TL;DR: Let’s see how to integrate AI Assistant into the Blazor PDF Viewer for smarter document handling. Leverage AI-powered summarization, redaction, and form-filling to automate tedious tasks. Boost productivity and simplify PDF management in your Blazor apps!
In today’s fast-paced digital environment, efficient document management is essential. PDFs play a crucial role in contracts, forms, research papers, and other documents; however, editing and managing them can often be challenging and time-consuming. This is where the PDF Viewer with AI Assistant comes in, offering a smart, efficient solution to streamline workflows, save time, and simplify working with PDFs.
By harnessing the power of artificial intelligence, Syncfusion Blazor PDF Viewer goes beyond standard document display. It supports intelligent features such as smart summarizer, smart redact, and smart form fill, transforming user experience, automating manual tasks, and boosting productivity.
Let’s see how to integrate AI Assistant in the Blazor PDF viewer to ease the PDF handling process.
Note: Before proceeding, refer to the getting started with Blazor PDF Viewer documentation.
Prerequisites
Azure OpenAI API key: Visit the Azure OpenAI website, create an account, and obtain your API key. In the Program.cs file, enter your AI key for the service, along with your endpoint and deployment name.
// Azure OpenAI Service
string apiKey = "your-api-key";
string deploymentName = "your-deployment-name";
// your-azure-endpoint-url
string endpoint = "";
Smart document summarizer in Blazor PDF Viewer: Streamlining information in the digital age
In today’s digital age, the explosion of information has made it essential to efficiently and quickly process vast amounts of text. The AI-powered document summarizer in Blazor PDF Viewer offers a powerful solution to these modern document management challenges.
This advanced tool analyzes and condenses lengthy documents into brief, focused summaries. By highlighting the document’s key points, it saves time and makes it easy to grasp essential information without reading every detail. Additionally, the document summarizer allows users to navigate directly to reference pages, providing quick access to the original context of summarized content. Users can also ask questions related to the document, and it will provide accurate answers, offering a more interactive way to explore and understand the content. This makes it even easier to dive deeper into specific details when needed, making it a necessary aid for navigating large volumes of information quickly and effectively.
Initialize the Blazor PDF Viewer
This section sets up the Blazor PDF Viewer component, specifying the DocumentPath and zoom mode for displaying the PDF file. It also attaches event handlers (DocumentLoaded, DocumentUnloaded) to manage the document’s lifecycle.
Refer to the following code example.
<SfPdfViewer2 @ref="sfPdfViewer2" DocumentPath="https://cdn.syncfusion.com/content/pdf/pdf-succinctly.pdf" ZoomMode="ZoomMode.FitToPage">
<PdfViewerEvents DocumentLoaded="DocumentLoaded" DocumentUnloaded="DocumentUnLoaded"></PdfViewerEvents>
</SfPdfViewer2>
Integrating AI service into Blazor PDF Viewer
The SummaryPDF function checks if the document has been loaded into the AI service. If not, it fetches the PDF document as a byte array, loads it into the AI-powered summarizer, and then generates a concise summary based on the AI service’s response.
Refer to the following code example.
private async Task<string> SummaryPDF()
{
string systemPrompt = "You are a helpful assistant. Your task is to analyze the provided text and generate a short summary.";
if (!isDocumentLoadedInAI)
{
byte[] bytes = await sfPdfViewer2.GetDocumentAsync();
documentStream = new MemoryStream(bytes);
await summarizer.LoadDocument(documentStream, "application/pdf");
isDocumentLoadedInAI = true;
}
//Get the summary of the PDF
return await summarizer.FetchResponseFromAIService(systemPrompt);
}
Summarizing the PDF content
This part processes the AI response by splitting the result into two parts:
- Main response
- Suggestions
It assigns the appropriate section to the args.Response property and stores the additional suggestions separately for further use.
Refer to the following code example.
var response = await summarizer.GetAnswer(args.Prompt);
// Split by "suggestions"
string[] responseArray = response.Split(new[] { "suggestions" }, StringSplitOptions.None);
if (responseArray.Length > 1)
{
args.Response = responseArray[0];
suggestions = responseArray[1];
}
Refer to the following output image.
