We are excited to introduce the busy indicator drawing helper for Windows Forms. This busy indicator drawing helper has been implemented in our 2018 Volume 2 release. Usually, an animated GIF image cannot be shown in a Windows Forms control without using the picture box control or writing our own custom control. The busy indicator drawing helper helps you show animated GIF images in a control. The main advantage of this helper is that you can use it in any Windows Forms control, regardless of whether it is a Syncfusion control.
This blog explores how you can show animated GIF images using the busy indicator drawing helper in Windows Forms controls.
Getting started
public partial class Form1 : Form { BusyIndicator busyIndicator = new BusyIndicator(); ObservableCollection<int> sampleData = new ObservableCollection<int>(); public Form1() { InitializeComponent(); } private void sfButton1_Click(object sender, EventArgs e) { this.sfButton1.Text = string.Empty; busyIndicator.Show(this.sfButton1); for (int i = 0; i <= 10000000; i++) { sampleData.Add(i); } busyIndicator.Hide(); this.sfButton1.Text = "Get items"; sampleData.Clear(); } }
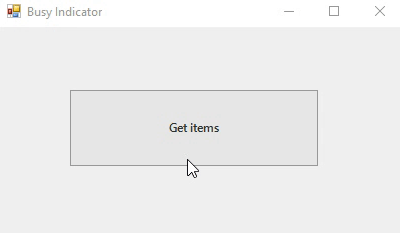
public partial class Form1 : Form { BusyIndicator busyIndicator = new BusyIndicator(); ObservableCollection<int> sampleData = new ObservableCollection<int>(); public Form1() { InitializeComponent(); } private void sfButton1_Click(object sender, EventArgs e) { this.sfButton1.Text = "Loading"; busyIndicator.Show(this.sfButton1, new Point((this.sfButton1.Width / 2) + this.busyIndicator.Image.Width, (this.sfButton1.Height / 2) - this.busyIndicator.Image.Height / 2)); for (int i = 0; i <= 10000000; i++) { sampleData.Add(i); } busyIndicator.Hide(); this.sfButton1.Text = "Get items"; sampleData.Clear(); } }
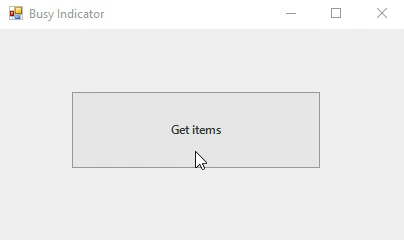
Showing busy indicator in asynchronous mode
BackgroundWorker backgroundWorker = new BackgroundWorker(); BusyIndicator busyIndicator = new BusyIndicator(); ObservableCollection<int> sampleData = new ObservableCollection<int>(); public Form1() { InitializeComponent(); backgroundWorker.WorkerSupportsCancellation = true; backgroundWorker.DoWork += DoWork; backgroundWorker.RunWorkerCompleted += RunWorkerCompleted; } private void DoWork(object sender, DoWorkEventArgs e) { this.sfButton1.Invoke(new Action(() => this.sfButton1.Text = string.Empty)); busyIndicator.Show(this.sfButton1); for (int i = 0; i <= 10000000; i++) { sampleData.Add(i); } } private void RunWorkerCompleted(object sender, RunWorkerCompletedEventArgs e) { this.sfButton1.Text = "Get items"; if (!backgroundWorker.IsBusy) backgroundWorker.CancelAsync(); busyIndicator.Hide(); sampleData.Clear(); } private void sfButton1_Click(object sender, EventArgs e) { backgroundWorker.RunWorkerAsync(); }
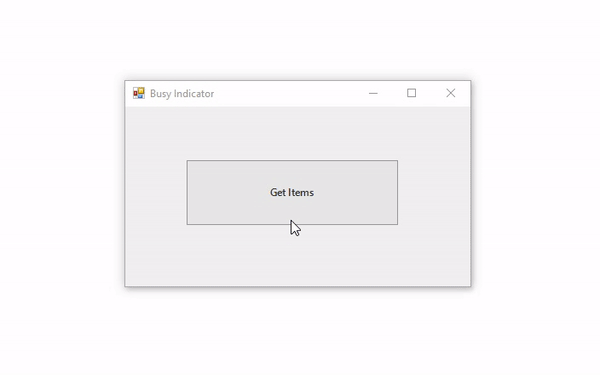
Built-in busy indicator in data grid
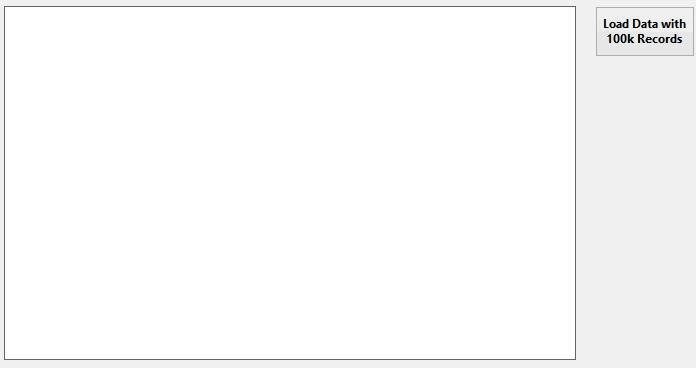
Summary
In this blog, we hope you enjoyed learning how to show the busy indicator in a Windows Forms control. You can also refer to this documentation to learn about additional options provided in the busy indicator. If you’re already a Syncfusion user, you can download the Windows Forms setup on Direct-Trac. If you’re not yet a Syncfusion user, you can download a free, 30-day trial on our website. Give us your feedback in the comments section below, our online forum, or a support ticket.
If you like this blog post, we think you’ll also like the following free e-books: