TL;DR: Easily convert PowerPoint presentations to PDF while preserving layout, formatting, and accessibility. The Syncfusion .NET PowerPoint Library allows seamless conversion with just a few lines of C# code—no Microsoft PowerPoint required. Explore advanced options like tagged PDFs for accessibility, font substitution, optimized images, and PDF/A compliance.
PowerPoint presentations often need to be shared across different platforms while maintaining their original layout and formatting. Converting them to PDF ensures consistency, accessibility, and easy distribution.
The Syncfusion .NET PowerPoint Library (Presentation) simplifies this process, offering seamless PowerPoint-to-PDF conversion with just a few lines of C# code. This standalone library operates independently of Microsoft PowerPoint, eliminating interop dependencies and providing a lightweight, efficient solution.
This PowerPoint-to-PDF conversion is available in multiple environments, such as:
- Windows
- Linux
- macOS
- Mobile devices
- Docker
- Cloud computing environments
Whether you’re customizing handouts, including hidden slides, or ensuring PDF conformance for accessibility, our .NET PowerPoint Library provides the flexibility needed for every scenario. This blog explores advanced options for PowerPoint-to-PDF conversion, including optimizing identical images, handling fonts without installing them on production machines, and configuring fallback fonts for Unicode text.
Let’s look at the various options available, along with code examples.
Note: If you are new to our .NET PowerPoint library, it is highly recommended to follow our getting started guide.
Advanced options:
- Accessible PDF document (PDF/UA)
- PDF conformance level (PDF/A)
- Handouts
- Include notes pages
- Include hidden slides
- Optimize identical images
Fallback fonts:
- Initialize default fallback fonts
- Fallback fonts based on script type
- Fallback fonts for a range of Unicode text
Font substitution:
Let’s get started with the implementation!

Say goodbye to tedious PDF tasks and hello to effortless document processing with Syncfusion's PDF Library.
Getting started
Step 1: First, create a new C# .NET Core Console App in Visual Studio.
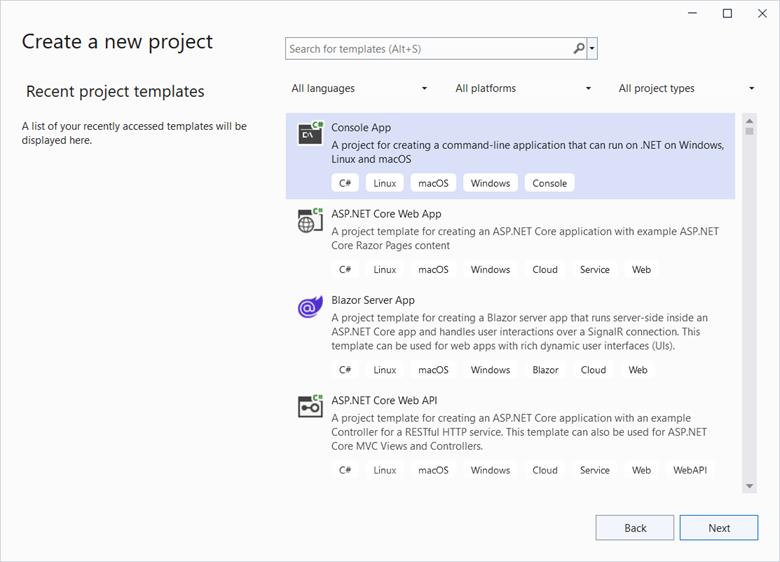
Step 2: Then, install the Syncfusion.PresentationRenderer.Net.Core NuGet package as a reference to the app from NuGet Gallery.
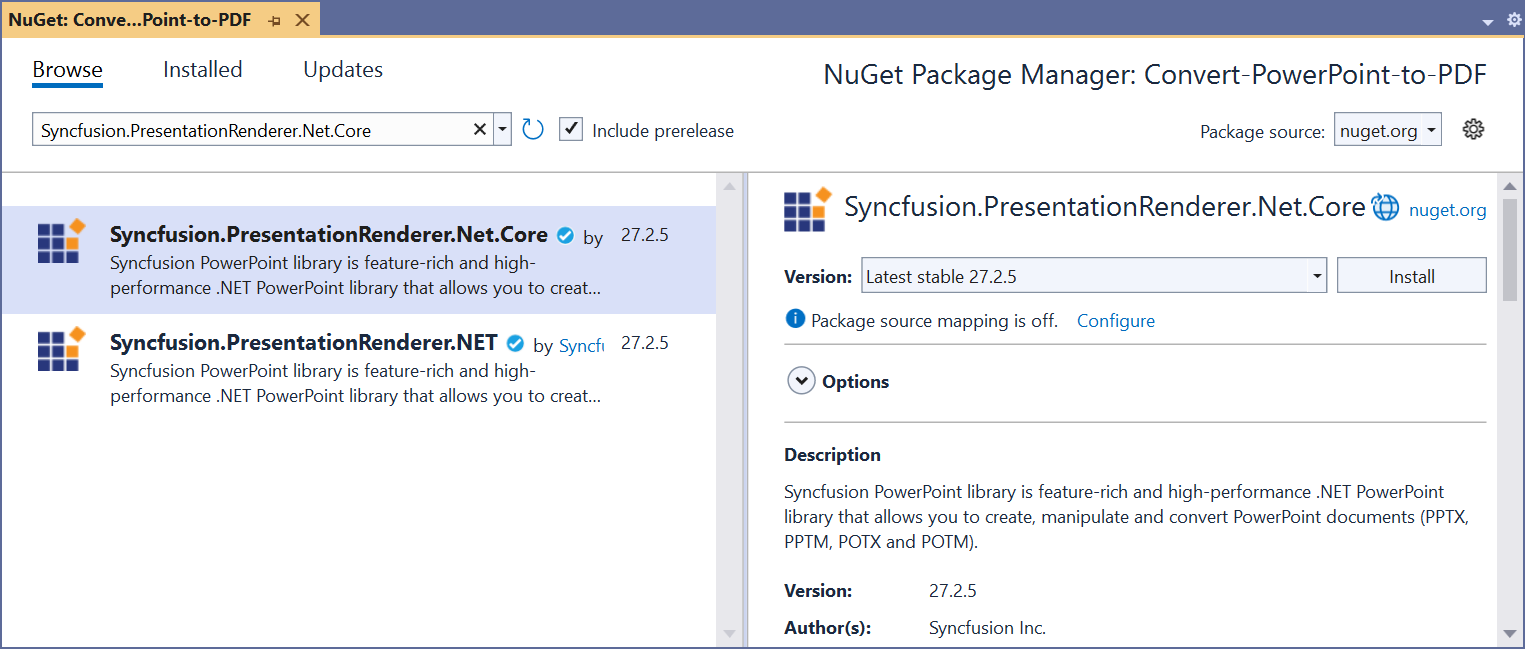
Step 3: Now, include the following namespaces in the Program.cs file.
using Syncfusion.Presentation; using Syncfusion.PresentationRenderer; using Syncfusion.Pdf;
The project is ready! Let’s write the code to convert a PowerPoint presentation into a PDF.
