The numeric text box control for Windows Forms accepts numeric values and restricts all other types of input values. It has built-in support for number types like numeric, percent, and currency. This blog post will provide an overview of the numeric text box control and its important features, such as configuring number ranges, cultures, custom units, and more.
Purchase form with numeric text box
Why do you need the numeric text box?
- Append custom units.
- Hide trailing zeros.
- Modify the appearance.
Getting Started
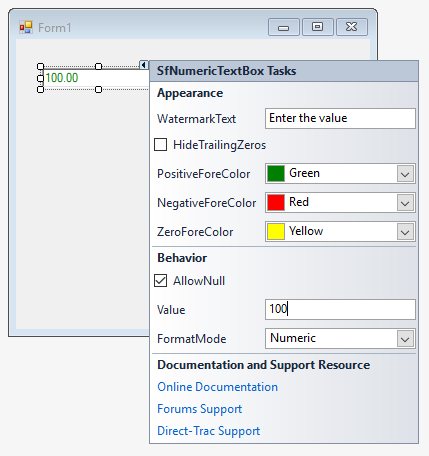
Note: The required assemblies Syncfusion.SfInput.WinForms and Syncfusion.Core.WinForms are added automatically to the application.
- Syncfusion.SfInput.WinForms
- Syncfusion.Core.WinForms
public Form1() { InitializeComponent(); //Initializing new instance of the SfNumericTextBox. SfNumericTextBox sfNumericTextBox = new SfNumericTextBox(); //Initializing the location of the SfNumericTextBox. sfNumericTextBox.Location = new System.Drawing.Point(348, 71); //Initializing the size of the SfNumericTextBox. sfNumericTextBox.Size = new System.Drawing.Size(94, 30); //Initializing the value of the SfNumericTextBox. sfNumericTextBox.Value = 1.23; //Initializing the format mode of the SfNumericTextBox. sfNumericTextBox.FormatMode = FormatMode.Currency; }
Number range support
//Initializing new instance of the SfNumericTextBox. SfNumericTextBox ageTextBox = new SfNumericTextBox(); //Define the minimum value for age text box. ageTextBox.MinValue = 18; //Define the maximum value for age text box. ageTextBox.MaxValue = 60;
Form receiving a value within the provided range
Culture format
//Initializing new instance of the SfNumericTextBox. SfNumericTextBox currencyTextBox = new SfNumericTextBox(); //Assigning the format mode to currency. currencyTextBox.FormatMode = FormatMode.Currency; //Assigning NumberFormatInfo property to German culture format //for displaying currency. currencyTextBox.NumberFormatInfo = new System.Globalization.CultureInfo("de-DE").NumberFormat;
Currency converter with currency symbols
Custom units
//Initializing new instance of the SfNumericTextBox. SfNumericTextBox yearTextBox = new SfNumericTextBox(); //Initializing the value of the SfNumericTextBox. yearTextBox.Value = 7.5; //Initializing the format mode of the SfNumericTextBox. yearTextBox.Suffix = "years";
Values with custom units
Hiding trailing zeros
//Initializing new instance of the SfNumericTextBox. SfNumericTextBox heightTextBox = new SfNumericTextBox(); //Enable the HideTrailingZeros to avoid trailing zeros. heightTextBox.HideTrailingZeros = true; //Initializing the value of the SfNumericTextBox. heightTextBox.Value = 0.20;
Resize form with hidden trailing zeros
UI appearance
The following style properties provide APIs to change the appearance of the numeric text box control.
- BackColor
- BorderColor
- FocusBorderColor
- HoverBorderColor
In the numeric text box control, a number can be differentiated as a positive value, a negative value, and a zero value using the fore color property. There is also an option to modify the watermark fore color.
To modify the fore colors, the following APIs can be used:
- NegativeForeColor
- PositiveForeColor
- WatermarkForeColor
- ZeroForeColor
//Initializing new instance of the SfNumericTextBox. SfNumericTextBox stockTextBox = new SfNumericTextBox(); //Initializing color value for Style property. stockTextBox.Style.BackColor = Color.PaleGreen; stockTextBox.Style.BorderColor = Color.Green; stockTextBox.Style.FocusBorderColor = Color.Blue; stockTextBox.Style.HoverBorderColor = Color.Black; stockTextBox.Style.NegativeForeColor = Color.Red; stockTextBox.Style.PositiveForeColor = Color.Green; stockTextBox.Style.WatermarkForeColor = Color.Gray; //Initializing the value of the SfNumericTextBox. stockTextBox.Value = 2.03; //Initializing the format mode of the SfNumericTextBox. stockTextBox.FormatMode = FormatMode.Percent;
Stock exchange form with different styles based on values
Comments (1)
Hi, How can I change the Step value which is getting changed on Up and down arrow? I tried handling KeyDown, KeyUp etc but after updating the value, it comes to old value again.
Comments are closed.