TL;DR: Learn to secure Word documents using the .NET Word Library (DocIO). This guide offers practical code examples for encrypting, decrypting, and managing editing permissions, ensuring robust document security without Microsoft Office dependencies.
Protecting sensitive information in Word documents is essential for securing legal contracts, financial reports, or private communication. Ensuring access and modifications are restricted to authorized users is critical in many real-world scenarios.
The Syncfusion .NET Word Library (DocIO) is a robust, high-performance library designed to add advanced Word document processing capabilities to .NET apps. It enables programmatic creation, editing, and manipulation of Word documents without Microsoft Office or interop dependencies.
In this blog, we’ll see how to use the .NET Word Library to protect Word documents. From encrypting them with passwords to restricting editing permissions, we’ll help you enhance the document security in your apps.
Agenda:
- Encrypt Word document with password
- Decrypt password-protected Word document
- Restrict editing by:
- Allowing only form fields
- Allowing only comments
- Allowing only reading
- Allowing only revisions
Note: Before proceeding, refer to the .NET Word Library getting started documentation.

Mastering Word documents is a breeze with the Syncfusion Word Library, simplifying every aspect of document creation and management!
Getting started with the .NET Word Library
Follow these steps to get started with the .NET Word Library:
Step 1: First, create a new C# .NET Core Console App in Visual Studio.
Step 2: Then, install the Syncfusion.DocIO.Net.Core NuGet package as a reference from NuGet Gallery.
Step 3: Now, include the following namespaces in the Program.cs file.
using Syncfusion.DocIO;
using Syncfusion.DocIO.DLS;
The project is ready! Let’s dive into the implementation of document protection.
Encrypt Word document with password
For sharing confidential Word documents like legal contracts or financial reports, encrypting the Word document with a password ensures that only authorized users can access it. This is crucial when handling sensitive information. The EncryptDocument method in the .NET Word Library allows us to programmatically add password protection to a Word document.
Refer to the following code example to encrypt a Word document with a password using C#.
// Open the file as a stream.
using (FileStream fileStream = new FileStream(Path.GetFullPath("Template.docx"), FileMode.Open, FileAccess.ReadWrite))
{
// Load an existing Word document.
using (WordDocument document = new WordDocument(fileStream, FormatType.Docx))
{
// Encrypt the Word document with a password.
document.EncryptDocument("password");
// Save the Word document.
using (FileStream outputStream = new FileStream(Path.GetFullPath("Result.docx"), FileMode.Create, FileAccess.ReadWrite))
{
document.Save(outputStream, FormatType.Docx);
}
}
}
Refer to the following image.
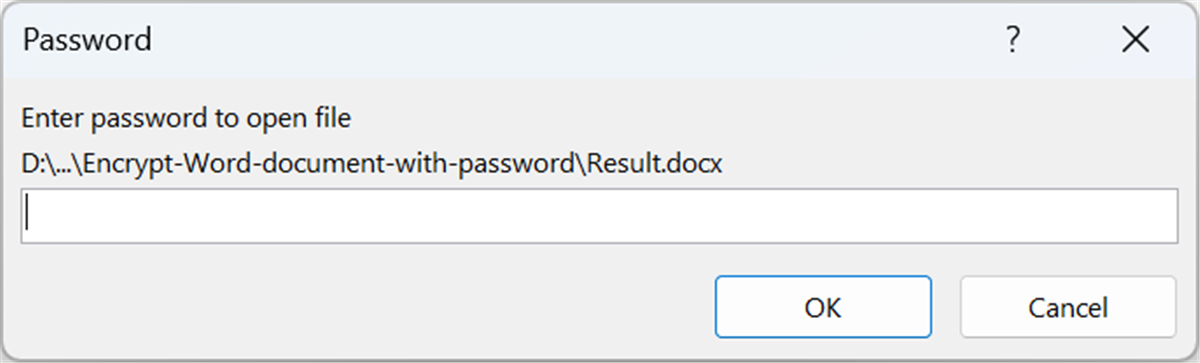
Decrypt password-protected Word document
When receiving a password-protected Word document, automating the decryption process is necessary to access and process the Word document without manually entering the password each time. This is common in document management systems.
With DocIO, you can decrypt a Word document by providing the correct password and then removing encryption using the RemoveEncryption method.
Refer to the following code example.
// Open the file as a stream.
using (FileStream fileStream = new FileStream(Path.GetFullPath("Template.docx"), FileMode.Open, FileAccess.ReadWrite))
{
// Open the encrypted Word document.
using (WordDocument document = new WordDocument(fileStream, FormatType.Docx, "password"))
{
// Remove encryption from the Word document.
document.RemoveEncryption();
// Save the Word document.
using (FileStream outputStream = new FileStream(Path.GetFullPath("Result.docx"), FileMode.Create, FileAccess.ReadWrite))
{
document.Save(outputStream, FormatType.Docx);
}
}
}
Refer to the following image.