//Open the PowerPoint presentation file stream. using (FileStream inputStream = new FileStream("Template.pptx", FileMode.Open, FileAccess.ReadWrite)) { //Load an existing PowerPoint Presentation. using (IPresentation pptxDoc = Presentation.Open(inputStream)) { //Convert PowerPoint into PDF document. using (PdfDocument pdfDocument = PresentationToPdfConverter.Convert(pptxDoc)) { //Save the PDF file to the file system. using (FileStream outputStream = new FileStream("Output.pdf", FileMode.Create, FileAccess.ReadWrite)) { pdfDocument.Save(outputStream); } } } }
By executing this code example, we will get the output like in the following image.
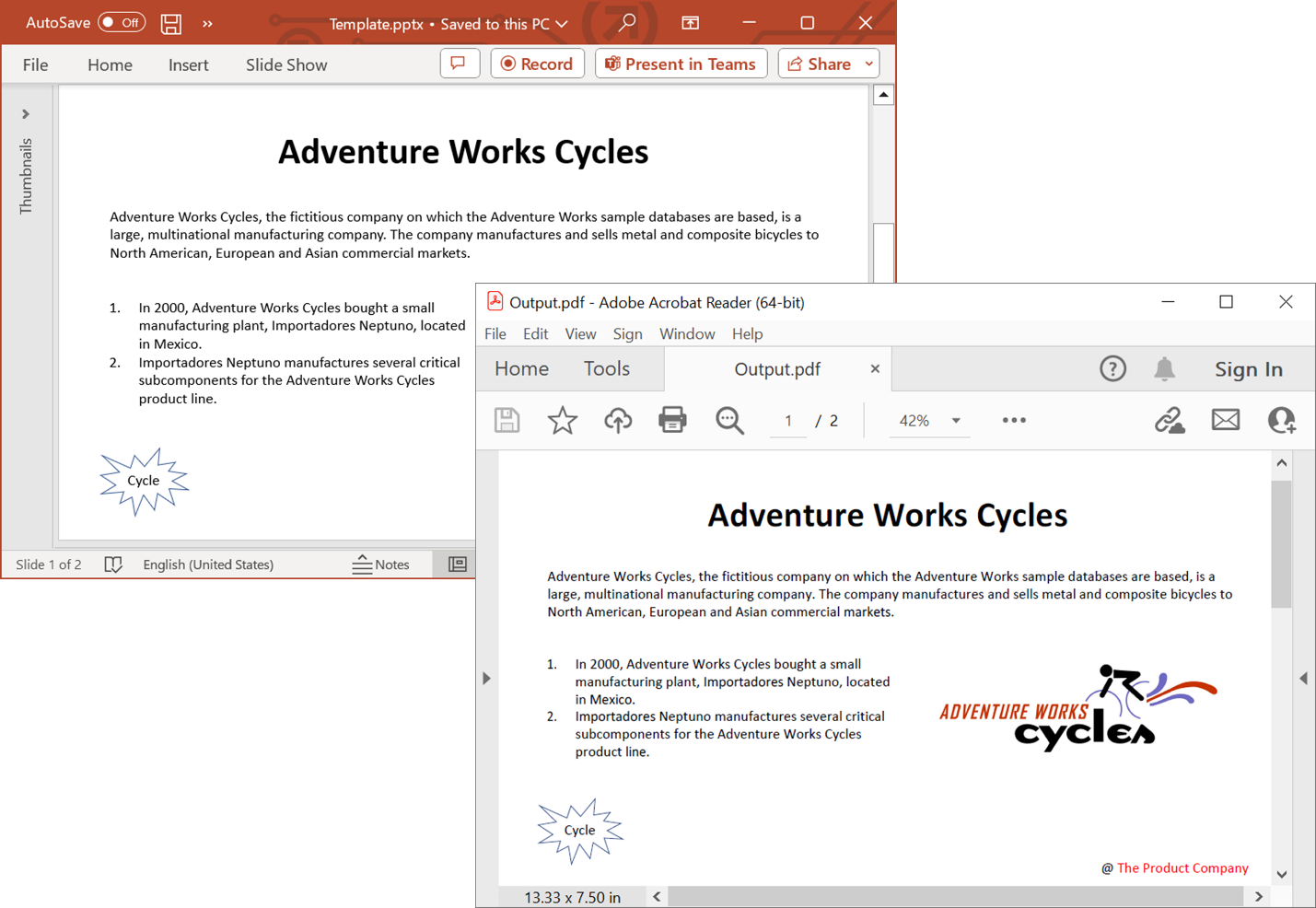
Now, let’s explore the advanced options!
Advanced options
Accessible PDF document (PDF/UA)
Generate tagged PDFs from your presentations to enhance screen reader compatibility and ensure accessibility for individuals with disabilities. This feature can help universities meet accessibility standards for visually impaired students.
To create an accessible PDF with structured tags, simply enable the AutoTag setting during conversion.
PresentationToPdfConverterSettings pdfConverterSettings = new PresentationToPdfConverterSettings(); //Enable this flag to generate an accessible PDF with structured tags. pdfConverterSettings.AutoTag = true; PdfDocument pdfDocument = PresentationToPdfConverter.Convert(pptxDoc, pdfConverterSettings);
Refer to the following image.
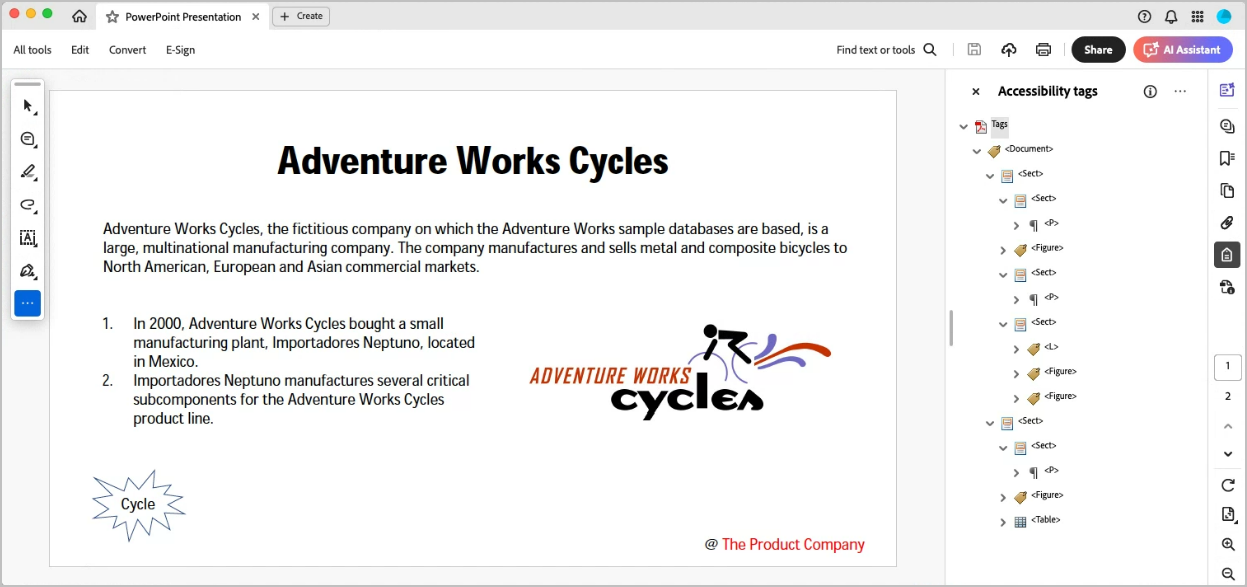
PDF conformance level (PDF/A)
When submitting documents to regulatory bodies for legal, compliance, or archival purposes, ensuring they adhere to industry standards like PDF/A is crucial. For example, financial institutions can use this feature to produce regulatory-compliant reports for long-term storage.