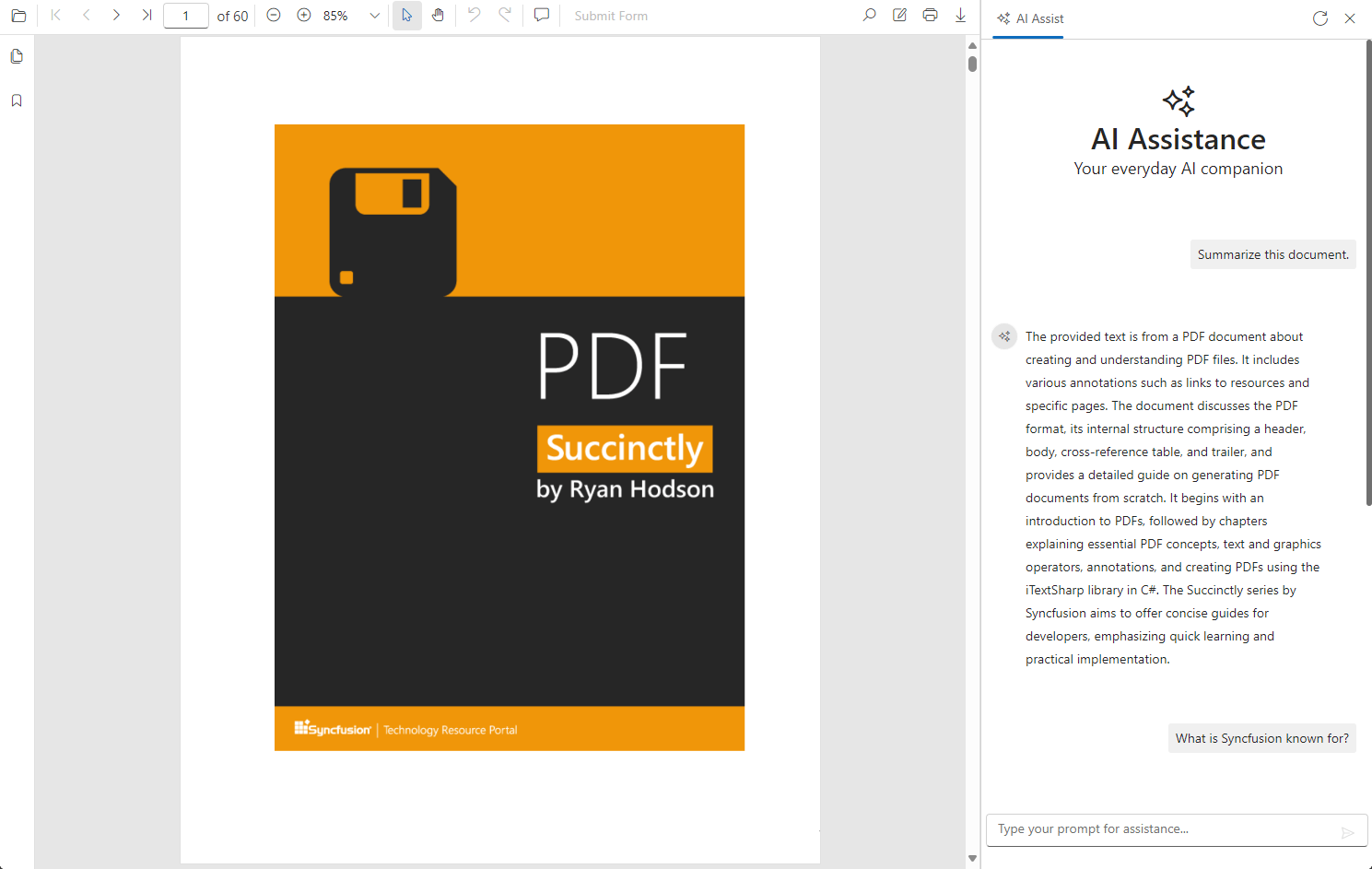
We have utilized the Blazor AI AssistView component to design the smart summarize demo. For more details, refer to its documentation and demo.
Note: Also, refer to the smart summarizer in Blazor PDF Viewer demos on the web and GitHub.
Smart redact in Blazor PDF Viewer: Safeguarding sensitive information with AI
Redaction involves removing or obscuring sensitive information in a document to ensure privacy and confidentiality.
With Blazor PDF Viewer’s smart redaction feature, users can choose specific patterns, such as emails or names, to identify them as sensitive information within a document. The AI then detects and highlights this content. If any identified details aren’t sensitive, users have the option to review and deselect them before applying the redaction. This approach ensures a streamlined and accurate process for safeguarding private data.
Manual redaction
For those who prefer a hands-on approach, manual redaction allows users to select specific content to be concealed directly. This feature offers precise control over the portions in a PDF document that need to be redacted, making it a great complement to the AI’s automated detection. By providing additional customization options, it ensures that only the desired sensitive information is protected.