Get insights into the Syncfusion’s Word Library and its stunning array of features with its extensive documentation.
Restrict editing
Restricting editing in a Word document helps control the changes or modifications that others can make. It ensures users can only perform specific actions, such as reading, filling out forms, or commenting. Using the DocIO, you can apply these restrictions programmatically.
Allow only form fields
When creating editable forms (e.g., job application forms or survey templates), restricting editing to only form fields ensures users can fill in specific sections without altering the rest of the document.
Refer to the following code example to restrict editing by allowing only form fields in a Word document using C#.
//Open the file as Stream.
using (FileStream fileStream = new FileStream(Path.GetFullPath("Template.docx"), FileMode.Open, FileAccess.ReadWrite))
{
//Load an existing Word document.
using (WordDocument document = new WordDocument(fileStream, FormatType.Docx))
{
// Set document protection with a password, allowing only form fields to be modified.
document.Protect(ProtectionType.AllowOnlyFormFields, "password");
// Save the Word document.
using (FileStream outputStream = new FileStream(Path.GetFullPath("Result.docx"), FileMode.Create, FileAccess.ReadWrite))
{
document.Save(outputStream, FormatType.Docx);
}
}
}
Refer to the following image.
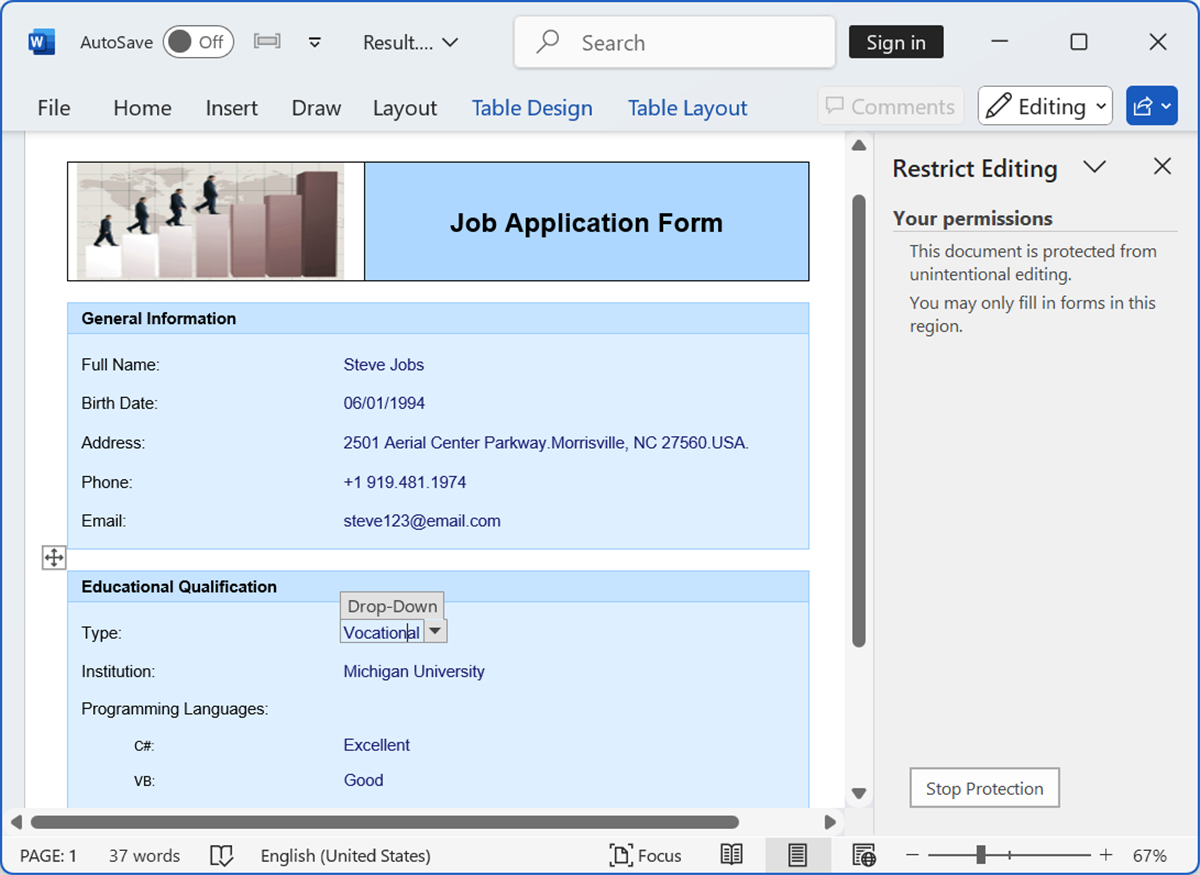
Allow only comments
In team collaborations or document reviews, restricting editing to only comments ensures that the content remains unchanged while allowing feedback to be added, such as in project proposals or academic papers.