To achieve this, set the desired conformance level during the conversion process as shown in the following code example.
PresentationToPdfConverterSettings pdfConverterSettings = new PresentationToPdfConverterSettings(); //Set the conformance for PDF/A3A conversion. pdfConverterSettings.PdfConformanceLevel = PdfConformanceLevel.Pdf_A3A; PdfDocument pdfDocument = PresentationToPdfConverter.Convert(pptxDoc,
pdfConverterSettings);
Refer to the following image.
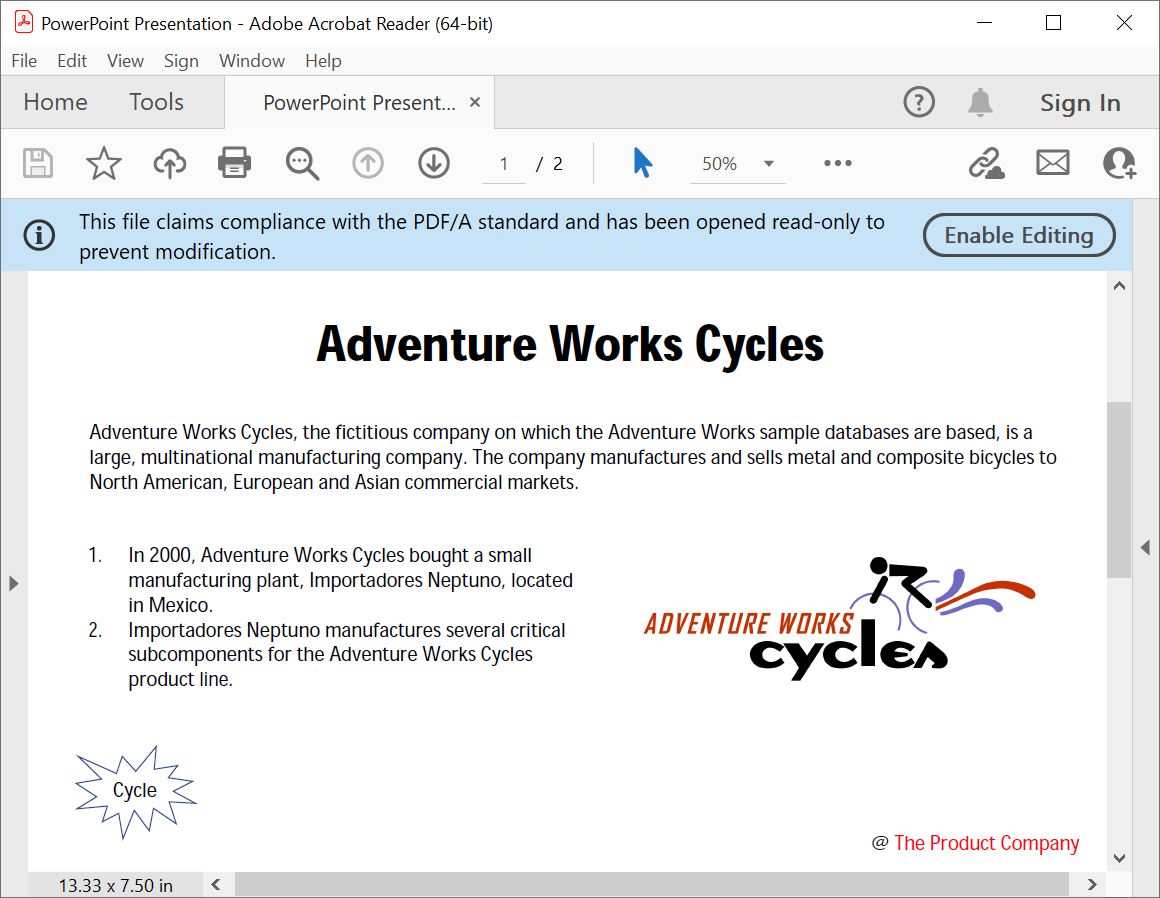
Handouts
For corporate training sessions, you might need to print multiple slides on a single page to reduce paper usage and provide a compact handout summarizing key points for participants. The handouts option enables converting slides into a PDF with multiple slides per page, making it ideal for printing and easy distribution while optimizing space and cost.
Let’s enable the handouts feature and specify the number of slides per page, as shown in the following code example.
PresentationToPdfConverterSettings pdfConverterSettings = new PresentationToPdfConverterSettings(); //Enable the handouts and number of pages per slide options in converter settings. pdfConverterSettings.PublishOptions = PublishOptions.Handouts; pdfConverterSettings.SlidesPerPage = SlidesPerPage.Nine; PdfDocument pdfDocument = PresentationToPdfConverter.Convert(pptxDoc, pdfConverterSettings);
Refer to the following image.
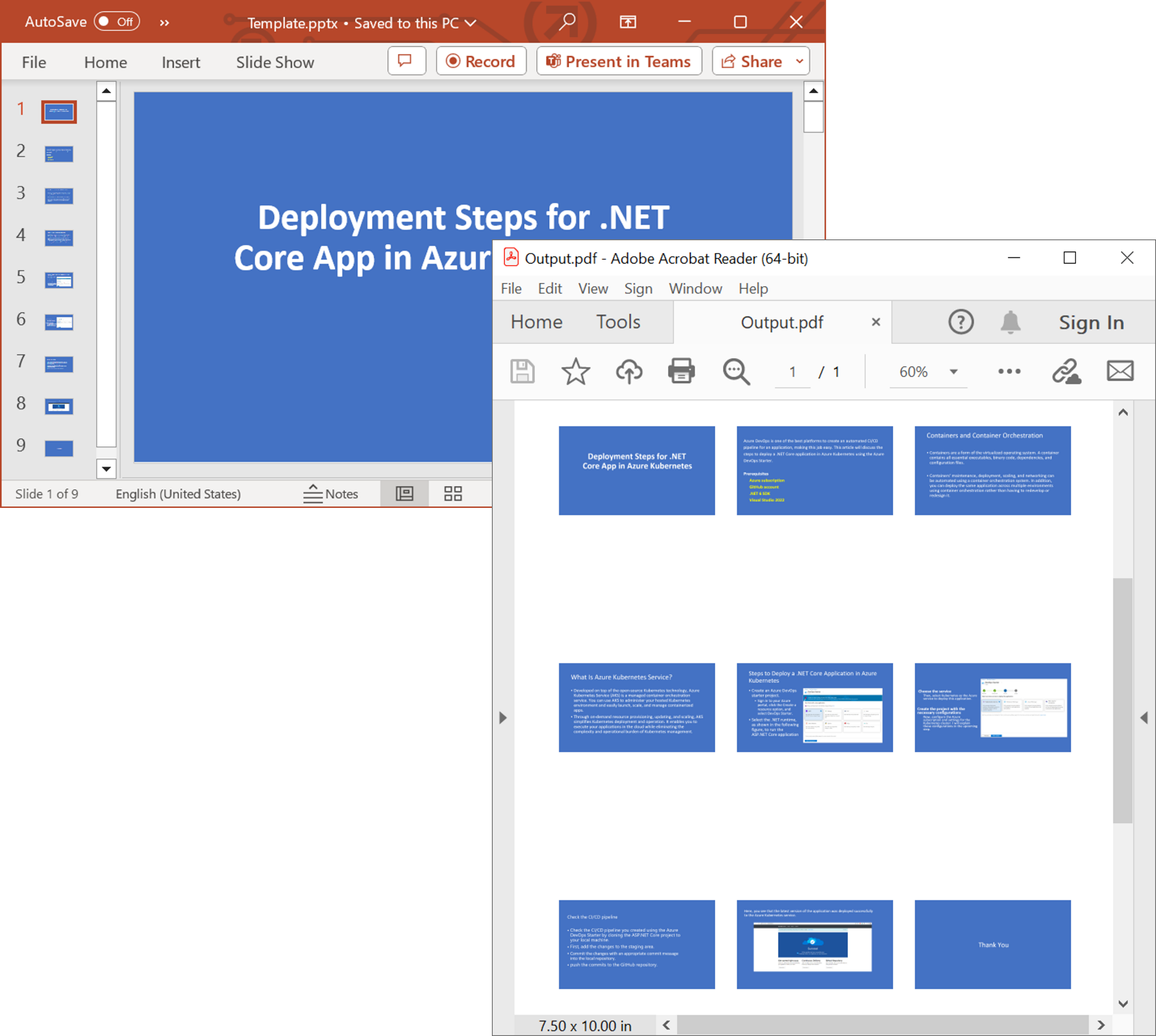
Include notes pages
In educational presentations, including speaker notes alongside slides in the PDF helps students follow along with explanations and key insights during a lecture or review. This option converts slides along with their associated notes, providing context and additional explanations, making the PDF more informative and suitable for distribution to audiences who need extra detail.
