TL;DR: Create interactive floor planners using the JavaScript Diagram library. Start by setting up a basic web app, including necessary scripts, and initialize the Diagram library. Use symbol palettes for reusable design elements and leverage features like undo/redo, saving/loading, exporting, and printing to enhance your diagramming experience.
A floor planner is a tool for creating floor plans and room layouts for various purposes, including interior design, architecture, real estate, and facility management. Users can arrange and visualize rooms, walls, furniture, appliances, and objects within a given space.
Syncfusion Javascript Diagram is a powerful and versatile library that allows designers to create interactive and visually appealing diagrams and enables you to build such floor planners with ease.
Let’s see how to use the Javascript Diagram library to create an interactive user interface that allows designers to create floor planners quickly and easily.
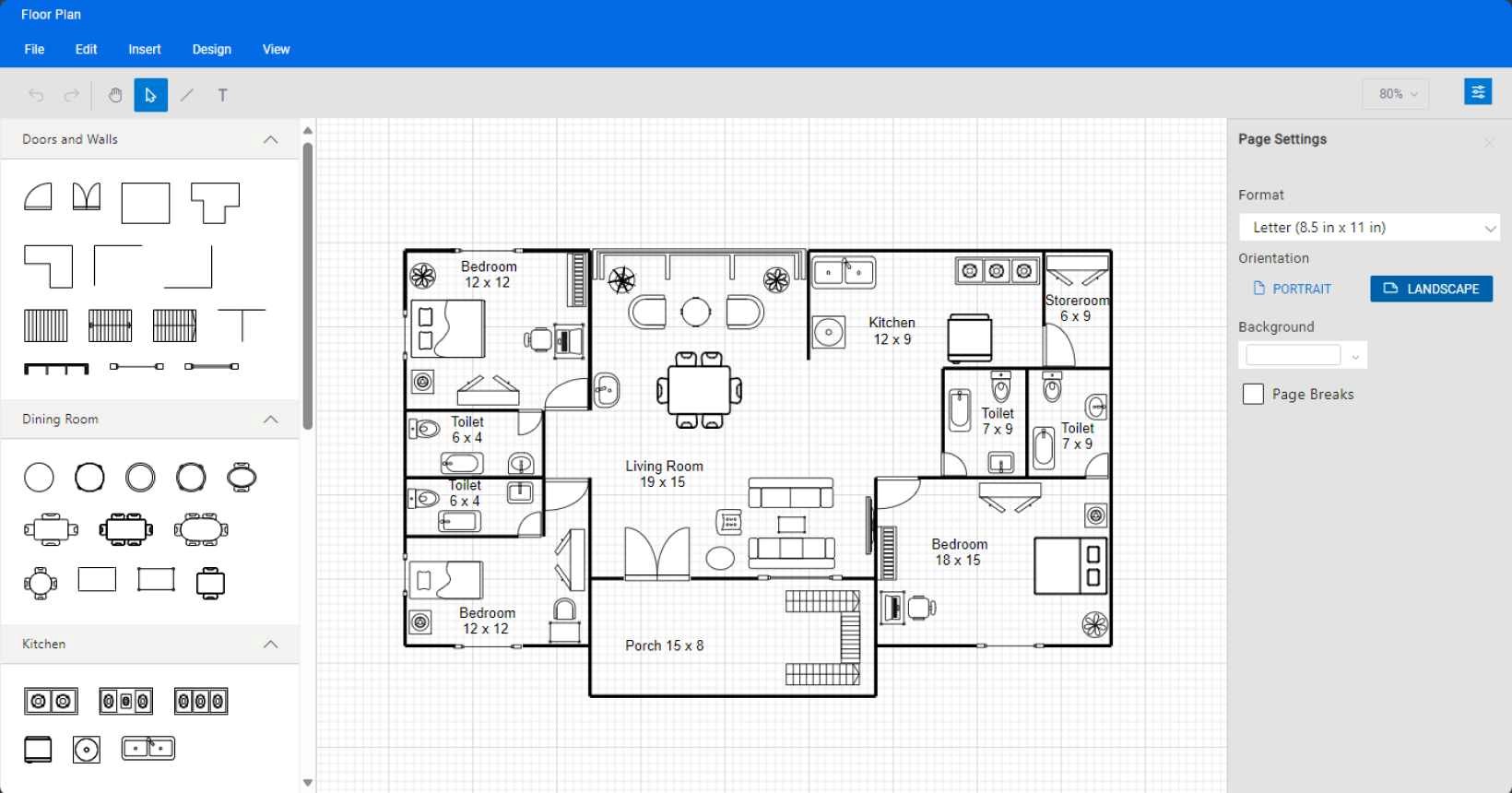
Getting started with JavaScript Diagram library
Follow these steps to add the Syncfusion JavaScript Diagram library to your web app:
Step 1: Create a folder and name it as Floor Planner. Then, add the index.html and index.js files to begin developing your web app.
Step 2: Add the dependent scripts and style CDN reference links for the JavaScript Diagram library in the index.html file. Refer to the following code example.
<head> <title>Essential JS2 for Javascript - Floor Planner</title> <script src="https://cdn.syncfusion.com/ej2/syncfusion-helper.js" type ="text/javascript"></script> <script src="https://cdn.syncfusion.com/ej2/20.4.38/dist/ej2.min.js" type="text/javascript"></script> <link href="https://cdn.syncfusion.com/ej2/20.4.38/fluent.css" rel="stylesheet"> <link href="https://cdn.syncfusion.com/ej2/ej2-buttons/styles/fluent.css" rel="stylesheet"> <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet"> <link href="https://cdn.syncfusion.com/ej2/20.4.38/ej2-base/styles/fluent.css" rel="stylesheet"> <link href="https://cdn.syncfusion.com/ej2/20.4.38/ej2-popups/styles/fluent.css" rel="stylesheet"> <link href="https://cdn.syncfusion.com/ej2/20.4.38/ej2-splitbuttons/styles/fluent.css" rel="stylesheet"> <link href="https://cdn.syncfusion.com/ej2/20.4.38/ej2-navigations/styles/fluent.css" rel="stylesheet"> <link href="https://cdn.syncfusion.com/ej2/20.4.38/ej2-inputs/styles/fluent.css" rel="stylesheet"> <link href="https://cdn.syncfusion.com/ej2/20.4.38/ej2-dropdowns/styles/fluent.css" rel="stylesheet"> <link href="./assets/Diagram_Builder_icon_v4/Diagram_Builder_Icon/style.css" rel="stylesheet"> <link href="./assets/index.css" rel="stylesheet"> <link href="./assets/dbstyle/diagrambuilder.css" rel="stylesheet"/> </head>
Step 3: Create an HTML div element that will act as the container for the JavaScript Diagram component in the index.html file.
<div class="db-diagram-container"> <div id="diagramContainerDiv" class='db-current-diagram-container'> <div id="diagram"></div> </div> </div> <script src="./scripts/index.js" type="text/javascript"></script>
Step 4: Initialize the JavaScript Diagram component by calling the ej.diagrams.Diagram() constructor method and pass the required arguments, such as the width and height, to render an empty diagram. Then, add the following code to the index.js file.
//Initialize the JavaScript Diagram component. var diagram = new ej.diagrams.Diagram({ width: '100%', height: '100%', }); diagram.appendTo('#diagram');
The following screenshot shows the diagramming page that was created.