Refer to the following code example to restrict editing by allowing only comments in a Word document using C#.
//Open the file as Stream.
using (FileStream fileStream = new FileStream(Path.GetFullPath("Template.docx"), FileMode.Open, FileAccess.ReadWrite))
{
//Load an existing Word document.
using (WordDocument document = new WordDocument(fileStream, FormatType.Docx))
{
//Set document protection with a password, allowing only comments to be added.
document.Protect(ProtectionType.AllowOnlyComments, "password");
// Save the Word document.
using (FileStream outputStream = new FileStream(Path.GetFullPath("Result.docx"), FileMode.Create, FileAccess.ReadWrite))
{
document.Save(outputStream, FormatType.Docx);
}
}
}
Refer to the following image.
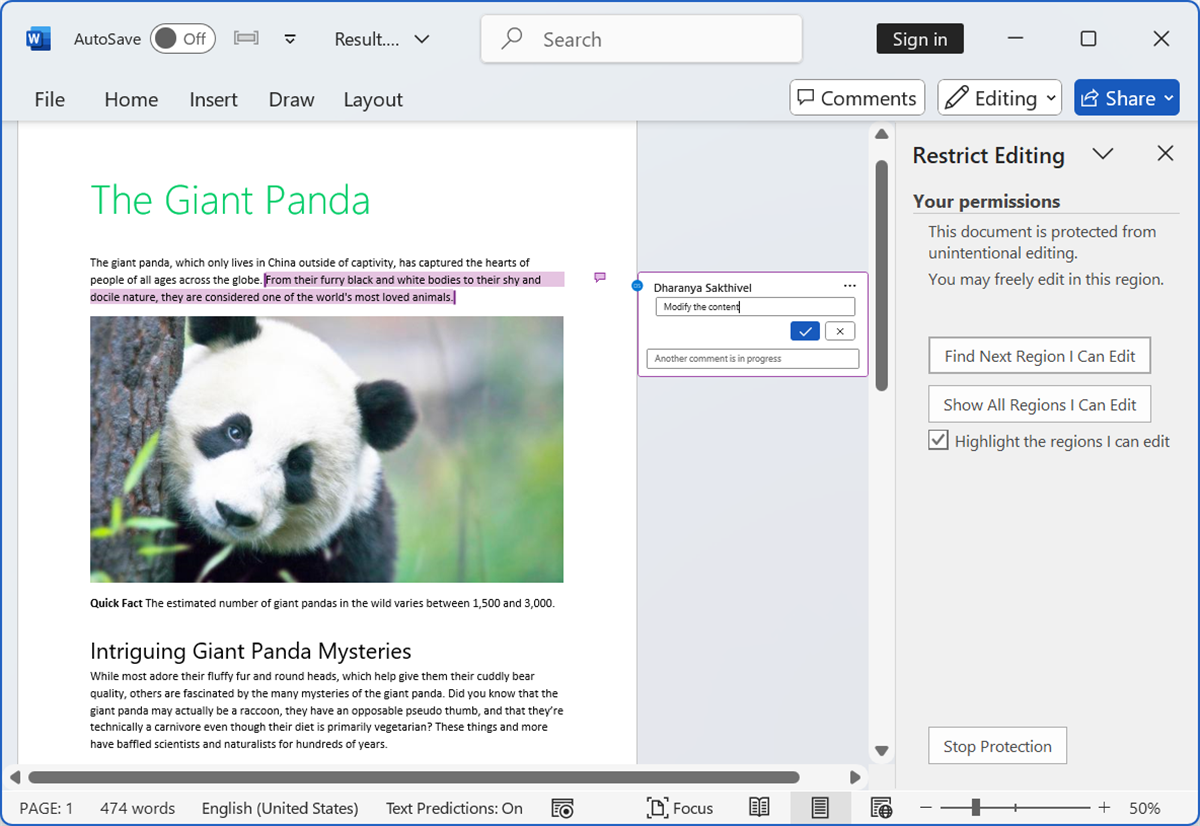

Unearth the endless potential firsthand through demos highlighting the features of the Syncfusion Word Library.