Initializing the Blazor PDF Viewer
Let’s initialize the Blazor PDF Viewer with advanced settings. It links document lifecycle events (DocumentLoaded, DocumentUnLoaded) and other interaction events like AnnotationAdded, AnnotationRemoved, and ZoomChanged to handle user interactions and state changes.
Refer to the following code example.
<SfPdfViewer2 @ref="sfPdfViewer2" DocumentPath="@documentPath" Width="100%" Height="calc(100% - 40px)" rectangleSettings="@rectangleSettings" EnableToolbar="false" EnableAnnotationToolbar="false" DownloadFileName="SmartRedaction.pdf" ZoomMode="ZoomMode.FitToPage">
<PdfViewerEvents DocumentLoaded="DocumentLoaded" DocumentUnloaded="DocumentUnLoaded" AnnotationAdded="OnAddAnnotation" AnnotationRemoved="OnRemoveAnnotation" ZoomChanged="OnZoomChanged"></PdfViewerEvents>
<PdfViewerContextMenuSettings ContextMenuItems="contextMenuItems"></PdfViewerContextMenuSettings>
</SfPdfViewer2>
Integrating AI service into Blazor PDF Viewer
The LoadDocument method loads the PDF document into the AI service for processing. It retrieves the document as a byte array from the SfPdfViewer2 component, converts it into a stream, and sends it to the AI-powered redaction tool (Smart redaction). The tool extracts sensitive text from the document and returns it as a list for further processing.
Refer to the following code example.
private async Task<List<string>> LoadDocument()
{
List<string> extractedTextList = new List<string>();
if (sfPdfViewer2 != null && smartRedaction != null)
{
//Load the document to the AI and get the extracted text
byte[] bytes = await sfPdfViewer2.GetDocumentAsync();
documentStream = new MemoryStream(bytes);
extractedTextList = await smartRedaction.LoadDocument(documentStream, "application/pdf");
}
return extractedTextList;
}
Redacting sensitive information in a PDF
Let’s organize the extracted text into a hierarchical structure, grouping sensitive text nodes by pageNumber to represent the page they appear on. The grouped nodes are used to build a TreeItem structure for easy navigation. A Select All option is included to apply actions to all sensitive information at once, with an updated isDataRendered flag indicating when the data is ready for rendering in the PDF Viewer.
Refer to the following code example.
// Group childNodes by pageNumber
var groupedByPage = childNodes.GroupBy(node => node.pageNumber)
.Select(group => new TreeItem
{
NodeId = group.Key.ToString(),
NodeText = "Page " + (group.Key + 1),
pageNumber = group.Key,
Expanded = true,
Child = group.ToList(),
IsChecked = true
})
.ToList();