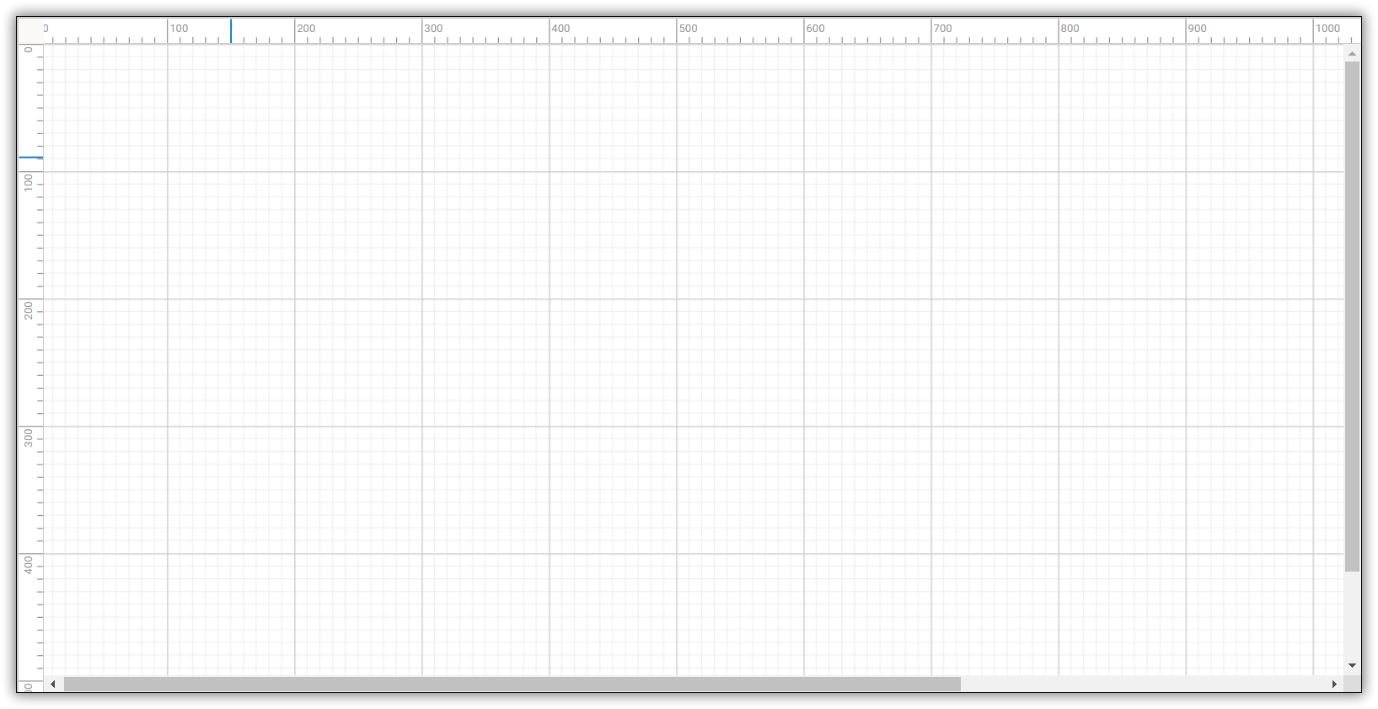
Note: For more details, refer to getting started with JavaScript Diagram component documentation.
Floor planner diagram symbols
A floor planner designer provides a variety of symbols, such as doors, walls, dining, kitchen, living rooms, and other components, to design floor planner diagrams.
Creating reusable floor planner symbols
The Javascript Diagram component provides a gallery of reusable nodes and connectors called SymbolPalettes. These palettes display a collection of shapes, and each palette showcases a set of nodes and connectors. We can drag and drop the symbols onto the diagram canvas as needed.
Follow these steps to create a diagram symbol palette with floor planner shapes:
Step 1: First, create an HTML div element that will contain the Diagram’s symbol palette.
<div class="db-palette-parent"> <div id="symbolpalette"></div> </div>
Step 2: Initialize the Syncfusion JavaScript Diagram’s symbol palette with properties such as width, height, symbolHeight, symbolWidth, and the collection of symbols to be included in the palettes. Also, define the getNodeDefaults function to include the default properties of the node.
//Initialize the symbol palette. var palette = new ej.diagrams.SymbolPalette({ expandMode: 'Multiple' , enableSearch: true , width: '100%', height: '100%', palettes: [ { id: 'door', expanded: true, symbols: doors, iconCss: 'e-ddb-icons e-flow', title: 'Doors and Walls' }, { id: 'diningRoom', expanded: true, symbols: diningRoom, iconCss: 'e-ddb-icons e-flow', title: 'Dining Room' }, { id: 'kitchen', expanded: true, symbols: kitchen, iconCss: 'e-ddb-icons e-flow', title: 'Kitchen'}, { id: 'bedRoom', expanded: true, symbols: bedRoom, iconCss: 'e-ddb-icons e-flow', title: 'Bed Room' }, { id: 'livingRoom', expanded: true, symbols: livingRoom, iconCss: 'e-ddb-icons e-flow', title: 'Living Room' }, { id: 'bathRoom', expanded: true, symbols: bathRoom, iconCss: 'e-ddb-icons e-flow', title: 'Bath Room' }, ], getNodeDefaults: function (node) { if(node.id === 'Door close'|| node.id === 'Double door close') { node.width = 25; node.height = 25; } else if(node.id ==='Circle Study Table'||node.id ==='Circular Table for Two' ||node.id ==='Circle Study Table1' ||node.id ==='Circle Study Table2' ||node.id ==='Circle Study Table3'){ node.width = 27; node.height = 27; } else if(node.id ==='Circle Dining Table'){ node. Width = 30; node. Height = 30 } else if(node.id ==='Rectangle Dining Table'){ node.width = 30; node.height = 30; } else if(node.id ==='Oblong Dining Table' ||node.id ==='Rectangle Dining Table'|| node.id ==='Oval Dining Table' ){ node.width = 50; node.height = 30; } else if(node.id === 'Rectangular Table for Two'){ node.width = 26; node.height = 30; } else if(node.id === 'Rectangle Study Table' || node.id === 'Rectangle Study Table1' ) { node.width = 35; node.height = 22; } else if(node.id ==='Small Gas Range') { node.width = 50; node.height =25; } else if(node.id === 'Large Gas Range' ||node.id ==='Large Gas Range1' ) { node.width = 50; node.height = 25; } else if(node.id ==='Refrigerator' ||node.id ==='Water Cooler') { node.width = 25; node.height = 25; } else if(node.id ==='Double bed' ||node.id ==='Double bed1') { node.width = 36; node.height = 30; } else if(node.id ==='Single bed' ||node.id ==='Single bed1') { node.width = 19; node.height = 30; } else if(node.id ==='Book Case' ) { node.width = 40; node.height = 20; } else if(node.id ==='Warddrobe1'){ node.width = 35; node.height = 30; } else if(node.id ==='Warddrobe') { node.width = 35; node.height = 20; } else if(node.id ==='Matte'||node.id ==='Matte1') { node.width = 20; node.height = 10; } else if(node.id ==='Large Plant' ||node.id ==='Small Plant'||node.id ==='Lamp light') { node.width = 20; node.height =20; } else if(node.id ==='TV') { node.width =40; node.height =12; } else if(node.id ==='Flat TV' ||node.id ==='Flat TV1') { node.width = 65; node.height =12; } else if(node.id ==='Chair' ||node.id ==='Chair1') { node.width = 30; node.height =25; } else if(node.id ==='Sofa' ||node.id ==='Double Sofa') { node.width = 50; node.height =25; } else if(node.id ==='Single Sofa'|| node.id ==='Couch') { node.width = 25; node.height = 25; } else if(node.id ==='Lounge'|| node.id ==='Piano') { node.width = 55; node.height = 25; } else if( node.id ==='Stool' ) { node.width = 24; node.height =24; } else if(node.id ==='Printer'||node.id ==='Laptop') { node.width = 25; node.height =25; } else if(node.id ==='Window1' || node.id ==='Window2') { node.width = 50; node.height =5; } else if( node.id ==='Window Garden' ) { node.width = 60; node.height =10; } else if(node.id ==='L Room' || node.id ==='Wall Corner1'){ node.width = 45; node.height =40; } else if(node.id ==='T Wall'){ node.width = 45; node.height = 30; } else if(node.id ==='Room' || node.id ==='T Room' || node.id ==='Wall Corner'|| node.id ==='Elevator') { node.width = 45; node.height =38; } else if(node.id ==='Staircase' || node.id ==='Staircase1' || node.id ==='Staircase2' ) { node.width = 40; node.height =30; } else if(node.id === 'Toilet1' || node.id === 'Toilet2'){ node.width = 27; node.height = 35; } else if(node.id === 'Corner Shower'|| node.id === 'Shower'){ node.width = 36; node.height = 35; } else if(node.id === 'Wash Basin1'){ node.width = 25; node.height = 25; } else if(node.id === 'Wash Basin2' || node.id === 'Wash Basin3'|| node.id === 'Wash Basin5' || node.id === 'Wash Basin6'){ node.width = 30; node.height = 22; } else if(node.id === 'Double Sink4' || node.id === 'Double Sink'|| node.id === 'Double Sink1' || node.id === 'Double Sink2'){ node.width = 50; node.height = 22; } else if(node.id === 'Refrigerator'|| node.id === 'Water Cooler' ) { node.width = 30; node.height = 30; } else if(node.id === 'Bath Tub' || node.id === 'Bath Tub1' || node.id === 'Bath Tub2' || node.id === 'Bath Tub3' || node.id === 'Bath Tub4'){ node.width = 40; node.height =22; } else { node.width = 50; node.height = 50; } }, symbolMargin: { left: 5, right: 5, top: 5, bottom: 5 }, getSymbolInfo: function (symbol) { return { tooltip : symbol.addInfo}; } }); palette.appendTo('#symbolpalette');
Step 3: Then, define and customize your palette symbols as needed.
