch (error) { console.error('Error:', error); convertTextToMindMap(inputText, diagram); } };
The core function convertTextToMindMap sends a request to the OpenAI API with a user-entered prompt to generate Mermaid structured mind map data from text descriptions. Then, it uses the React Diagram’s loadDiagramFromMermaid method to render the mind map.
After executing the above code examples, we’ll get the output that resembles the following image.
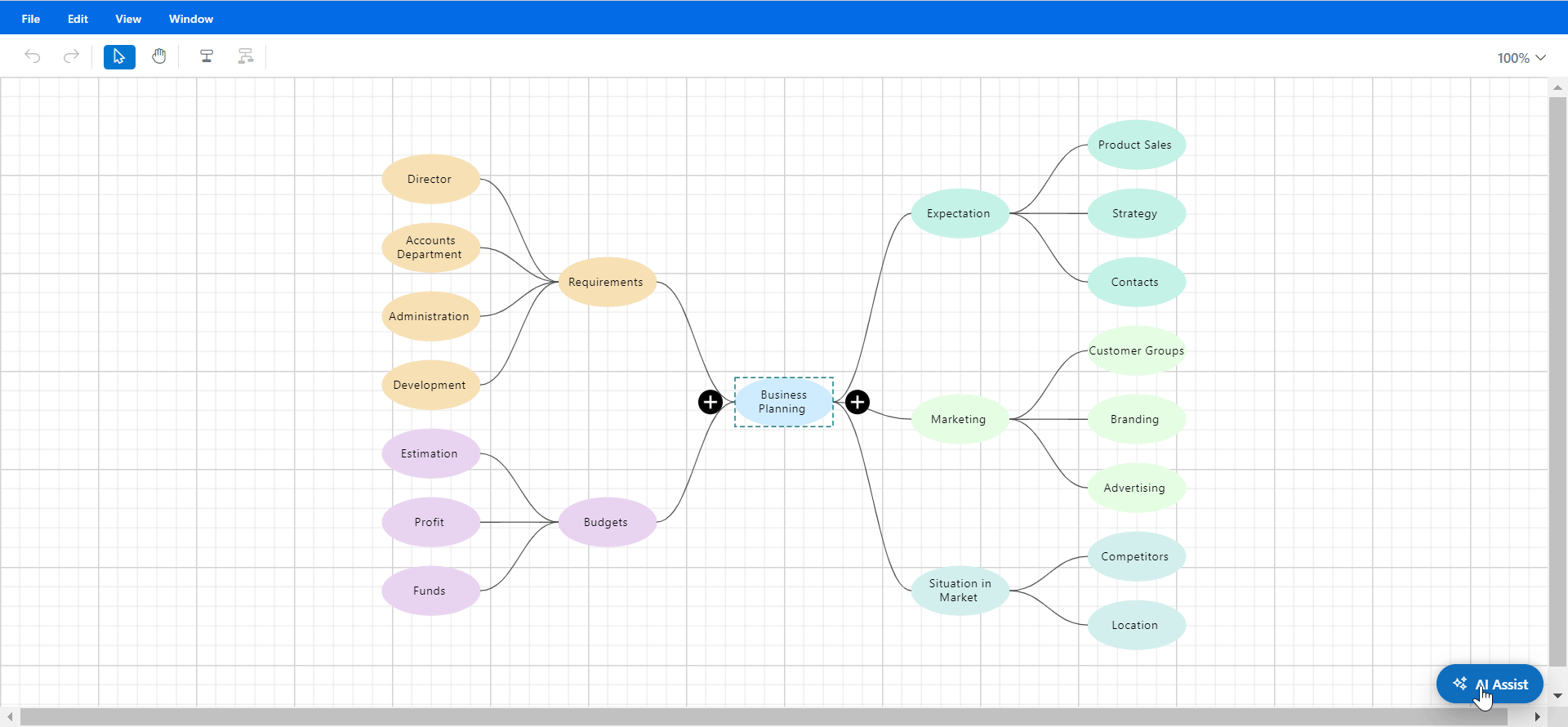
GitHub reference
For more details, refer to the AI-powered smart mind map creation using the React Diagram Library GitHub demo.

Explore the endless possibilities with Syncfusion’s outstanding React UI components.
Conclusion
Thanks for reading! In this blog, we’ve explored how to create an AI-assisted smart mind map creation app using the OpenAI and Syncfusion React Diagram Library. Try it out today and leave your feedback in the comments section given below!
Existing customers can download the latest version of Essential Studio® from the License and Downloads page. If you are not yet a customer, try our 30-day free trial to check out these new features.
You can also contact us through our support forum, feedback portal, or support portal. We are always happy to assist you!