Refer to the following code example.
PresentationToPdfConverterSettings pdfConverterSettings = new PresentationToPdfConverterSettings(); //Enables the option to include notes page content in the PDF conversion. pdfConverterSettings.PublishOptions = PublishOptions.NotesPages; PdfDocument pdfDocument = PresentationToPdfConverter.Convert(pptxDoc, pdfConverterSettings);
Refer to the following image.

Include hidden slides
For a customized client presentation, we might need to hide some slides during live meetings but need to include them in a post-meeting PDF to provide a complete view of the project. This option converts slides that are hidden in the original presentation, ensuring that the final PDF contains all content while maintaining flexibility in your original file.
PresentationToPdfConverterSettings pdfConverterSettings = new PresentationToPdfConverterSettings(); //Enables the inclusion of hidden slides during the conversion process. pdfConverterSettings.ShowHiddenSlides = true; PdfDocument pdfDocument = PresentationToPdfConverter.Convert(pptxDoc, pdfConverterSettings);
Refer to the following image.
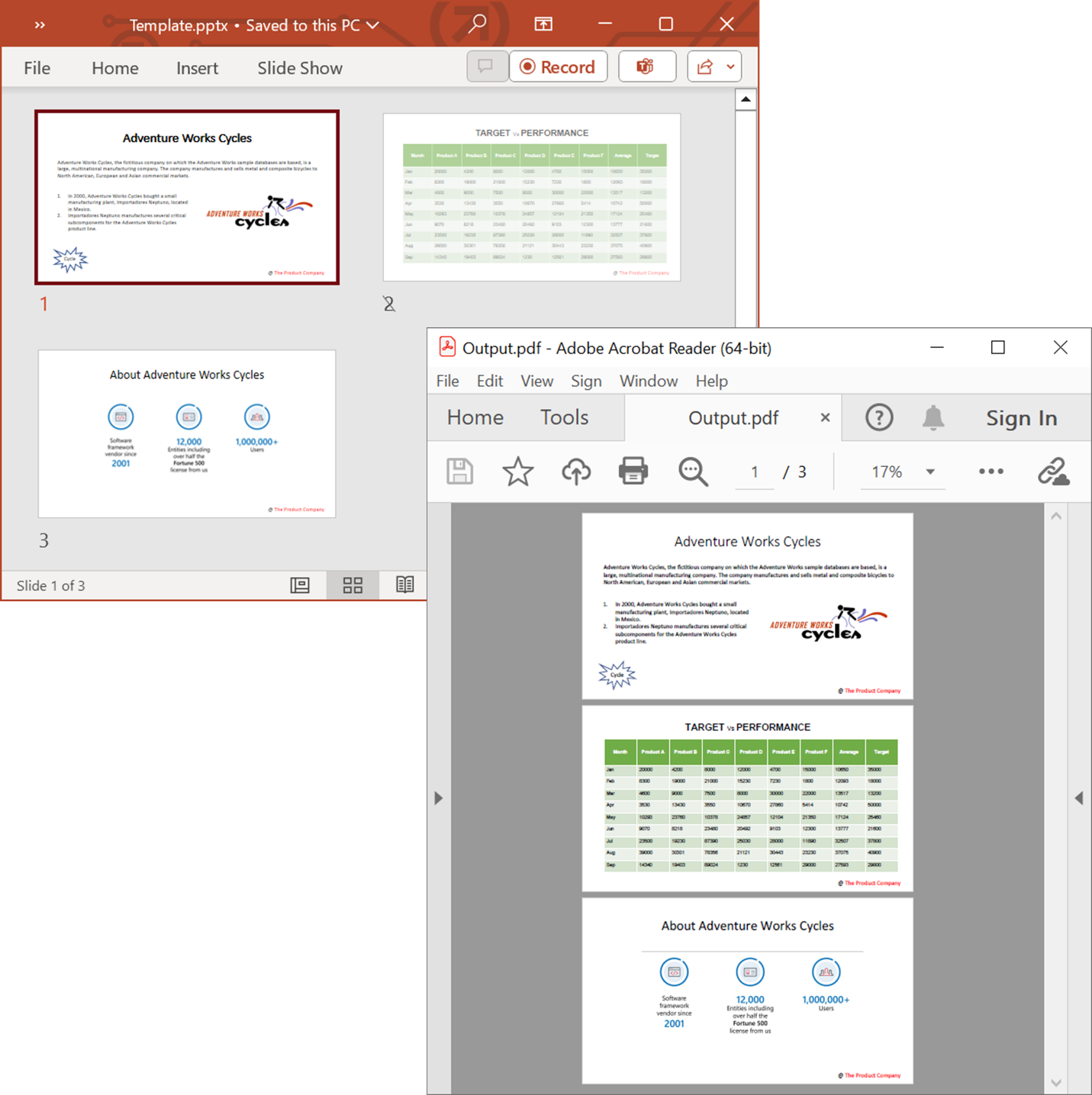
Optimize identical images
File size can be a limitation when distributing large PDFs via email or cloud services. Optimizing the size ensures smoother sharing and faster loading without compromising the document’s quality. This option optimizes identical images, such as repeated logos, reducing the file size and memory usage during conversion, resulting in a more efficient, smaller PDF.
Refer to the following code example to optimize identical images in PowerPoint to PDF conversion.