//Path values of the shapes in the symbol palette var doors = [ { id: 'Door close',addInfo:'Door close', shape: { type: 'Path', data: 'M1 71L1 79L72 79V71M1 71L72 71M1 71C1 32.3401 32.3401 1 71 1H72V71' }}, { id: 'Double door close',addInfo:'Double door close', shape: { type: 'Path', data:"M143 71V79L1 79L1 71M143 71L1 71M143 71V1H142C103.34 1 72 32.3401 72 71M143 71H72M1 71L1 1H2C40.6599 1 72 32.3401 72 71M1 71L72 71M72 78.5V71"}}, { id:'Room',addInfo:'Room', shape :{ type: 'Path', data:"M4 4H104V104H4V4Z"}}, { id:'T Room',addInfo:'T Room', shape :{ type: 'Path', data:"M4 4H273V145.749H192.981V250H71.25V110.312H4V4Z"}}, { id:'L Room',addInfo:'L Room', shape :{ type: 'Path', data:"M4 4H273V250H146.5V110.312H4V4Z"}}, { id:'Wall Corner',addInfo:'Wall Corner', shape :{ type: 'Path', data:"M104 4H4V104"}}, { id:'Wall Corner1',addInfo:'Wall Corner', shape :{ type: 'Path',data:'M0 100L100 100L100 0'}}, { id:'T Wall',addInfo:'T Wall', shape :{ type: 'Path', data:"M106 4.00002L206 4.00004M106 4.00002L106 104M106 4.00002L0 4"}}, { id:'Window Garden',addInfo:'Window Garden',dragSize:{width:76,height:29}, shape :{ type: 'Path', data:"M1.5 0H0V1.5V38.5H3V3H152V38.5H155V1.5V0H153.5H1.5ZM6.5 5H5V6.5V38.5H8V8H53V38.5H56V8H100V38.5H103V8H147V38.5H150V6.5V5H148.5H103H101.5H100H56H54.5H53H6.5Z"}}, { id:'Window1',addInfo:'Window',dragSize:{width:66,height:7}, shape :{ type: 'Path', data:'M22 5.5L124 5.50001M145 9L128 9V1L145 1V9ZM1 1L18 1L18 9L1 9L1 1ZM128 8L124 8V2L128 2V8ZM18 2L22 2L22 8L18 8L18 2Z'}}, { id:'Window2',addInfo:'Window',dragSize:{width:66,height:7}, shape :{ type: 'Path', data:"M22 3.5L124 3.50001M22 6.5L124 6.50001M145 9L128 9V1L145 1V9ZM1 1L18 1L18 9L1 9L1 1ZM128 8L124 8V2L128 2V8ZM18 2L22 2L22 8L18 8L18 2Z"}}, . . . . . . . . . . . . ]; var diningRoom = [ { id:'Circle Study Table',addInfo:'Circle Study Table', dragSize:{height:40,width:40}, shape :{ type: 'Path', data:"M57 29C57 44.464 44.464 57 29 57C13.536 57 1 44.464 1 29C1 13.536 13.536 1 29 1C44.464 1 57 13.536 57 29Z"}}, { id:'Circle Study Table2',addInfo:'Circle Study Table',dragSize:{height:40,width:40}, shape :{ type: 'Path', data:'M57 29C57 44.464 44.464 57 29 57C13.536 57 1 44.464 1 29C1 13.536 13.536 1 29 1C44.464 1 57 13.536 57 29Z M53 29C53 42.2548 42.2548 53 29 53C15.7452 53 5 42.2548 5 29C5 15.7452 15.7452 5 29 5C42.2548 5 53 15.7452 53 29Z'}}, { id:'Circular Table for Two',addInfo:'Dual Circle Study Table',shape :{ type: 'Path', data:"M12.7839 6.68319C12.3695 3.67862 14.704 1 17.737 1H33.4357C36.4079 1 38.7239 3.57736 38.4072 6.53267L37.5 14.7962C33 12.2962 30.4479 12.2502 25.5 12.0049C20.8774 12.1817 18.4551 13.0876 13.9551 15.0876L12.7839 6.68319Z M32 2C32 4.20914 30.2091 6 28 6L23 6C20.7909 6 19 4.20914 19 2V1L32 1V2Z M38.6314 67.656C38.8308 70.546 36.5401 73 33.6433 73H17.5439C14.579 73 12.2654 70.4347 12.5705 67.4855L13.4604 58.6325C17.7149 60.6639 20.3595 61.8118 25.5 61.9951C30.7634 61.8969 34.0464 60.4596 38.1993 58.8266L38.6314 67.656Z M19 72C19 69.7909 20.7909 68 23 68H28C30.2091 68 32 69.7909 32 72V73H19V72Z M51 37C51 50.8071 39.8071 62 26 62C12.1929 62 1 50.8071 1 37C1 23.1929 12.1929 12 26 12C39.8071 12 51 23.1929 51 37Z M48 37C48 49.1503 38.1503 59 26 59C13.8497 59 4 49.1503 4 37C4 24.8497 13.8497 15 26 15C38.1503 15 48 24.8497 48 37Z"}}, { id:'Rectangle Dining Table',addInfo:'Rectangle Dining Table', dragSize:{width:60,height:40},shape :{ type: 'Path', data: 'M26.5284 6.47992C26.2452 3.54248 28.5543 1 31.5053 1H47.4947C50.4457 1 52.7548 3.54248 52.4716 6.47992L51.65 15L27.35 15L26.5284 6.47992Z M46 2C46 4.20914 44.2091 6 42 6L37 6C34.7909 6 33 4.20914 33 2V1L46 1V2Z M52.4716 73.5201C52.7548 76.4575 50.4457 79 47.4947 79H31.5053C28.5543 79 26.2452 76.4575 26.5284 73.5201L27.35 65H51.65L52.4716 73.5201Z M33 78C33 75.7909 34.7909 74 37 74H42C44.2091 74 46 75.7909 46 78V79H33V78Z M73.5201 27.5284C76.4575 27.2452 79 29.5543 79 32.5053V48.4947C79 51.4457 76.4575 53.7548 73.5201 53.4716L65 52.65V28.35L73.5201 27.5284Z M78 47C75.7909 47 74 45.2091 74 43V38C74 35.7909 75.7909 34 78 34H79V47H78Z M6.47992 53.4716C3.54248 53.7548 1 51.4457 1 48.4947L1 32.5053C1 29.5543 3.54249 27.2452 6.47992 27.5284L15 28.35L15 52.65L6.47992 53.4716Z M61 15H19C16.7909 15 15 16.7909 15 19V61C15 63.2091 16.7909 65 19 65H61C63.2091 65 65 63.2091 65 61V19C65 16.7909 