TL;DR: Learn to create globally accessible, culturally relevant Flutter apps. Covering everything from adapting UI elements like dates, currencies, and text direction to implementing multilingual support, this guide offers step-by-step instructions with Syncfusion Flutter widgets and packages. Ideal for developers looking to expand their app’s reach with easy-to-implement, user-friendly localization techniques.
In today’s global market, an app should be able to serve people from different countries and cultures. Globalization and localization simply mean making your app easy to use and understand for anyone.
Localization is the process of adapting your app to meet users’ language, cultural, and regional preferences in different parts of the world. It involves more than just translating text—localization ensures that dates, times, currencies, and even visual elements are appropriate for the target audience. Localizing your app makes it more relevant and user-friendly for people from different regions, which can significantly improve user experience and increase your app’s global reach.
Syncfusion Flutter widgets are written natively in Dart to help you create rich, high-quality apps for iOS, Android, Web, Windows, macOS, and Linux from a single code base.
In Flutter, localization is made easier with built-in support for multiple languages and tools that help you manage and implement translations efficiently. This process allows your app to automatically adjust its content based on the user’s language and region settings, providing a seamless experience for users everywhere.
In this blog, we’ll explore the globalization and localization features supported in the Syncfusion Flutter widgets.
Globalization
Globalization refers to designing and developing your app to serve the users belonging to various languages and regions without requiring significant changes to the core code. This involves considering factors such as:
- Date and time formats: Handling different date, time, and number formats based on the user’s locale.
- Currency formats: Displaying prices and currencies according to regional standards.
- Text direction: Supporting both left-to-right (LTR) and right-to-left (RTL) text directions.
The following table displays the globalization options supported by the Syncfusion Flutter widgets.
Widgets | Links |
SfCartesianChart | |
SfCalendar | |
SfDataGrid | |
SfDateRangePicker | |
SfGauges | |
SfRangeSlider | |
SfRangeSelector |
Localization
Localization is the process of adapting the content of your app to a specific locale, including translating text, adjusting layouts, and even changing icons or images to suit cultural preferences. It involves:
- Translating UI text: Converting all user-facing text to the target language.
- Adjusting layouts: Ensuring the UI works well in different languages, especially those requiring more space or having a different text direction.
- Cultural adaptations: Adapting images, colors, and icons to be culturally appropriate.
By default, Syncfusion Flutter widgets are localized in English (en-US). However, we can easily extend this support to other languages by adding the syncfusion_localizations package in our Flutter app. This package currently supports 76 languages, allowing you to make your app more accessible to users globally.
The following table displays the languages supported by the Syncfusion Flutter widgets.
Supported locales | Languages |
af | Afrikaans |
am | Amharic |
ar | Arabic |
az | Azerbaijani |
be | Belarusian |
bg | Bulgarian |
bn | Bengali Bangla |
bs | Bosnian |
ca | Catalan Valencian |
cs | Czech |
da | Danish |
de | German |
el | Modern Greek |
en | English |
es | Spanish Castilian |
et | Estonian |
eu | Basque |
fa | Persian |
fi | Finnish |
fil | Filipino Pilipino |
fr | French |
gl | Galician |
gu | Gujarati |
he | Hebrew |
hi | Hindi |
hr | Croatian |
hu | Hungarian |
hy | Armenian |
id | Indonesian |
is | Icelandic |
it | Italian |
ja | Japanese |
ka | Georgian |
kk | Kazakh |
km | Khmer Central Khmer |
kn | Kannada |
ko | Korean |
ky | Kirghiz Kyrgyz |
lo | Lao |
lt | Lithuanian |
lv | Latvian |
mk | Macedonian |
ml | Malayalam |
mn | Mongolian |
mr | Marathi |
ms | Malay |
my | Burmese |
nb | Norwegian Bokmål |
ne | Nepali |
nl | Dutch Flemish |
no | Norwegian |
or | Oriya |
pa | Panjabi Punjabi |
pl | Polish |
ps | Pushto Pashto |
pt | Portuguese (+ one country variation) |
ro | Romanian Moldavian Moldovan |
ru | Russian |
si | Sinhala Sinhalese |
sk | Slovak |
sl | Slovenian |
sq | Albanian |
sr | Serbian |
sv | Swedish |
sw | Swahili |
ta | Tamil |
te | Telugu |
th | Thai |
tl | Tagalog |
tr | Turkish |
uk | Ukrainian |
ur | Urdu |
uz | Uzbek |
vi | Vietnamese |
zh | Chinese (+ 2 country variations) |
zu | Zulu |
Let’s see how to localize the text in the Syncfusion Flutter Calendar widget. To achieve localization for Syncfusion widgets, add the syncfusion localizations and syncfusion flutter calendar packages as dependencies in your pubspec.yaml file. This will enable you to include additional languages and integrate the Syncfusion Calendar widget into your Flutter app.