sensitiveInfo.Add(new TreeItem
{
NodeId = "Select All",
NodeText = $"Select All ({this.textBoundsCount})",
Expanded = true,
Child = groupedByPage
});
isDataRendered = true;
Refer to the following output image.
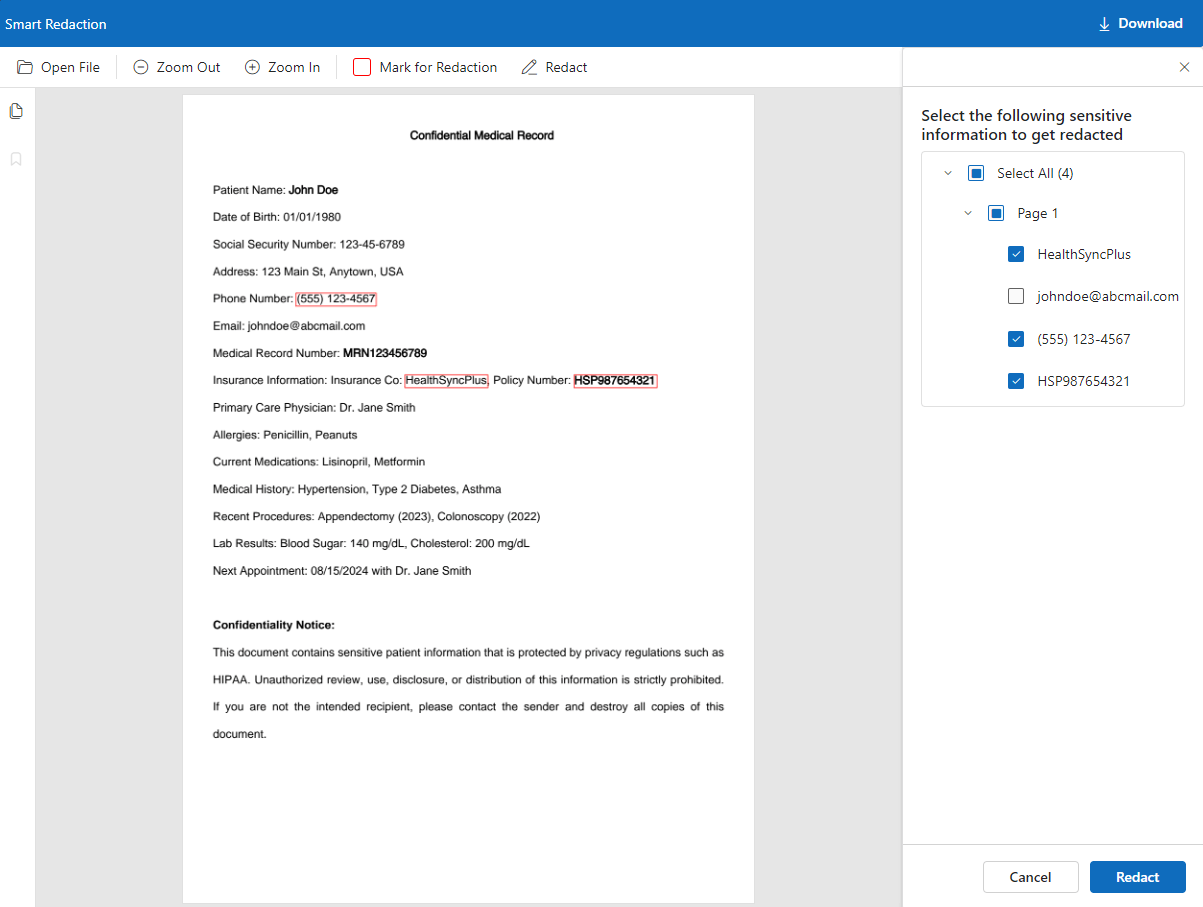
Note: For more details, refer to the smart redaction in Blazor PDF Viewer demos on the web and GitHub.
Smart form fill in Blazor PDF Viewer: Enhancing data entry with AI
Smart form fill is an AI-powered tool designed to simplify and improve how information is entered into forms. Leveraging advanced technology, it streamlines data entry by making it faster and more accurate through auto-completion in PDF forms.
This feature utilizes AI to enhance the process of filling out forms. It can analyze the content from the clipboard, automatically populate related fields based on context, validate data for accuracy, and offer suggestions for corrections. This feature significantly reduces manual effort and enhances data consistency in document management.
Initialize the Blazor PDF Viewer
Let’s set up the Blazor PDF Viewer component inside a scrollable division with a custom height (ViewerHeight). Event handlers and context menu settings are also attached to handle document-loading events and control the viewer’s context menu.
Refer to the following code example.
<div style="overflow:auto;height:@ViewerHeight;">
<SfPdfViewer2 @ref="sfPdfViewer2" DocumentPath="@documentPath" Width="100%" Height="100%" EnableToolbar="false" EnableAnnotationToolbar="false" DownloadFileName="SmartFill.pdf" ZoomMode="ZoomMode.FitToPage">
<PdfViewerEvents DocumentLoaded="DocumentLoaded"></PdfViewerEvents>
<PdfViewerContextMenuSettings ContextMenuItems="contextMenuItems"></PdfViewerContextMenuSettings>
</SfPdfViewer2>
</div>
Integrating AI service into Blazor PDF Viewer
The GetCompletionAsync method sends a prompt to the AI service, requesting it to process and return the result as an XFDF (XML Forms Data Format) string. This string represents form field data that can be directly imported into the PDF Viewer.
Refer to the following code example.
// Reuest to AI
string resultantXfdfFile = await openAIService.GetCompletionAsync(mergePrompt, false);
Smart form filling using Blazor PDF Viewer
Let’s process the XFDF data returned by the AI service. Then, convert the string into a MemoryStream and import it into the Blazor PDF Viewer using the ImportFormFieldsAsync API. This allows the form field data to be populated in the PDF Viewer, updating the document with AI-generated content.
Refer to the following code example.