63.2091 15 61 15Z M2 34C4.20914 34 6 35.7909 6 38L6 43C6 45.2091 4.20914 47 2 47H1L1 34H2Z' }}, { id:'Rectangle Study Table',addInfo:'Rectangle Study Table',dragSize:{width:38,height:22}, shape :{ type: 'Path', data:'M79 1H3C1.89543 1 1 1.89543 1 3V49C1 50.1046 1.89543 51 3 51H79C80.1046 51 81 50.1046 81 49V3C81 1.89543 80.1046 1 79 1Z'}}, . . . . . . . . . . . . ]; var bedRoom = [ { id:'Double bed',addInfo:'Double bed', shape :{ type: 'Path', data:'M1 54V2C1 1.44772 1.44772 1 2 1H44C44.5523 1 45 1.44771 45 2V54C45 54.5523 44.5523 55 44 55H2C1.44772 55 1 54.5523 1 54Z M7.57446 14.4176C8.01615 12.4296 7.72961 10.0298 7.34925 8.31942C7.20247 7.65937 7.68924 7 8.36541 7H19.6515C20.3227 7 20.8097 7.6496 20.6533 8.3023C20.4282 9.24153 20.1765 10.5125 20.1765 11.5C20.1765 12.4875 20.4282 13.7585 20.6533 14.6977C20.8097 15.3504 20.3227 16 19.6515 16H8.68924C7.9291 16 7.4096 15.1596 7.57446 14.4176Z M25.5745 14.4176C26.0161 12.4296 25.7296 10.0298 25.3493 8.31942C25.2025 7.65937 25.6892 7 26.3654 7H37.6515C38.3227 7 38.8097 7.6496 38.6533 8.3023C38.4282 9.24153 38.1765 10.5125 38.1765 11.5C38.1765 12.4875 38.4282 13.7585 38.6533 14.6977C38.8097 15.3504 38.3227 16 37.6515 16H26.6892C25.9291 16 25.4096 15.1596 25.5745 14.4176Z M45 53V27.695C41.8333 26.4298 34.1 24.6584 28.5 27.695C22.9 30.7316 16.5 25.5863 14 22.6341C10.1667 18.2057 2.2 13.0182 1 27.6951V53H45Z M1 54V2C1 1.44772 1.44772 1 2 1H44C44.5523 1 45 1.44771 45 2V54C45 54.5523 44.5523 55 44 55H2C1.44772 55 1 54.5523 1 54Z M7.57446 14.4176C8.01615 12.4296 7.72961 10.0298 7.34925 8.31942C7.20247 7.65937 7.68924 7 8.36541 7H19.6515C20.3227 7 20.8097 7.6496 20.6533 8.3023C20.4282 9.24153 20.1765 10.5125 20.1765 11.5C20.1765 12.4875 20.4282 13.7585 20.6533 14.6977C20.8097 15.3504 20.3227 16 19.6515 16H8.68924C7.9291 16 7.4096 15.1596 7.57446 14.4176Z M25.5745 14.4176C26.0161 12.4296 25.7296 10.0298 25.3493 8.31942C25.2025 7.65937 25.6892 7 26.3654 7H37.6515C38.3227 7 38.8097 7.6496 38.6533 8.3023C38.4282 9.24153 38.1765 10.5125 38.1765 11.5C38.1765 12.4875 38.4282 13.7585 38.6533 14.6977C38.8097 15.3504 38.3227 16 37.6515 16H26.6892C25.9291 16 25.4096 15.1596 25.5745 14.4176Z M25.5745 14.4176C26.0161 12.4296 25.7296 10.0298 25.3493 8.31942C25.2025 7.65937 25.6892 7 26.3654 7H37.6515C38.3227 7 38.8097 7.6496 38.6533 8.3023C38.4282 9.24153 38.1765 10.5125 38.1765 11.5C38.1765 12.4875 38.4282 13.7585 38.6533 14.6977C38.8097 15.3504 38.3227 16 37.6515 16H26.6892C25.9291 16 25.4096 15.1596 25.5745 14.4176Z'}}, . . . . . . . . . . . . ] var livingRoom=[ { id:'TV',addInfo:'TV',dragSize:{width:48,height:20},shape :{ type: 'Path', data:'M55 23H4C2.34315 23 1 24.3431 1 26V40H58V26C58 24.3431 56.6569 23 55 23ZM55 23L48.1968 4.29128C47.4783 2.31534 45.6004 1 43.4979 1H16.5021C14.3996 1 12.5217 2.31533 11.8032 4.29128L5 23H55ZM16 1V23M25 1V23M34 1V23M43 1V23M1 36.5H58'}}, { id:'Flat TV',addInfo:'Flat TV',dragSize:{width:66,height:13}, shape :{ type: 'Path', data:'M1 15H92M1 15V20H92V15M1 15V10H92V15M79 7L85 1M79 20L85 26M14 7L8 1M14 20L8 26M37 2V7M39 2V7M41 2V7M43 2V7M45 2V7M47 2V7M49 2V7M51 2V7M53 2V7M55 2V7M23 2H70L76 7H17L23 2ZM5 7H88V10H5V7Z'}}, { id:'Flat TV1',addInfo:'Flat TV',dragSize:{width:66,height:13}, shape :{ type: 'Path', data:'M1 14H92M1 14V19H92V14M1 14V9H92V14M37 1V6M39 1V6M41 1V6M43 1V6M45 1V6M47 1V6M49 1V6M51 1V6M53 1V6M55 1V6M23 1H70L76 6H17L23 1ZM5 6H88V9H5V6Z'}}, { id:'Elevator',addInfo:'Elevator',dragSize:{width:37,height:37}, shape :{ type: 'Path', data:'M14.3536 14.6464L77.3536 77.6464M77.3536 14.3536L14.3536 77.3536M14 14H78V78H14V14ZM11 11H81V81H11V11ZM9 89H1V1H91V89H83V9H9V89Z'}}, . . . . . . . . . . . . ]; var kitchen=[ { id:'Small Gas Range' ,addInfo:'Small Gas Range',dragSize:{width:70,height:25},shape :{ type: 'Path', data:'M67.5828 1.96454C67.5828 1.43184 67.151 1 66.6183 1L1.96405 1.00001C1.43135 1.00001 0.999512 1.43184 0.999512 1.96454L0.999515 34.0355C0.999515 34.5682 1.43135 35 1.96405 35L66.6183 35C67.151 35 67.5828 34.5682 67.5828 34.0355V1.96454Z M33.5828 4.79852C33.5828 4.26582 33.151 3.83398 32.6183 3.83398L4.79737 3.83399C4.26467 3.83399 3.83283 4.26583 3.83283 4.79853L3.83284 31.2028C3.83284 31.7355 4.26468 32.1673 4.79738 32.1673L32.6183 32.1673C33.151 32.1673 33.5828 31.7355 33.5828 31.2028L33.5828 4.79852Z M64.7488 4.79852C64.7488 4.26582 