To begin the Flutter localization process, using the MaterialApp widget in your Flutter apps is essential. Without MaterialApp, localization cannot be achieved. This widget includes several key properties that facilitate localization:
1. Locale
This property sets the initial locale for the app’s Localizations widget. If the locale is not specified, the system’s default locale is used.
2. LocalizationsDelegates
These delegates collectively define all the localized resources for the app’s Localizations widget. You should specify this parameter if your app needs translations for one or more locales listed in GlobalMaterialLocalizations. The delegates will handle the retrieval of localized resources.
To add localization for Syncfusion Flutter widgets, you must include the SfGlobalLocalizations.delegate parameter in the localizationsDelegates list. This delegate ensures that the Syncfusion widgets within your Flutter app are localized according to the selected language and locale.
3. supportedLocales
This is a list of locales that your app supports. By default, only American English (en-US) is supported. You should configure this list according to the locales your app will support. This list is crucial and cannot be null, with its default value being [const Locale (‘en’, ‘US’)].
The order of the locales in the supportedLocales list is important. The default locale resolution algorithm, basicLocaleListResolution, prioritizes matching in the following order:
- Locale.languageCode, Locale.scriptCode, and Locale.countryCode
- Locale.languageCode and Locale.scriptCode only
- Locale.languageCode and Locale.countryCode only
- Locale.languageCode only
- Locale.countryCode only when all preferred locales fail to match
- Returns the first element of supported locales as a fallback
Refer to the following code example to add multiple localization for the Flutter Calendar widget.
Locale _locale = const Locale('en', 'US');
final CalendarController _calendarController = CalendarController();
@override
void initState() {
_calendarController.view = CalendarView.month;
super.initState();
}
@override
Widget build(BuildContext context) {
return MaterialApp(
locale: _locale,
localizationsDelegates: const [
GlobalMaterialLocalizations.delegate,
GlobalWidgetsLocalizations.delegate,
GlobalCupertinoLocalizations.delegate,
SfGlobalLocalizations.delegate,
],
supportedLocales: const [
Locale('en', 'US'),
Locale('es', 'ES'),
Locale('ja', 'JP'),
// Add other locales here.
],
home: Scaffold(
body: SfCalendar(
allowedViews: const <CalendarView>[
CalendarView.day,
CalendarView.week,
CalendarView.workWeek,
CalendarView.month,
CalendarView.timelineDay,
CalendarView.timelineWeek,
CalendarView.timelineWorkWeek,