PresentationToPdfConverterSettings pdfConverterSettings = new PresentationToPdfConverterSettings(); //Set true to optimize the memory usage for identical images. pdfConverterSettings.OptimizeIdenticalImages = true; PdfDocument pdfDocument = PresentationToPdfConverter.Convert(pptxDoc, pdfConverterSettings);
Refer to the following image.
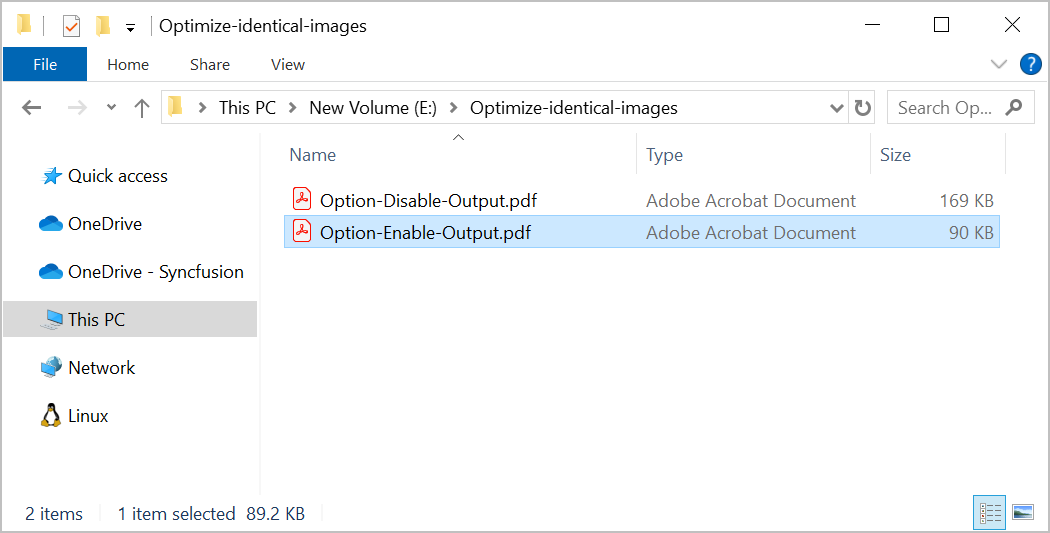

Embark on a virtual tour of Syncfusion's PDF Library through interactive demos.
Fallback fonts
When converting PowerPoint presentations to PDF, missing glyphs from the specified font can lead to improperly rendered text. To resolve this, the .NET PowerPoint library allows users to specify fallback fonts. When the primary font does not contain the necessary glyph, the library automatically switches to a fallback font, ensuring the text is correctly rendered in the output PDF. This is particularly useful for presentations with multilingual content or uncommon characters.
Users can configure fallback fonts in the following ways:
- Initialize default fallback fonts.
- Set custom fonts as fallback fonts for specific script types, such as Arabic, Hebrew, Chinese, Japanese, and more.
- Set custom fonts as fallback fonts for a particular range of Unicode text.
This ensures accurate rendering of the text across different languages and scripts in the final PDF output.
Initialize default fallback fonts
When a presentation contains text in scripts like Arabic or Chinese, missing fonts can cause incorrect rendering. By initializing default fallback fonts, you ensure text is displayed correctly using the pre-defined fonts for each script. This is essential for businesses with multilingual presentations.
Use the InitializeDefault API to apply pre-defined fallback fonts for specific scripts during conversion.
//Open the PowerPoint presentation file stream. using (FileStream fileStreamInput = new FileStream(“Template.pptx”, FileMode.Open, FileAccess.Read)) { //Open the existing PowerPoint presentation with the loaded stream. using (IPresentation pptxDoc = Presentation.Open(fileStreamInput)) { //Initialize the default fallback fonts collection. pptxDoc.FontSettings.FallbackFonts.InitializeDefault(); //Convert PowerPoint into PDF document. using (PdfDocument pdfDocument = PresentationToPdfConverter.Convert(pptxDoc)) { //Save the PDF file to the file system. using (FileStream outputStream = new FileStream(“Output.pdf”, FileMode.Create, FileAccess.ReadWrite)) { pdfDocument.Save(outputStream); } } } }
Refer to the following image.
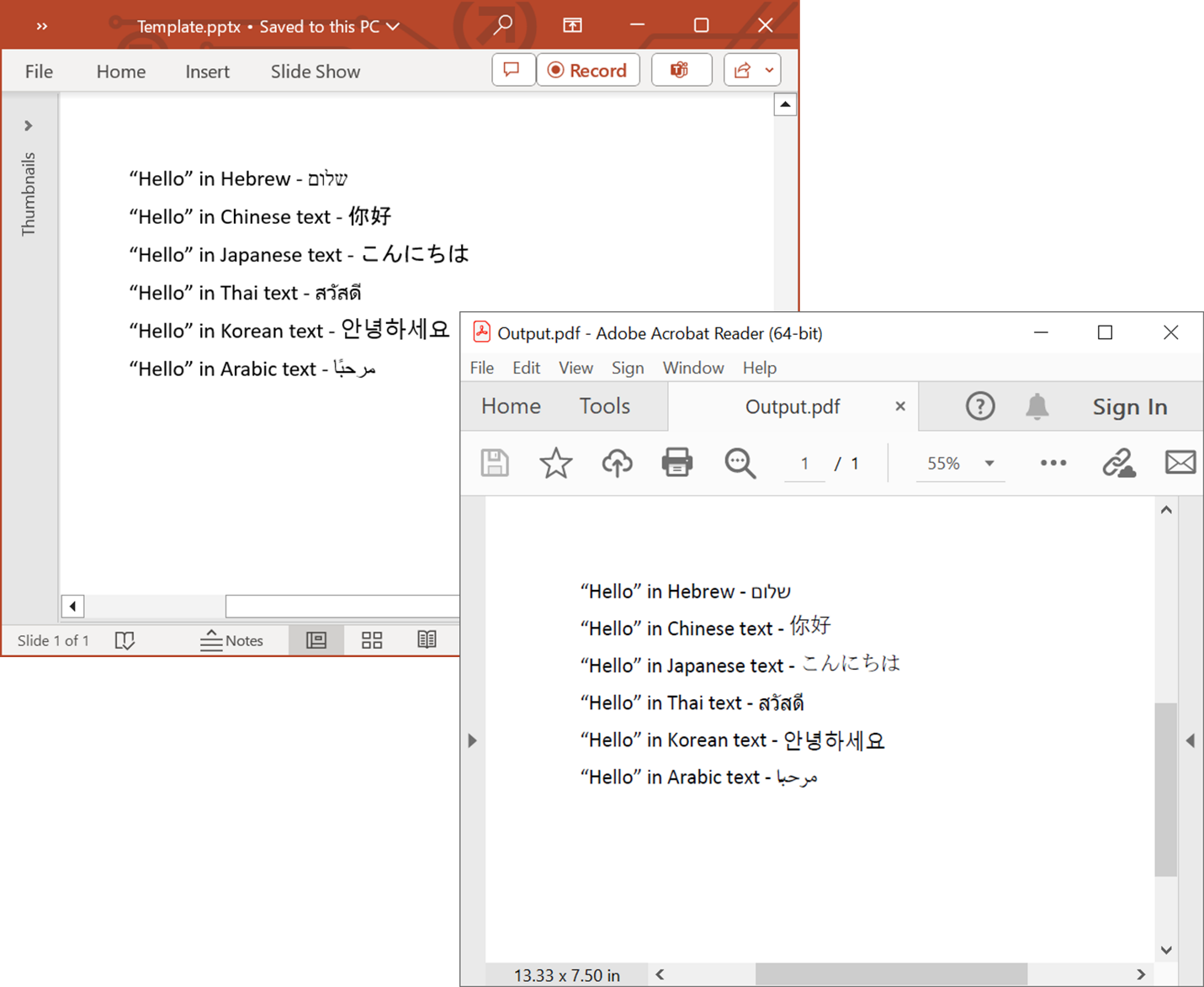
Fallback fonts based on script type
Different scripts require specific fonts for proper rendering when working with multilingual presentations. For instance, Arabic text may need one font, while Hebrew or Chinese requires a different font to ensure clarity and consistency. By setting fallback fonts based on the script type, you can ensure that each script is displayed with the appropriate font, preserving the professional look of your presentation.