Allow only reading
Restricting editing to read-only ensures that the content cannot be modified, preserving its integrity while allowing users to view it. This is especially useful for sharing Word documents like company policies or public reports.
Refer to the following code example.
//Open the file as Stream.
using (FileStream fileStream = new FileStream(Path.GetFullPath("Template.docx"), FileMode.Open, FileAccess.ReadWrite))
{
//Load an existing Word document.
using (WordDocument document = new WordDocument(fileStream, FormatType.Docx))
{
//Set document protection with a password, allowing only reading (no editing).
document.Protect(ProtectionType.AllowOnlyReading, "password");
// Save the Word document.
using (FileStream outputStream = new FileStream(Path.GetFullPath("Result.docx"), FileMode.Create, FileAccess.ReadWrite))
{
document.Save(outputStream, FormatType.Docx);
}
}
}
Refer to the following image.
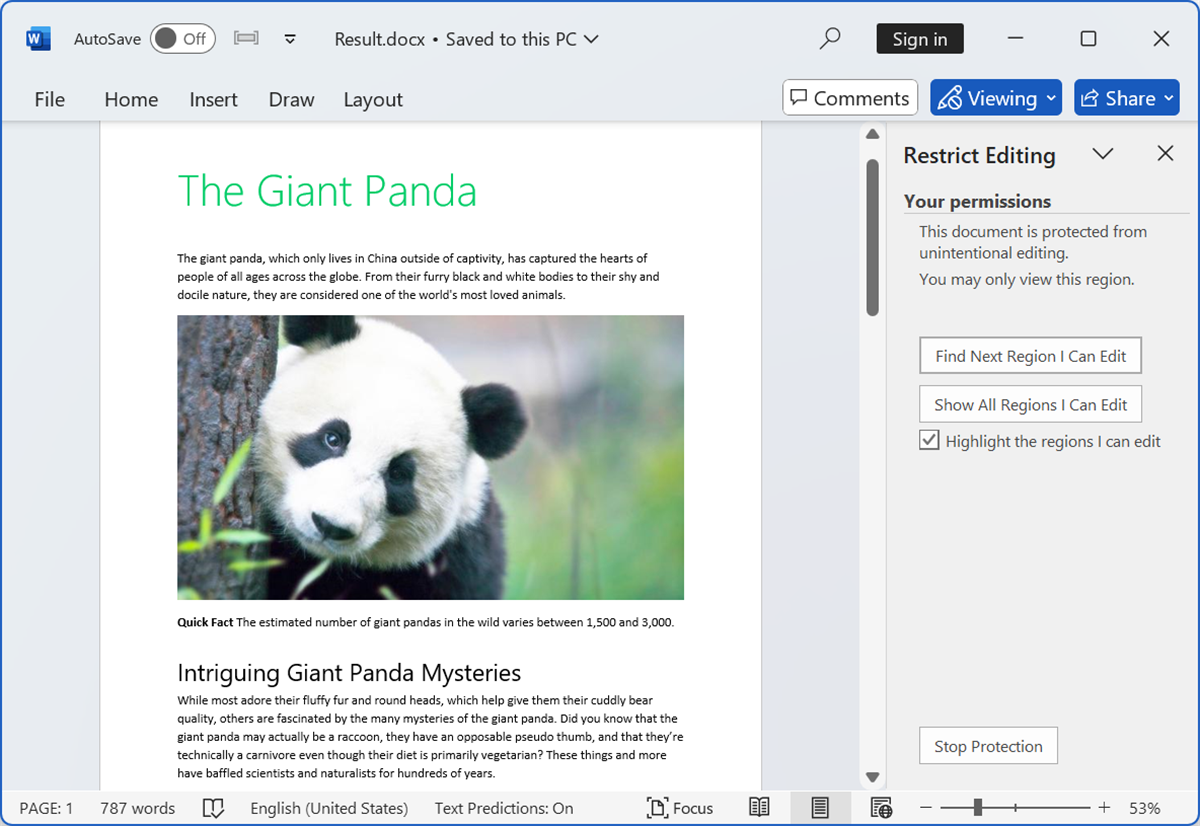
Allow only revisions
In collaborative environments, allowing only revisions enables team members to suggest changes without altering the original document. This is useful for drafting business plans or proposals that require review and approval.
Refer to the following code example to restrict editing by allowing only revisions in a Word document using C#.