CalendarView.schedule,
],
showDatePickerButton: true,
controller: _calendarController,
showNavigationArrow: true,
monthViewSettings: const MonthViewSettings(
appointmentDisplayMode: MonthAppointmentDisplayMode.appointment),
timeSlotViewSettings: const TimeSlotViewSettings(
minimumAppointmentDuration: Duration(minutes: 60),
),
),
),
);
}
Refer to the following image.
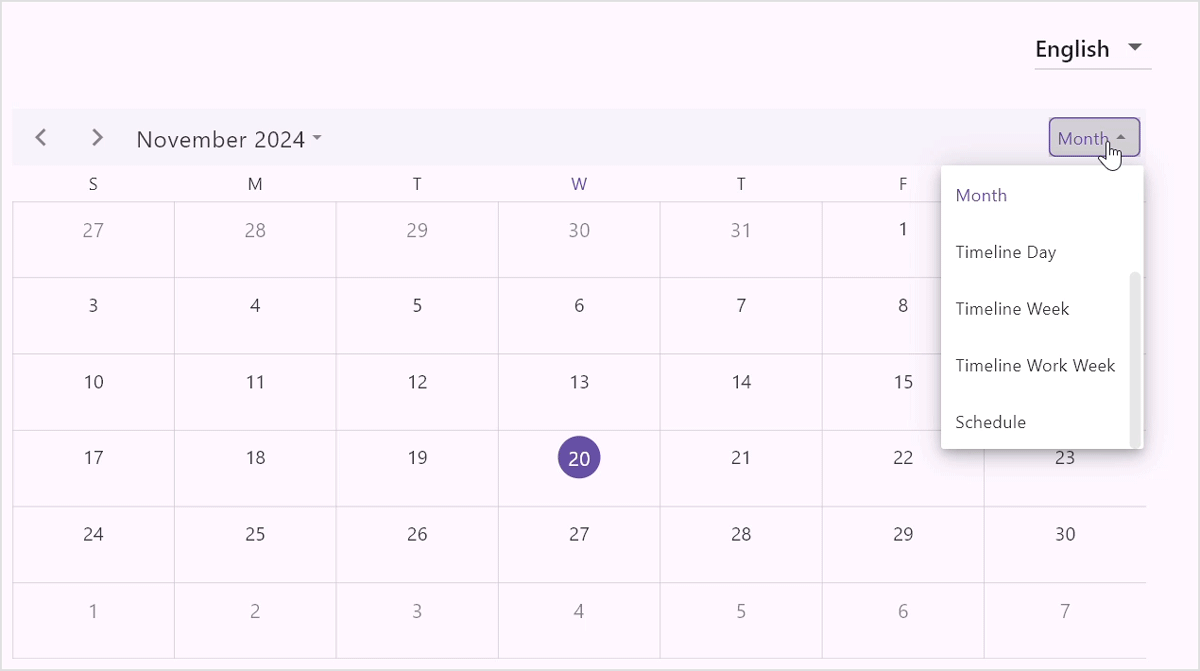
In some cases, simply specifying the language code may not be enough to properly differentiate between variations of the same language. For example, the Chinese language has both simplified and traditional scripts and regional differences in how characters are written. To handle this, you can specify additional parameters like the script and country codes in the supportedLocales list.
Refer to the following code example. Here, languageCode is the primary language code (e.g., zh for Chinese), scriptCode specifies the script type, such as Hant for traditional Chinese or Hans for simplified Chinese, and countryCode specifies the region, like TW for Taiwan or CN for China.
Locale _locale = const Locale.fromSubtags(languageCode: 'zh');
final CalendarController _calendarController = CalendarController();
// Full Chinese support for CN, TW, and HK
final List<Locale> _locales = const <Locale>[
Locale.fromSubtags(languageCode: 'zh'), // Generic Chinese 'zh'
Locale.fromSubtags(
languageCode: 'zh',
scriptCode: 'Hant'), // Generic traditional Chinese 'zh_Hant'
Locale.fromSubtags(
languageCode: 'zh',
scriptCode: 'Hans',
countryCode: 'CN'), // 'zh_Hans_CN'
Locale.fromSubtags(
languageCode: 'zh',
scriptCode: 'Hant',
countryCode: 'HK'), // 'zh_Hant_HK'
];
@override
void initState() {
_calendarController.view = CalendarView.month;
super.initState();
}
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
locale: _locale,
localizationsDelegates: const [
GlobalMaterialLocalizations.delegate,
GlobalWidgetsLocalizations.delegate,
GlobalCupertinoLocalizations.delegate,
SfGlobalLocalizations.delegate,
],
supportedLocales: _locales,
home: Scaffold(
body: SfCalendar(
controller: _calendarController,
monthViewSettings: const MonthViewSettings(
showAgenda: true,
),
),
),
);
}
Refer to the following image.
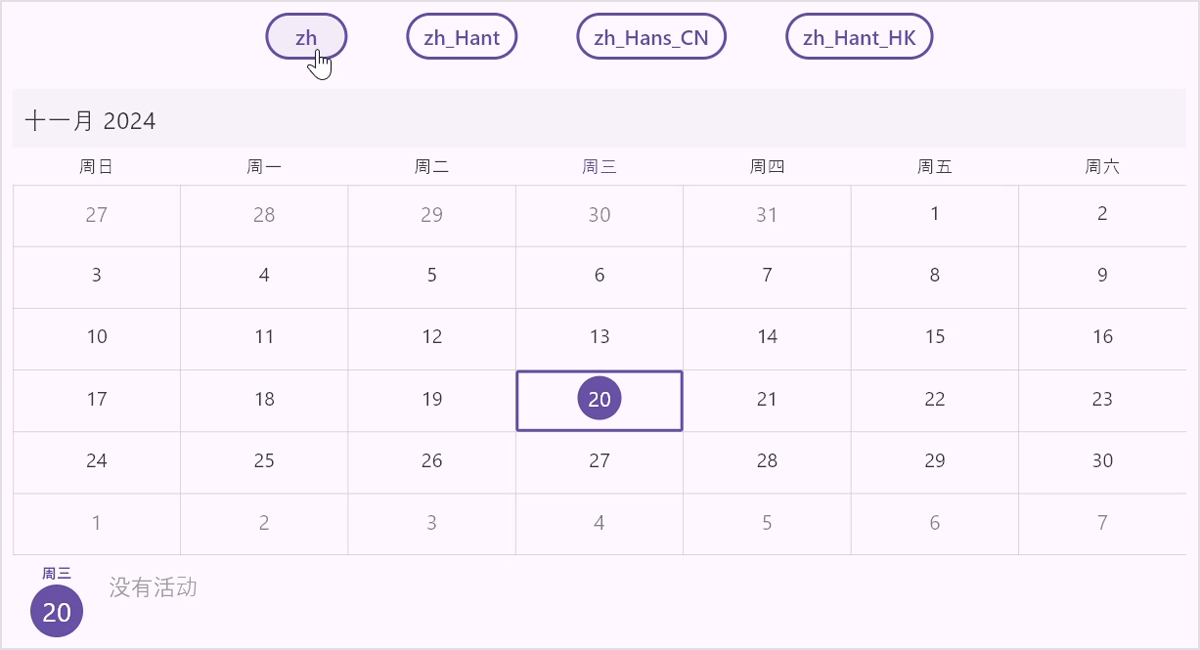
How to create custom localization language in Syncfusion Flutter widgets
While Syncfusion Flutter widgets provide extensive language support, there may be cases where you need to add a custom culture or modify existing localizations. This is where extending the SfLocalizations class becomes useful. Creating a subclass of SfLocalizations allows you to override specific properties to provide custom text for different labels in Syncfusion widgets.