You can easily add fallback fonts for various script types, such as Arabic, Hebrew, Chinese, Japanese, Thai, and Korean. Here’s how to do it:
//Open the PowerPoint presentation file stream. using (FileStream inputStream = new FileStream(“Template.pptx”, FileMode.Open, FileAccess.Read)) { //Open the existing PowerPoint presentation with the loaded stream. using (IPresentation pptxDoc = Presentation.Open(inputStream)) { //Add fallback font for "Arabic" script type. pptxDoc.FontSettings.FallbackFonts.Add(ScriptType.Arabic, "Arial, Times New Roman"); //Add fallback font for "Hebrew" script type. pptxDoc.FontSettings.FallbackFonts.Add(ScriptType.Hebrew, "Arial, Courier New"); //Add fallback font for "Chinese" script type. pptxDoc.FontSettings.FallbackFonts.Add(ScriptType.Chinese, "DengXian, MingLiU"); //Add fallback font for "Japanese" script type. pptxDoc.FontSettings.FallbackFonts.Add(ScriptType.Japanese, "Yu Mincho, MS Mincho"); //Add fallback font for "Thai" script type. pptxDoc.FontSettings.FallbackFonts.Add(ScriptType.Thai, "Tahoma, Microsoft Sans Serif"); //Add fallback font for "Korean" script type. pptxDoc.FontSettings.FallbackFonts.Add(ScriptType.Korean, "Malgun Gothic, Batang"); //Convert PowerPoint into PDF document. using (PdfDocument pdfDocument = PresentationToPdfConverter.Convert(pptxDoc)) { //Save the PDF file to the file system. using (FileStream outputStream = new FileStream(“Output.pdf”, FileMode.Create, FileAccess.ReadWrite)) { pdfDocument.Save(outputStream); } } } }
Refer to the following image.
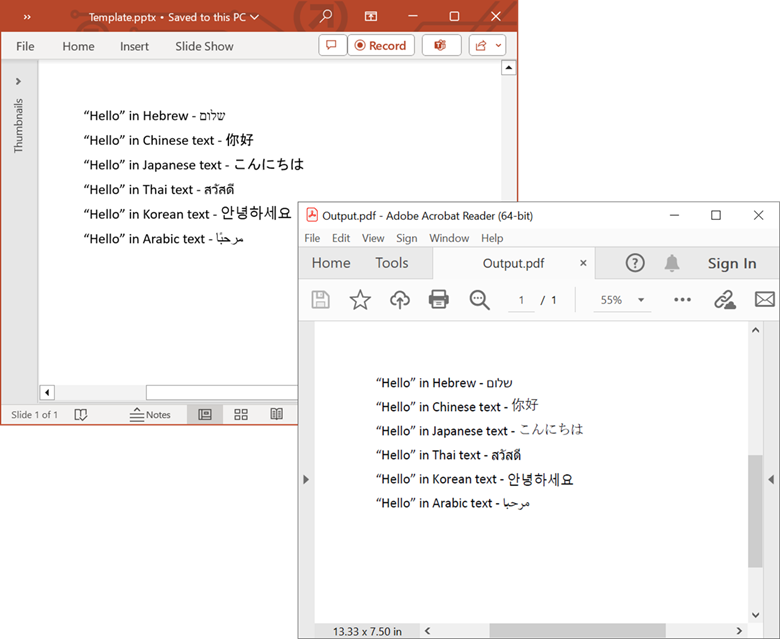
Fallback fonts for a range of Unicode text
For multilingual PowerPoint presentations, you can specify fallback fonts for different Unicode ranges to ensure proper rendering of scripts like Arabic, Chinese, or Japanese.
Here’s how to set fallback fonts for specific Unicode ranges:
//Open the PowerPoint presentation file stream. using (FileStream inputStream = new FileStream(“Template.pptx”, FileMode.Open, FileAccess.Read)) { //Open the existing PowerPoint presentation with the loaded stream. using (IPresentation pptxDoc = Presentation.Open(inputStream)) { //Add fallback font for specific Unicode range. // Arabic. pptxDoc.FontSettings.FallbackFonts.Add(new FallbackFont(0x0600, 0x06ff, "Arial")); // Hebrew. pptxDoc.FontSettings.FallbackFonts.Add(new FallbackFont(0x0590, 0x05ff, "Arial")); // Hindi. pptxDoc.FontSettings.FallbackFonts.Add(new FallbackFont(0x0900, 0x097F, "Mangal")); // Chinese. pptxDoc.FontSettings.FallbackFonts.Add(new FallbackFont(0x4E00, 0x9FFF, "DengXian")); // Japanese. pptxDoc.FontSettings.FallbackFonts.Add(new FallbackFont(0x3040, 0x309F, "MS Mincho")); // Thai. pptxDoc.FontSettings.FallbackFonts.Add(new FallbackFont(0x0E00, 0x0E7F, "Tahoma")); // Korean. pptxDoc.FontSettings.FallbackFonts.Add(new FallbackFont(0xAC00, 0xD7A3, "Malgun Gothic")); //Convert PowerPoint into PDF document. using (PdfDocument pdfDocument = PresentationToPdfConverter.Convert(pptxDoc)) { //Save the PDF file to the file system. using (FileStream outputStream = new FileStream(“Output.pdf”, FileMode.Create, FileAccess.ReadWrite)) { pdfDocument.Save(outputStream); } } } }
Refer to the following image.