// Convert the string directly to a MemoryStream
using (MemoryStream inputFileStream = new MemoryStream(Encoding.UTF8.GetBytes(resultantXfdfFile)))
{
// Import the form field data as XFDF
if (sfPdfViewer2 != null)
{
await sfPdfViewer2.ImportFormFieldsAsync(inputFileStream, FormFieldDataFormat.Xfdf);
}
}
Refer to the following output image.
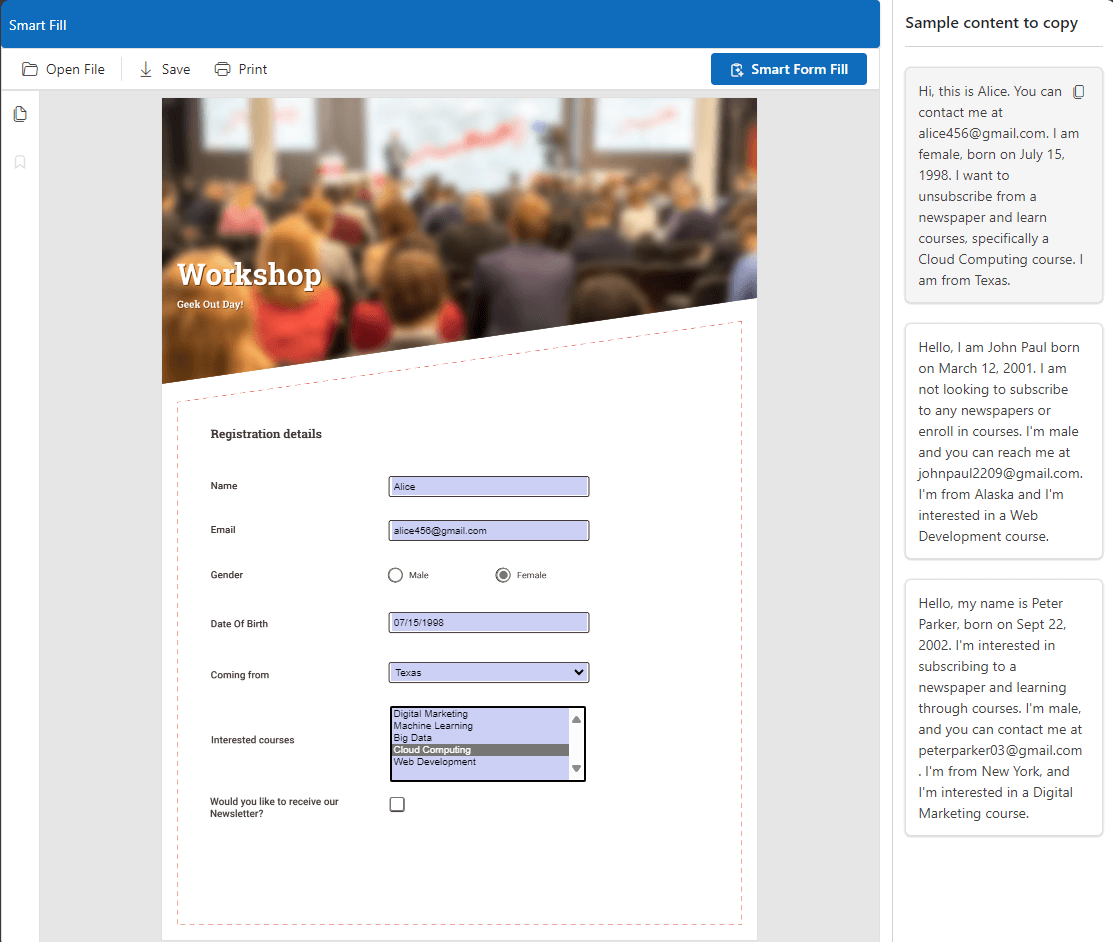
Note: For more details, refer to the smart form filling in Blazor PDF Viewer demos on the web and GitHub.

Syncfusion Blazor components can be transformed into stunning and efficient web apps.
Conclusion
Thanks for reading! Syncfusion Blazor PDF Viewer with AI Assistant exemplifies how technology can enhance productivity by automating manual tasks and transforming our approach to managing valuable information. From summarizing intricate documents to redacting sensitive information and automating form entries, these intelligent features offer a streamlined and seamless user experience.
Embrace the future of document management with AI-powered Blazor PDF Viewer, where innovation and simplicity come together to create smarter workflows.
Our existing customers can download the latest version from the license and downloads page. If you are not a Syncfusion customer, try our 30-day free trial to check out our newest features.
You can also contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!