For example, if you want to change the labels in a calendar widget for English (en), you can create a class called SfLocalizationsExt that extends SfLocalizations. In this class, you can override properties like noEventsCalendarLabel, todayLabel, and weeknumberLabel to display custom text. You can adjust the labels to fit your app’s style.
After creating your custom localization class, create a delegate by extending the LocalizationsDelegate<SfLocalizations> class. This delegate will load your custom localization when the app is set to the relevant locale. Finally, we should add this delegate to the localizationsDelegates list in the MaterialApp widget to make sure our custom localization is used when needed.
Here’s an example of how you can implement this for the custom English (en) language:
class _CustomLocalizationDemoState extends State<_CustomLocalizationDemo> {
final Locale _locale = const Locale('en');
final CalendarController _calendarController = CalendarController();
@override
void initState() {
_calendarController.view = CalendarView.month;
super.initState();
}
@override
Widget build(BuildContext context) {
return MaterialApp(
locale: _locale,
localizationsDelegates: [
GlobalMaterialLocalizations.delegate,
// Specify the delegate directly
SfLocalizationsEnDelegate.delegate,
],
supportedLocales: [
_locale,
],
home: Scaffold(
body: SfCalendar (
….
),
),
);
}
}
/// The translations for English (`en`).
class SfLocalizationsExt extends SfLocalizations {
SfLocalizationsExt();
@override
String get noEventsCalendarLabel => '𝓝𝓞 𝓔𝓥𝓔𝓝𝓣𝓢';
@override
String get noSelectedDateCalendarLabel => '𝓝𝓞 𝓢𝓔𝓛𝓔𝓒𝓣𝓔𝓓 𝓓𝓐𝓣𝓔';
@override
String get todayLabel => '𝓣𝓞𝓓𝓐𝓨';
@override
String get weeknumberLabel => '𝓦𝓔𝓔𝓚 𝓝𝓤𝓜𝓑𝓔𝓡';
@override
String get allDayLabel => '𝓐𝓛𝓛 𝓓𝓐𝓨';
@override
String get allowedViewDayLabel => '𝓓𝓐𝓨';
@override
String get allowedViewMonthLabel => '𝓜𝓞𝓝𝓣𝓗';
@override
String get allowedViewScheduleLabel => '𝓢𝓒𝓗𝓔𝓓𝓤𝓛𝓔';
@override
String get allowedViewTimelineDayLabel => '𝓣𝓘𝓜𝓔𝓛𝓘𝓝𝓔 𝓓𝓐𝓨';
@override
String get allowedViewTimelineMonthLabel => '𝓣𝓘𝓜𝓔𝓛𝓘𝓝𝓔 𝓜𝓞𝓝𝓣𝓗';
@override
String get allowedViewTimelineWeekLabel => '𝓣𝓘𝓜𝓔𝓛𝓘𝓝𝓔 𝓦𝓔𝓔𝓚';
@override
String get allowedViewTimelineWorkWeekLabel => '𝓣𝓘𝓜𝓔𝓛𝓘𝓝𝓔 𝓦𝓞𝓡𝓚 𝓦𝓔𝓔𝓚';
@override
String get allowedViewWeekLabel => '𝓦𝓔𝓔𝓚';
@override
String get allowedViewWorkWeekLabel => '𝓦𝓞𝓡𝓚 𝓦𝓔𝓔𝓚';
// other properties
}
class SfLocalizationsEnDelegate extends LocalizationsDelegate<SfLocalizations> {
static LocalizationsDelegate<SfLocalizations> delegate = SfLocalizationsEnDelegate();
@override
bool isSupported(Locale locale) => locale.languageCode == 'en';
@override
Future<SfLocalizations> load(Locale locale) {
return SynchronousFuture<SfLocalizations<(SfLocalizationsExt());
}
@override
bool shouldReload(LocalizationsDelegate<SfLocalizations> old) => false;
}
Refer to the following image.