//Open the file as Stream.
using (FileStream fileStream = new FileStream(Path.GetFullPath("Template.docx"), FileMode.Open, FileAccess.ReadWrite))
{
//Load an existing Word document.
using (WordDocument document = new WordDocument(fileStream, FormatType.Docx))
{
// Set document protection with a password, allowing only revisions (track changes).
document.Protect(ProtectionType.AllowOnlyRevisions, "password");
// Save the Word document.
using (FileStream outputStream = new FileStream(Path.GetFullPath("Result.docx"), FileMode.Create, FileAccess.ReadWrite))
{
document.Save(outputStream, FormatType.Docx);
}
}
}
Refer to the following image.
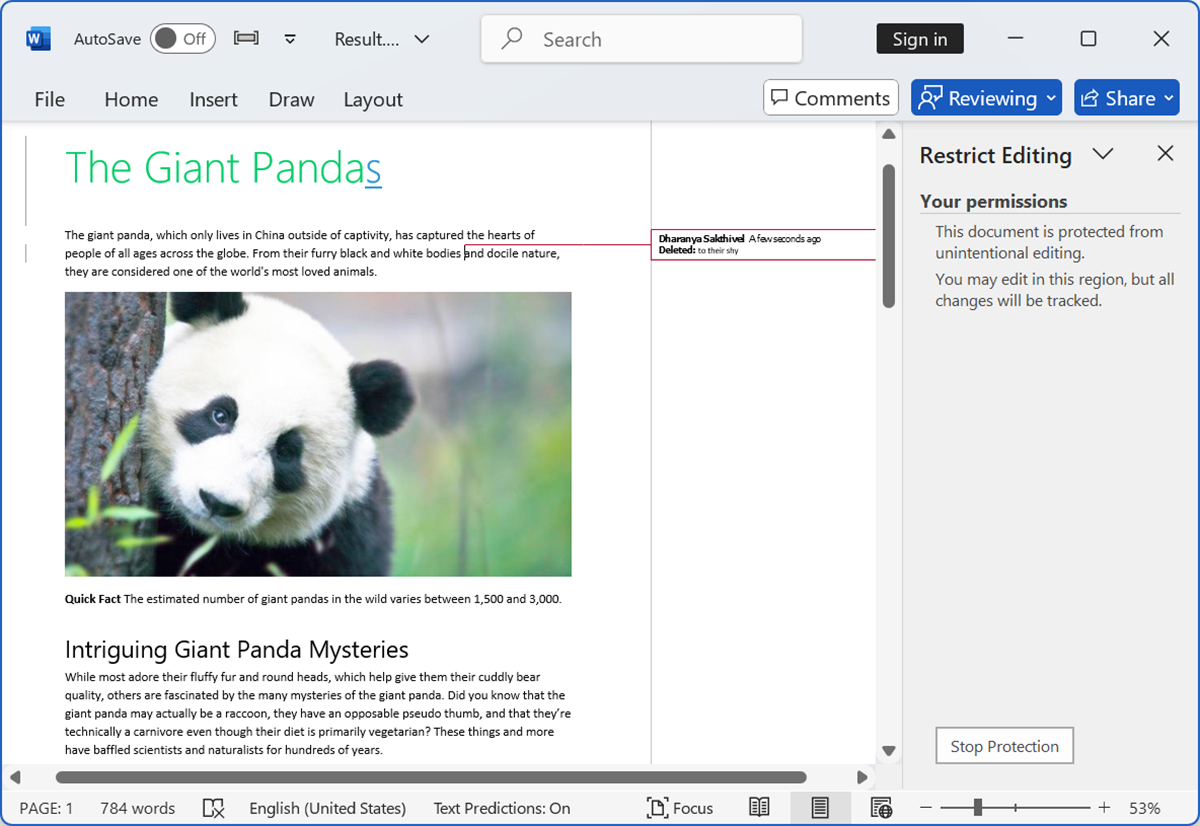
GitHub reference
You can find all the examples of how to protect Word documents using the .NET Word Library in the GitHub repository.

The Syncfusion Word Library provides cross-platform compatibility and can be tailored to your unique needs.
Conclusion
Thanks for reading! In this blog, we’ve seen how to protect Word documents and restrict editing permissions using the Syncfusion .NET Word Library (DocIO). Please take a moment to peruse its documentation, where you’ll find other options and features, all with accompanying code examples.
Apart from this protecting Word documents functionality, our .NET Word Library has the following significant functionalities:
- Create, read, and edit Word documents programmatically.
- Create complex reports by merging data from various data sources into a Word template through mail merge.
- Merge, split, and organize Word documents.
- Convert Word documents into HTML, RTF, PDF, images, and other formats.
You can find more examples of Word Library on this GitHub location.
Are you already a Syncfusion user? You can download the product setup here. If you’re not yet a Syncfusion user, you can download a 30-day free trial.
If you have questions, contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!