64.317 3.83398 63.7843 3.83398L35.9634 3.83399C35.4307 3.83399 34.9988 4.26583 34.9988 4.79853L34.9989 31.2028C34.9989 31.7355 35.4307 32.1673 35.9634 32.1673L63.7843 32.1673C64.317 32.1673 64.7489 31.7355 64.7489 31.2028L64.7488 4.79852Z M26.4988 18C26.4988 22.6944 22.6933 26.5 17.9988 26.5C13.3044 26.5 9.49885 22.6944 9.49885 18C9.49885 13.3056 13.3044 9.5 17.9988 9.5C22.6933 9.5 26.4988 13.3056 26.4988 18Z M25.4363 18C25.4363 22.1076 22.1065 25.4375 17.9988 25.4375C13.8912 25.4375 10.5613 22.1076 10.5613 18C10.5613 13.8924 13.8912 10.5625 17.9988 10.5625C22.1065 10.5625 25.4363 13.8924 25.4363 18Z M25.0574 24.6826L24.6818 25.0583L22.0015 22.378L22.3772 22.0024L25.0574 24.6826Z M13.9442 13.5703L13.5685 13.946L10.888 11.2655L11.2637 10.8898L13.9442 13.5703Z M24.786 11.0938L25.1616 11.4694L22.4813 14.1497L22.1057 13.774L24.786 11.0938Z M13.7733 21.9844L14.1489 22.36L11.4686 25.0403L11.093 24.6646L13.7733 21.9844Z M21.452 18C21.452 19.9071 19.906 21.4531 17.9988 21.4531C16.0917 21.4531 14.5457 19.9071 14.5457 18C14.5457 16.0929 16.0917 14.5469 17.9988 14.5469C19.906 14.5469 21.452 16.0929 21.452 18Z M57.6658 18C57.6658 22.6944 53.8603 26.5 49.1658 26.5C44.4714 26.5 40.6658 22.6944 40.6658 18C40.6658 13.3056 44.4714 9.5 49.1658 9.5C53.8603 9.5 57.6658 13.3056 57.6658 18Z M56.6033 18C56.6033 22.1076 53.2735 25.4375 49.1658 25.4375C45.0582 25.4375 41.7283 22.1076 41.7283 18C41.7283 13.8924 45.0582 10.5625 49.1658 10.5625C53.2735 10.5625 56.6033 13.8924 56.6033 18Z M56.2235 24.6826L55.8478 25.0583L53.1675 22.378L53.5432 22.0024L56.2235 24.6826Z M45.1121 13.5703L44.7365 13.946L42.056 11.2655L42.4317 10.8898L45.1121 13.5703Z M55.952 11.0938L56.3276 11.4694L53.6474 14.1497L53.2717 13.774L55.952 11.0938Z M44.9403 21.9844L45.3159 22.36L42.6356 25.0403L42.26 24.6646L44.9403 21.9844Z M52.619 18C52.619 19.9071 51.0729 21.4531 49.1658 21.4531C47.2587 21.4531 45.7127 19.9071 45.7127 18C45.7127 16.0929 47.2587 14.5469 49.1658 14.5469C51.0729 14.5469 52.619 16.0929 52.619 18Z'}}, . . . . . . . . . . . . ] var bathRoom =[ { id:'Toilet1',addInfo:'Toilet',dragSize:{width:30,height:30}, shape :{ type: 'Path', data: 'M46.4065 37.9425C46.4065 55.0951 36.823 69.0002 25.0011 69.0002C13.1792 69.0002 3.5957 55.0951 3.5957 37.9425C3.5957 30.1141 5.2059 23.4623 8.5 18C8.61402 18 25 18 25 18H41.2425C44.5366 23.4623 46.4065 30.1141 46.4065 37.9425Z M41.2161 43.5006C41.2161 53.9728 33.9559 62.4621 24.9999 62.4621C16.0439 62.4621 8.78369 53.9728 8.78369 43.5006C8.78369 33.0284 16.0439 24.5391 24.9999 24.5391C33.9559 24.5391 41.2161 33.0284 41.2161 43.5006Z M1 3.61538C1 2.17095 2.17095 1 3.61538 1H46.3846C47.8291 1 49 2.17095 49 3.61538V15.3846C49 16.8291 47.8291 18 46.3846 18H3.61538C2.17095 18 1 16.8291 1 15.3846V3.61538Z M26.9995 9C26.9995 10.1046 26.1041 11 24.9995 11C23.8949 11 22.9995 10.1046 22.9995 9C22.9995 7.89543 23.8949 7 24.9995 7C26.1041 7 26.9995 7.89543 26.9995 9Z M31 33C31 37.4183 28.3137 41 25 41C21.6863 41 19 37.4183 19 33C19 28.5817 21.6863 25 25 25C28.3137 25 31 28.5817 31 33Z '}}, { id:'Toilet2',addInfo:'Toilet',dragSize:{width:30,height:30}, shape :{ type: 'Path', data:'M46.989 33.0731C46.989 53.4671 37.3818 69.9996 25.5307 69.9996C13.6795 69.9996 4.07227 53.4671 4.07227 33.0731C4.07227 19.1497 4 18 4 18C4.17549 18.0144 15.9306 18.2553 26.5002 17.9993C36.1582 17.7654 46.989 17.9999 46.989 17.9999C46.989 17.9999 46.989 21.6119 46.989 33.0731Z M41 44.5C41 54.7173 34.0604 63 25.5 63C16.9396 63 10 54.7173 10 44.5C10 34.2827 16.9396 26 25.5 26C34.0604 26 41 34.2827 41 44.5Z M1 3.61538C1 2.17095 2.17095 1 3.61538 1H46.3846C47.8291 1 49 2.17095 49 3.61538V15.3846C49 16.8291 47.8291 18 46.3846 18H3.61538C2.17095 18 1 16.8291 1 15.3846V3.61538Z M26.9995 9C26.9995 10.1046 26.1041 11 24.9995 11C23.8949 11 22.9995 10.1046 22.9995 9C22.9995 7.89543 23.8949 7 24.9995 7C26.1041 7 26.9995 7.89543 26.9995 9Z M31 34C31 38.4183 28.3137 42 25 42C21.6863 42 19 38.4183 19 34C19 29.5817 21.6863 26 25 26C28.3137 26 31 29.5817 31 34Z'}}, . . . . . . . . . . . . ]
The following image shows the collection of floor planner shapes in the symbol palette.
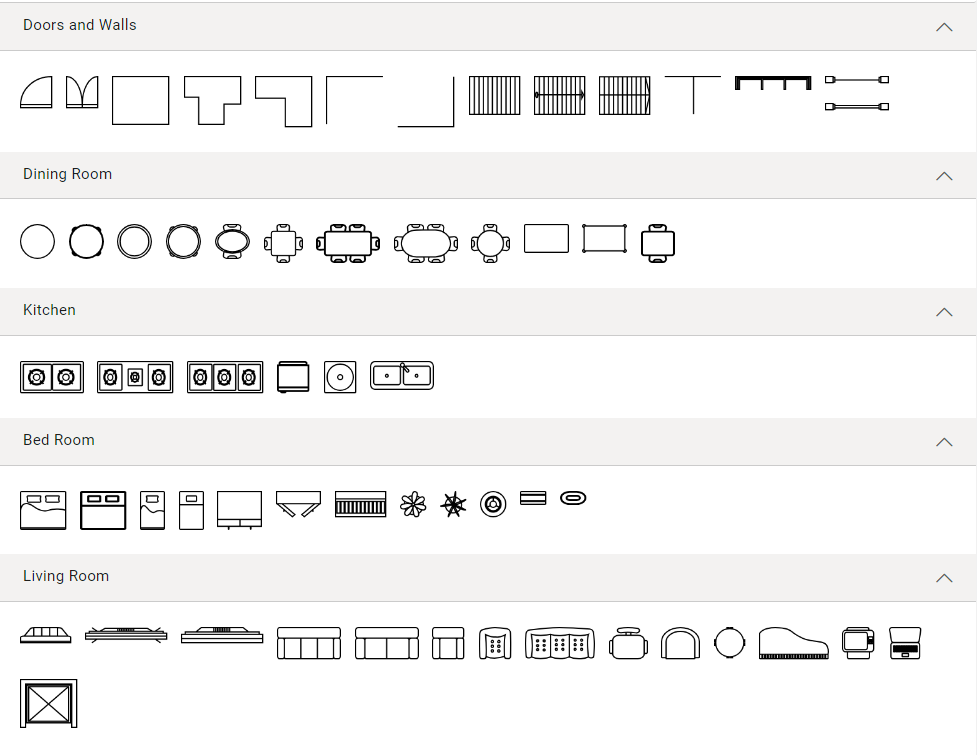
Note: Refer to the symbol palette in JavaScript Diagram library documentation for more details on adding, grouping, and customizing the symbol palette appearance.