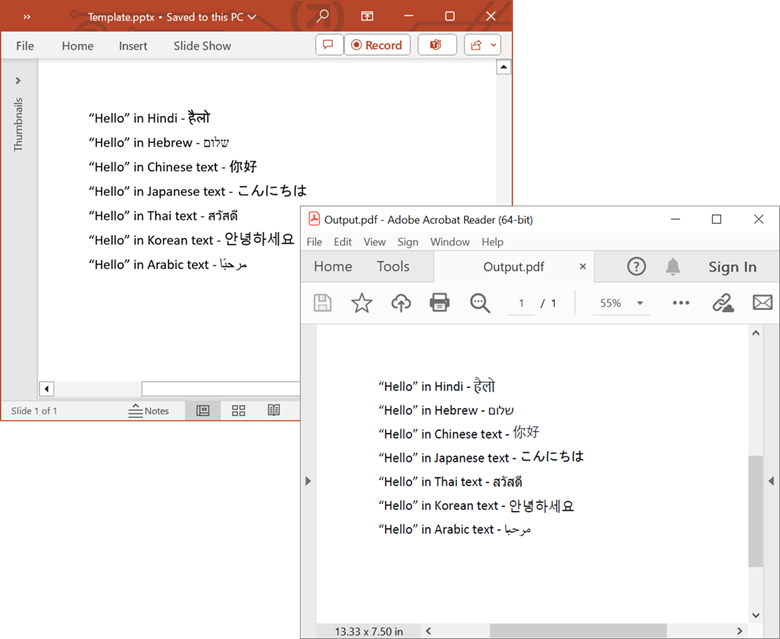
Font substitution
When converting PowerPoint presentations to PDFs, the fonts used in the presentation must be installed on the machine when the conversion is performed. If the required fonts are missing, our .NET PowerPoint Library uses a default font, which may lead to discrepancies in the rendered PDF due to differences in font glyphs. This can cause layout and design issues, especially for presentations relying on specific fonts.
To avoid this problem, we offer the ability to specify alternate fonts when the original fonts are unavailable.
Use alternate fonts from installed fonts
Consider an enterprise deploying a document automation solution that generates PDFs from PowerPoint templates. The presentation uses custom fonts, but these fonts are not installed on all production machines. The layout of the generated PDFs could vary significantly if fallback fonts like Microsoft Sans Serif are used by default.
By handling the SubstituteFont event in the .NET PowerPoint Library, users can substitute missing fonts with appropriate alternatives from the installed fonts. This ensures that the visual integrity of the presentation is preserved, even if the exact font is unavailable.
Refer to the following code example.
//Open the PowerPoint presentation file stream. using (FileStream inputStream = new FileStream(“Template.pptx”, FileMode.Open, FileAccess.ReadWrite)) { //Load an existing PowerPoint Presentation. using (IPresentation pptxDoc = Presentation.Open(inputStream)) { //Hook the font substitution event to handle unavailable fonts. //This event will be triggered when a font used in the document is not found in the production environment. pptxDoc.FontSettings.SubstituteFont += FontSettings_SubstituteFont; //Convert PowerPoint into PDF document. using (PdfDocument pdfDocument = PresentationToPdfConverter.Convert(pptxDoc)) { //Unhook the font substitution event after the conversion is complete. pptxDoc.FontSettings.SubstituteFont -= FontSettings_SubstituteFont; //Save the PDF file to the file system. using (FileStream outputStream = new FileStream(“Output.pdf”, FileMode.Create, FileAccess.ReadWrite)) { pdfDocument.Save(outputStream); } } } }
In the following code example, the event handler substitutes missing fonts with available fonts installed on the machine during PowerPoint to PDF conversion. If the font Arial Unicode MS is missing, it is replaced with the installed Arial font. For other missing fonts, it uses the installed Times New Roman font.
static void FontSettings_SubstituteFont(object sender, SubstituteFontEventArgs args) { //Check if the original font is "Arial Unicode MS" and substitute it with "Arial". if (args.OriginalFontName == "Arial Unicode MS") args.AlternateFontName = "Arial"; else //Subsitutue "Times New Roman" for any other missing fonts. args.AlternateFontName = "Times New Roman"; }
Refer to the following image.
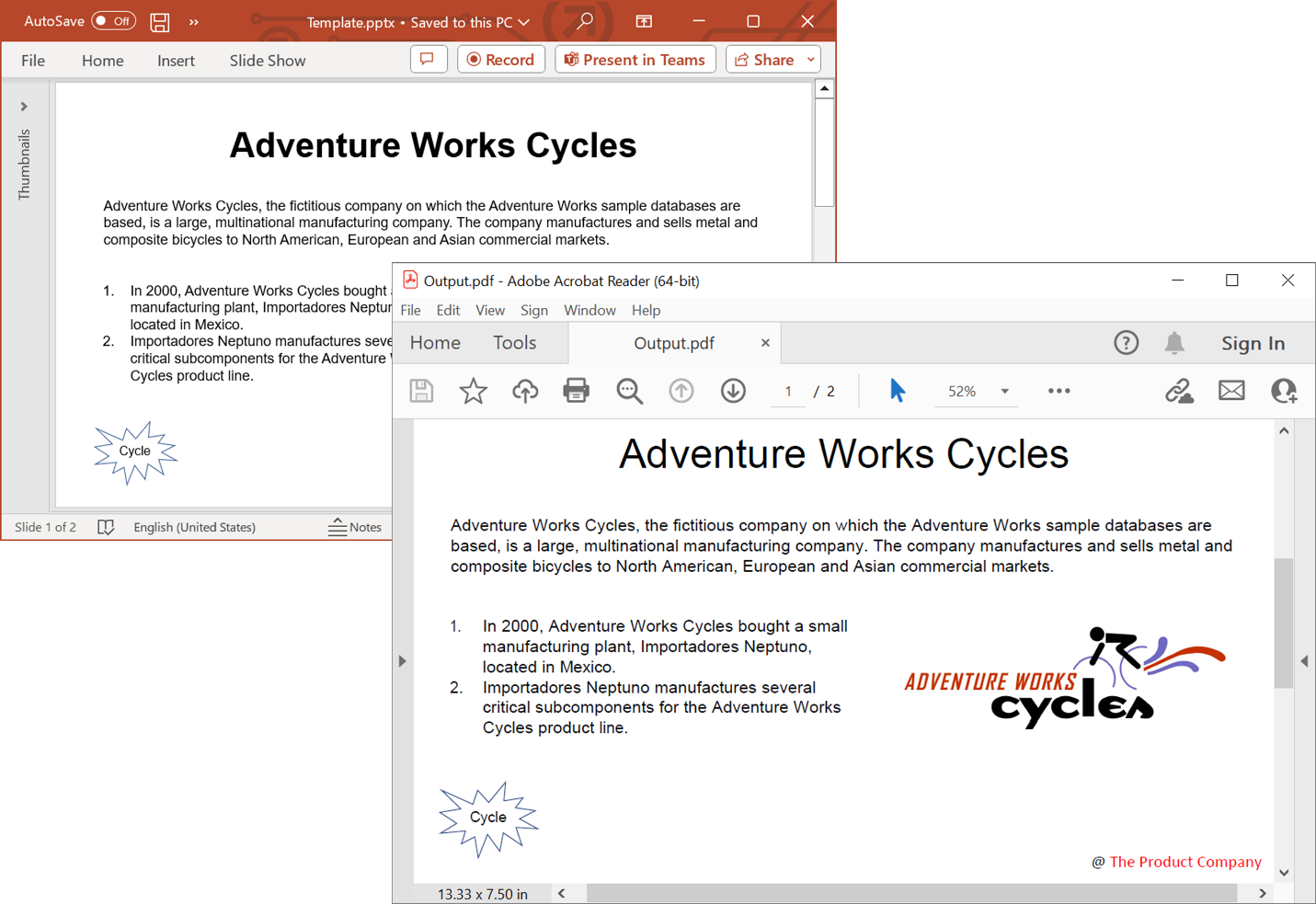

Unleash the full potential of Syncfusion's PDF Library! Explore our advanced resources and empower your apps with cutting-edge functionalities.
Use alternate font without installing
In restricted environments where installing fonts on deployment machines is not allowed, our .NET PowerPoint Library allows fonts to be used without installation. By providing a font stream through the SubstituteFont event during PowerPoint to PDF conversion, you can ensure the presentation’s design is maintained without violating deployment policies. This is ideal for scenarios involving proprietary or custom fonts in environments with strict IT restrictions.
Refer to the following code example.
//Open the PowerPoint presentation file stream. using (FileStream inputStream = new FileStream("Template.pptx", FileMode.Open, FileAccess.ReadWrite)) { //Load an existing PowerPoint Presentation. using (IPresentation pptxDoc = Presentation.Open(inputStream)) { //Hook the font substitution event to handle unavailable fonts. //This event will be triggered when a font used in the document is not found in the production environment. pptxDoc.FontSettings.SubstituteFont += FontSettings_SubstituteFont; //Convert PowerPoint into PDF document. using (PdfDocument pdfDocument = PresentationToPdfConverter.Convert(pptxDoc)) { //Unhook the font substitution event after the conversion is complete. pptxDoc.FontSettings.SubstituteFont -= FontSettings_SubstituteFont; //Save the PDF file to the file system. using (FileStream outputStream = new FileStream(“Output.pdf”, FileMode.Create, FileAccess.ReadWrite)) { pdfDocument.Save(outputStream); } } } }
In this method, an appropriate alternate font is substituted when the Arial Unicode MS font is missing in a PowerPoint presentation. Depending on the font style (Italic, Bold, or Regular), a corresponding font file (e.g., Arial_italic.TTF, Arial_bold.TTF, or Arial.TTF) is provided via a file stream.
static void FontSettings_SubstituteFont(object sender, SubstituteFontEventArgs args) { //Check if the original font is "Arial Unicode MS" and substitute it with "Calibri". if (args.OriginalFontName == "Arial Unicode MS") { switch (args.FontStyle) { case FontStyle.Italic: args.AlternateFontStream = new FileStream(@"Data/calibrii.ttf", FileMode.Open, FileAccess.ReadWrite); break; case FontStyle.Bold: args.AlternateFontStream = new FileStream(@"Data/calibrib.ttf", FileMode.Open, FileAccess.ReadWrite); break; default: args.AlternateFontStream = new FileStream(@"Data/calibri.ttf", FileMode.Open, FileAccess.ReadWrite); break; } } else //Subsitutue "Times New Roman" for any other missing fonts. args.AlternateFontStream = new FileStream(@"Data/times.ttf", FileMode.Open, FileAccess.ReadWrite); }
Refer to the following image.
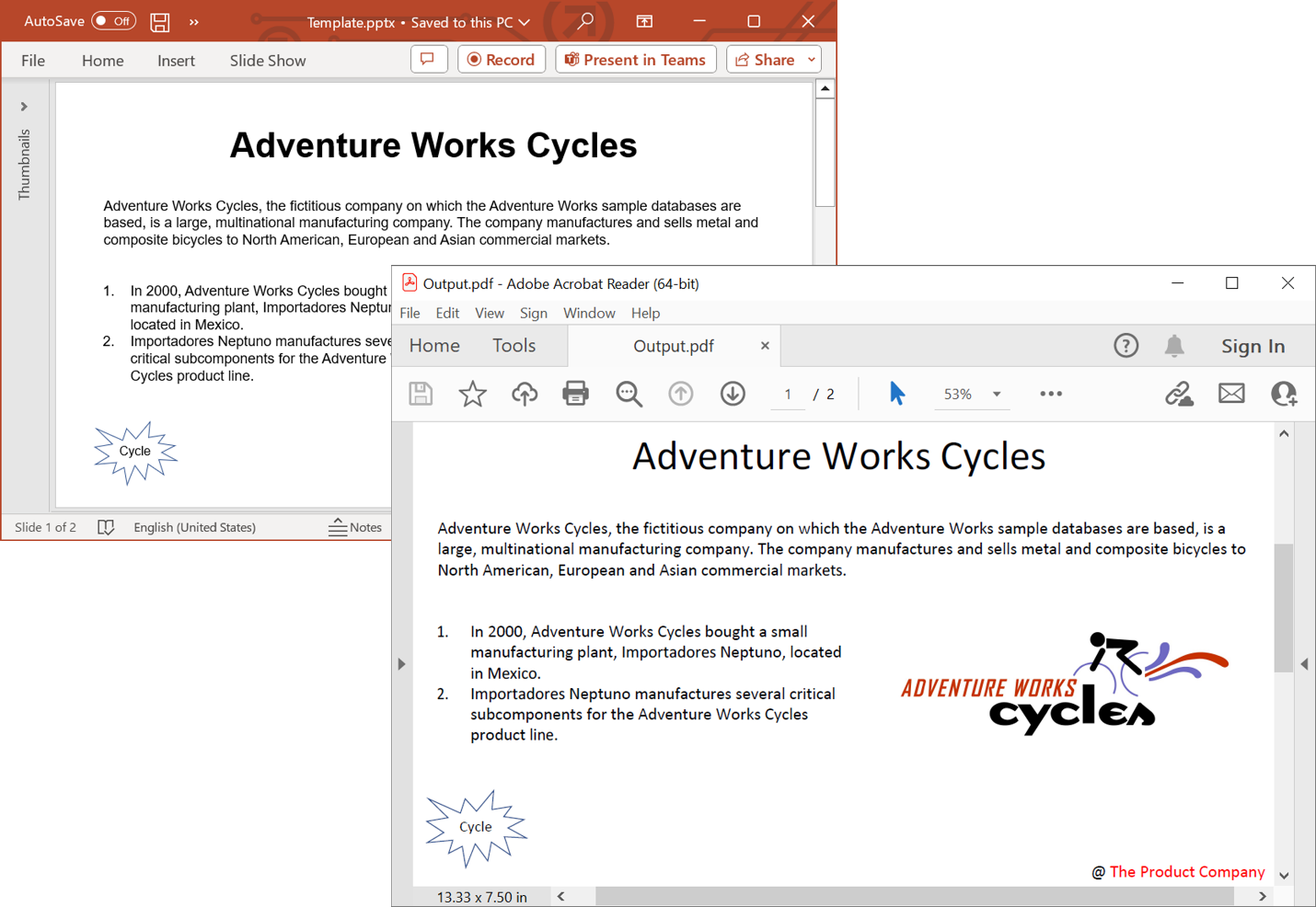
GitHub reference
For more details, refer to the PowerPoint to PDF conversion with advanced options in C# using the .NET PowerPoint Library GitHub demo.

Syncfusion’s high-performance PDF Library allows you to create PDF documents from scratch without Adobe dependencies.
Conclusion
Thanks for reading! Syncfusion .NET PowerPoint Library provides customizable settings to optimize the PowerPoint to PDF conversion process. It supports PDF/UA for accessibility and PDF/A for archiving, including options for handouts, note pages, and hidden slides. Whether you’re working with PowerPoint presentations on any .NET platform, the PowerPoint to PDF converter streamlines the conversion process for .NET developers.
Take a moment to peruse the PowerPoint to PDF conversion documentation, where you can find other options and features, all with code examples.
Are you already a Syncfusion user? You can download the product setup here. If you’re not yet a Syncfusion user, you can download a 30-day free trial.
If you have questions, contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!