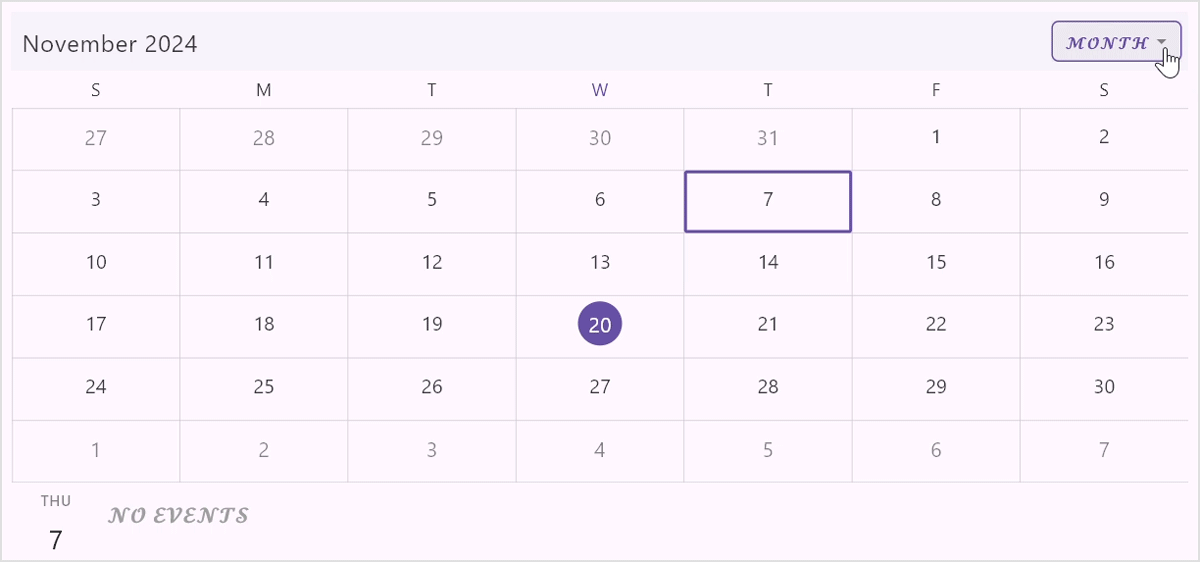
We have reached the end of this blog. The following table lists the Syncfusion Flutter widgets that support localization. This table will help you quickly identify which widgets can be localized, allowing you to create a more accessible and user-friendly app for a global audience.
Widgets | In-built localization |
Barcode | This widget does not use predefined English words, so it does not support localization. |
Yes | |
Yes | |
Yes | |
Yes | |
Gauges | This widget does not use predefined English words, so it does not support localization. |
Maps | This widget does not use predefined English words, so it does not support localization. |
Slider | This widget does not use predefined English words, so it does not support localization. |
TreeMap | This widget does not use predefined English words, so it does not support localization. |
This widget does not use predefined English words, so it does not support localization. | |
Yes | |
SparkCharts | This widget does not use predefined English words, so it does not support localization. |
Signature Pad | This widget does not use predefined English words, so it does not support localization. |
Xlsio | This widget does not use predefined English words, so it does not support localization. |
GitHub reference
For more details, refer to the Globalization and localization support in the Flutter widgets GitHub demo.

Unlock the power of Syncfusion’s highly customizable Flutter widgets.
Conclusion
Thanks for reading! In this blog, we’ve explored the globalization and localization features supported in the Syncfusion Flutter widgets. By implementing these features, you can provide your global audience with a more personalized and engaging experience. Give it a try, and feel free to share your feedback in the comment section below.
If you’re an existing customer, you can download the latest version of Essential Studio® from the License and Downloads page. For those new to Syncfusion, try our 30-day free trial to explore all our features.
If you have questions, contact us through our support forum, support portal, or feedback portal. As always, we are happy to assist you!