Create floor planner design using JavaScript Diagram library
You can now design your floor planner diagram by adding it to the diagram surface.
Step 1: Add floor planner symbols to the editor
We can create the floor planner design by dragging the symbols from the symbol palette and dropping them onto the diagram surface at the desired location.
Refer to the following image.
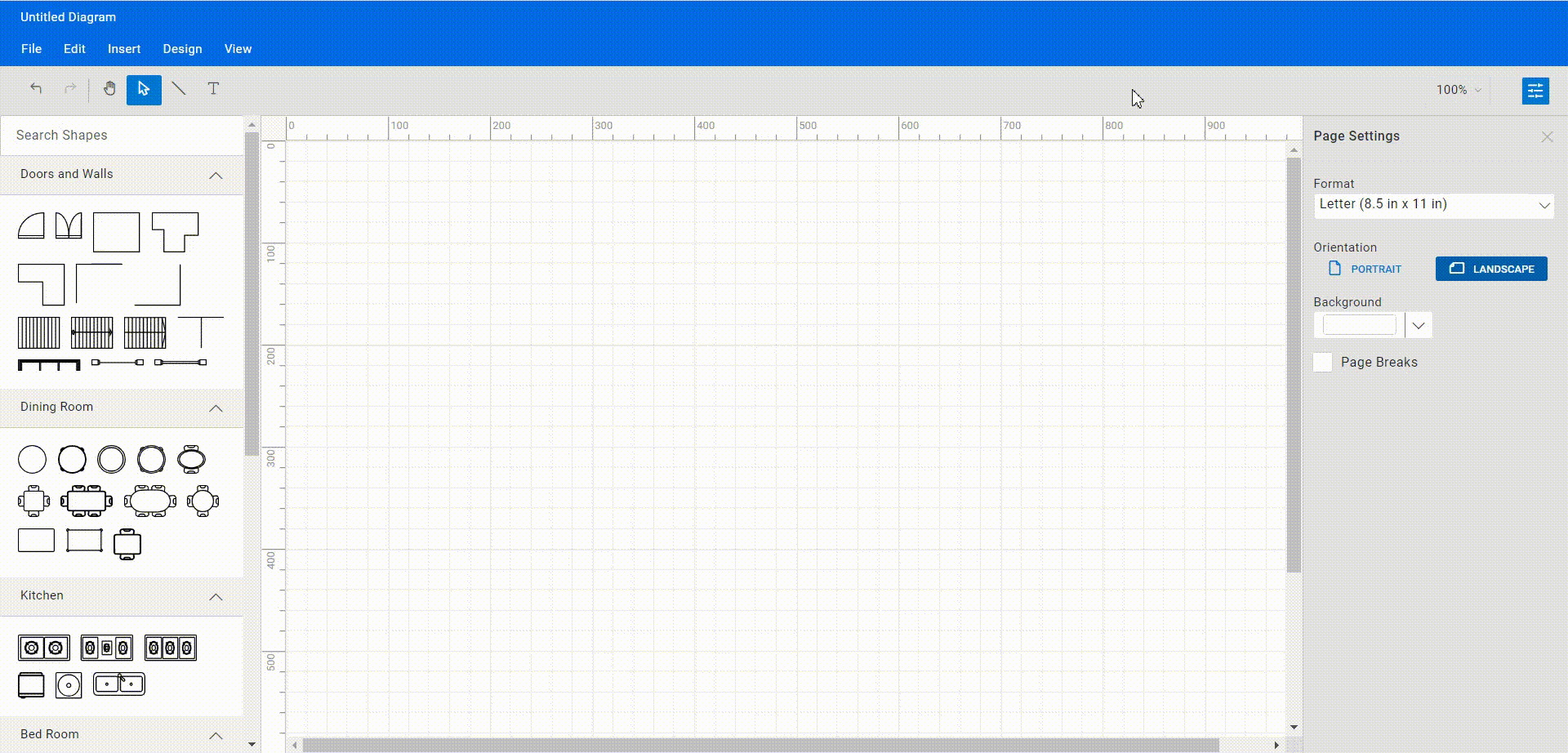
Step 2: Moving symbols
Now, you can change the position of the dropped symbols by simply clicking and dragging them to the desired location on the diagram surface.
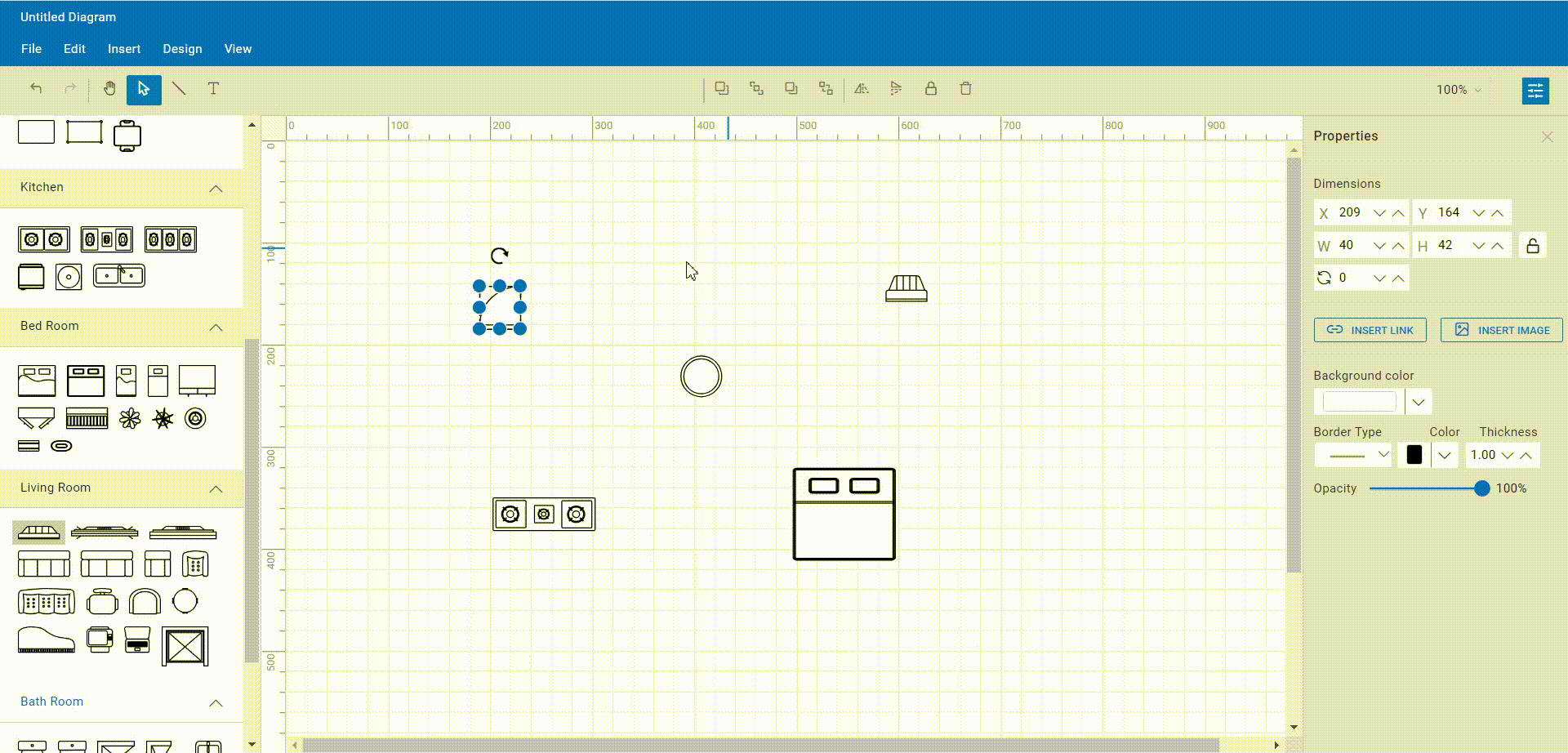
Step 3: Loading and saving the floor planner diagram
We can also save our work and resume it later by loading the saved diagram back onto the diagram canvas.
To save a diagram, go to the File menu and select Save. This will save the diagram as a file on the local drive.
To load an existing diagram file, navigate to the File -> Open menu. This will open the file dialog box. From there, we can browse and select the saved diagram file we want to load.
This feature provides great flexibility and convenience, allowing us to easily pick up where we left off on a diagram or to make changes to a previously saved diagram. It’s an essential feature for any diagramming app that enables users to create and manage complex diagrams.
Refer to the following GIF image.
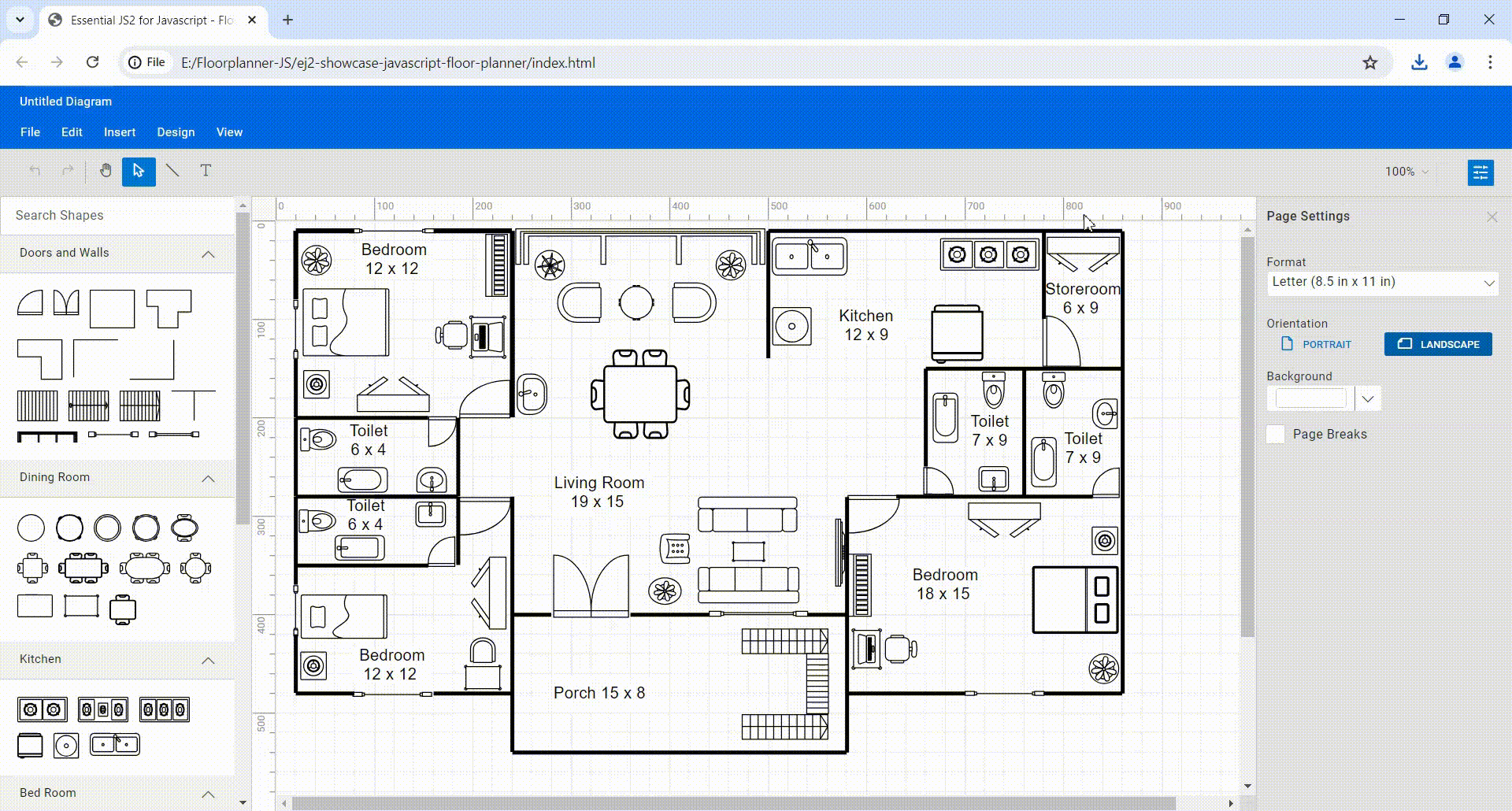
Note: For more details, refer to loading and saving diagrams in the Javascript Diagram library documentation.
Step 4: Undo or redo the diagram action
The Javascript Diagram library supports undo and redo operations to track the changes made to a diagram. You can use these features to delete a mistake quickly or restore an action you previously did.
To undo an action, use the Ctrl + Z keyboard shortcut, click the Undo button on the toolbar, or use the Undo commands from the Edit menu. This will undo the most recent action that was performed.
To redo an action, use the Ctrl + Y keyboard shortcut, click the Redo button on the toolbar, or use the Redo commands from the Edit menu. This will redo the most recently undone action.
Refer to the following image.
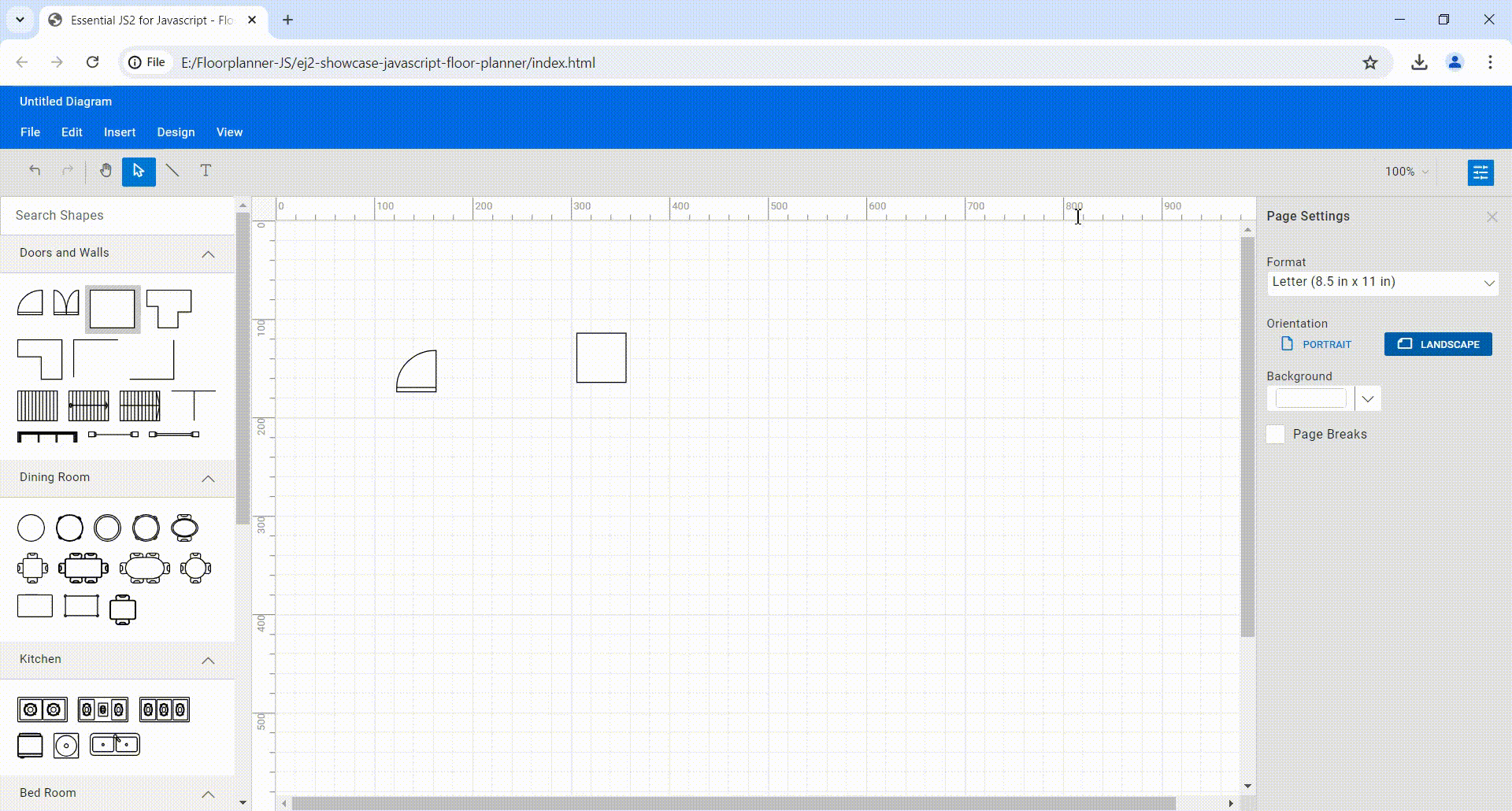
Step 5: Export diagram
You can export the created floor planner diagram as an image file in different formats such as JPEG, PNG, and SVG. By exporting the diagram as an image file, you can easily share it via email or other digital means or embed it in a document or presentation.
To do this, click the File menu and select Export. A dialog box will appear where you can specify the name and image file format to save the floor planner diagram. You can also export only the content area of the diagram by excluding the blank areas or export the entire diagram (including blank areas) based on the width and height specified in the page settings.
Step 6: Print the diagram
To print a diagram, click the File menu and select Print. This will open the Print dialog box, where you can select your printer and customize the print settings, such as orientation, paper size, and page margins. Then, click on the Print button to print the floor planner diagram.
Refer to the following image.
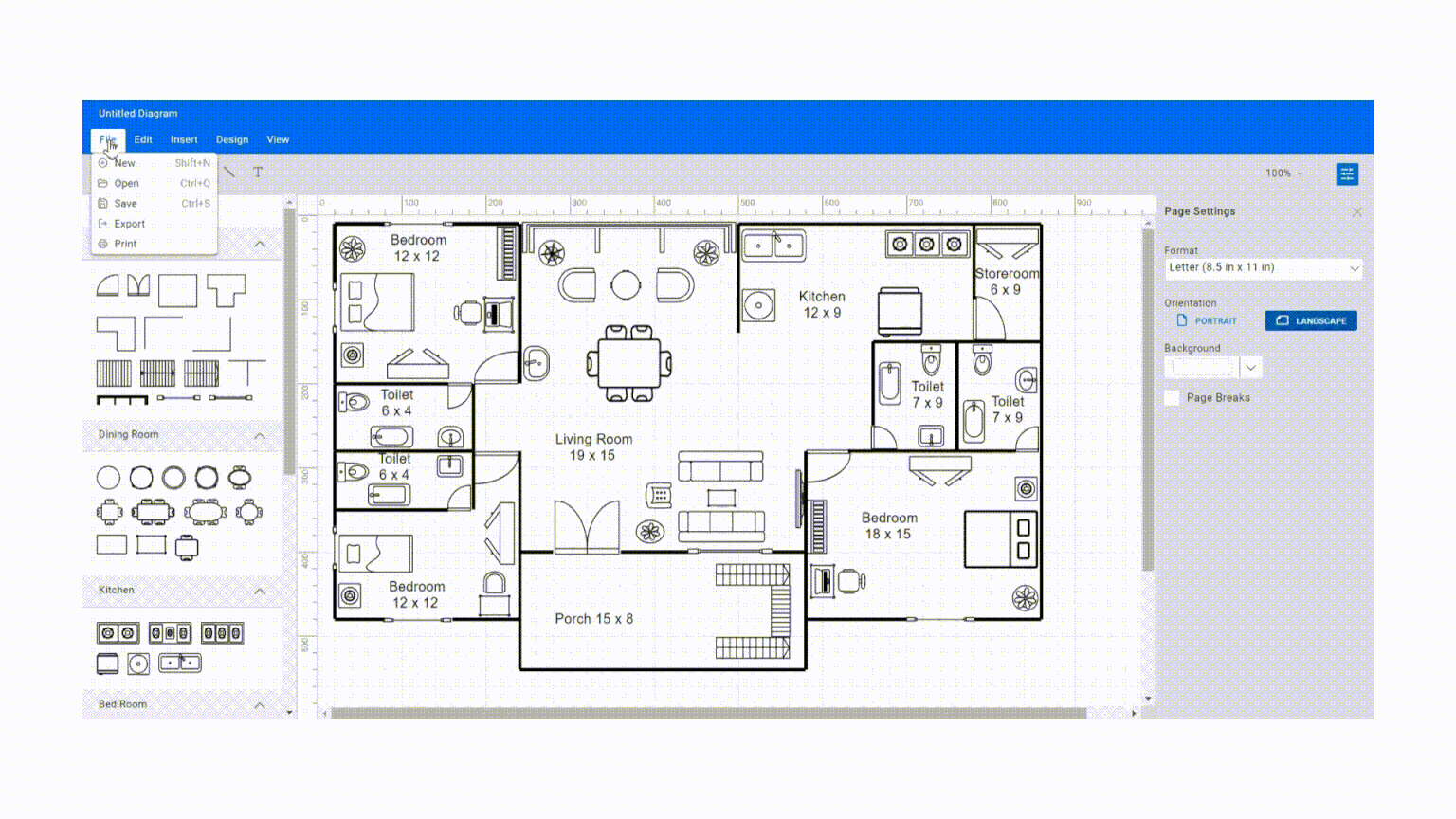
Step 7: Pan and zoom
The Javascript Diagram library supports the following panning and zooming options:
- Pan using the scrollbars: The most straightforward way to pan a diagram is by using the scrollbars on the right and bottom sides. You can use these to scroll through the diagram in the desired direction.
- Pan using the mouse wheel: You can also pan a diagram by using the mouse wheel. To scroll up or down, rotate the mouse wheel forward or backward; to scroll left or right, hold Shift while rotating the scroll wheel forward or backward.
- Pan using the pan tool: You can also pan a diagram by selecting the Pantool from the toolbar. Then, hold down the left mouse button and drag the mouse to move the diagram in any direction.
- Zoom using keyboard shortcuts: The most efficient way to zoom in and out of the diagram is to use the Ctrl + mouse wheel shortcut.
- Zoom using the toolbar option: You can also zoom in or out in a diagram using the zoom dropdown in the upper-right corner of the app window. In the Zoom dropdown, you can select the Zoom To Fit option to fit the entire floor planner diagram to the window.
GitHub reference
For more details, refer to creating a floor planner diagram using the JavaScript Diagram library GitHub demo.

Easily build real-time apps with Syncfusion’s high-performance, lightweight, modular, and responsive JavaScript UI components.
Conclusion
Thanks for reading! In this blog, we’ve seen how to create a floor planner diagram using the Syncfusion JavaScript Diagram library. You can also use the Diagram library to create organizational charts, flowcharts, mind maps, floor plans, network diagrams, or logic circuit diagrams. Try it out and leave your feedback in the comments section below!
If you’re an existing Syncfusion user, the newest version of Essential Studio® is available from the License and Downloads page. If you’re new to Syncfusion, you can take advantage of our 30-day free trial to explore the features and capabilities of our components.
You can also reach us through our support forum, support portal, or feedback portal. We are always happy to assist you!
Related blogs
- Top 5 Techniques to Protect Web Apps from Unauthorized JavaScript Execution
- Easily Render Flat JSON Data in JavaScript File Manager
- Effortlessly Synchronize JavaScript Controls Using DataManager
- Optimizing Productivity: Integrate Salesforce with JavaScript Scheduler