TL;DR: A content security policy (CSP) is a set of rules you can define for your browser that dictate which resources from which domains are trusted. A CSP gives you control over what your page loads and improves app security by helping prevent cross-site scripting (XSS) attacks. Implementing and debugging CSPs can be challenging, but the security is worth the trouble.
Today’s web applications are more complicated than four to five years ago. They heavily depend on third-party services to deliver complex user requirements. While such libraries ease the work of developers in implementing complicated features, their use also exposes apps to attack.
In this article, I will discuss how we can protect our web apps against the security threats introduced by third-party libraries using content security policies.
What is a content security policy (CSP)?
A content security policy (CSP) is a set of rules you can define for your browser that dictate which resources from which domains are trusted. The browser follows this set of defined rules while loading your page, determining what can come through and what cannot.
A CSP ensures overall website protection by allowing only specific types of elements to load or execute within your webpages.
Here are a couple of simple examples of a CSP rule:
- Default Policy (default-src ‘self’;): Only load content from the same origin as the webpage.
- Script Policy (script-src ‘self’ https://writergate.com;): Only allow scripts from the same origin and from https://writergate.com.
Why a CSP is important?
Protection against cross-site scripting (XSS) attacks
A CSP helps you prevent cross-site scripting (XSS) attacks by allowing only the scripts from trusted resources to execute. Here’s an example of a basic configuration of this CSP policy content in the Apache server.
<IfModule mod_headers.c>
Header set Content-Security-Policy "default-src 'self'; script-src 'self' https://writergate.com; style-src 'self'; img-src 'self'; font-src 'self';"
</IfModule>
Prevention of data theft
Attackers can steal user information like login credentials and personal data by inserting illegal code into a program. The adoption of a CSP would reduce the chances of unauthorized access as well as data stealing by specifying trusted sources for scripts, styles, and other resources.
Control over what content can be loaded on your site
A CSP gives you the ability to determine what your web pages will load. You can decide which external resources are permitted (e.g., scripts, images, and styles). This means only safe content meant for it is loaded on the site, preventing untrusted or malicious sources from introducing potential security threats.
Following is an example of how this CSP policy content could be configured in the Apache server in its simplest form.
<IfModule mod_headers.c>
Header set Content-Security-Policy "default-src 'self'; script-src 'self' https://writergate.com; style-src 'self' https://writergate.com; img-src 'self' https://writergate.com; font-src 'self' https://writergate.com;"
</IfModule>
Benefits of using a CSP
- High security: Improves app security, since it verifies the source of the script before executing it.
- Reduced risk of data breaches: Preventing malicious code from stealing sensitive information, a CSP decreases unauthorized data access.
- Improved user trust and confidence: A secure web app with a CSP fosters user trust, making users feel safer while using the site and sharing their information.
- Better control over third-party content: Permitting developers to stipulate secure third-party sources reduces the risk of introducing vulnerabilities via untrusted content.
- Simplified security management: A CSP makes security management easier by providing clear rules on content sources and making it simpler to maintain and update security measures.
How to implement a CSP?
Creating a content security policy (CSP) is easy and only takes a few steps. Here’s how you can create and implement a basic CSP:
Step 1: Identify content sources
Determine which sources you want to allow for scripts, styles, images, and other resources. This includes your domain and any trusted third-party services:
- First-party content: Your domain.
- Third-party content: External services for scripts, styles, fonts, images, etc.
Step 2: Define your CSP policy
Write a CSP policy that specifies allowed sources using directives like:
- default-src: Fallback for other directives if not specified (default-src ‘self’;).
- script-src: Specifies allowed sources for JavaScript (script-src ‘self’ https://writergate.com;).
- style-src: Specifies allowed sources for CSS (style-src ‘self’ https://writergate.com;).
- img-src: Specifies allowed sources for images (img-src ‘self’ https://writergate.com;).
Example CSP policy:
Content-Security-Policy: default-src 'self'; script-src 'self' https://writergate.com; style-src 'self' https://writergate.com;
Step 3: Add CSP header to your web server
Configure your web server to include the CSP header. This can be done through the server configuration file or web framework settings. Examples of popular web servers:
- Apache
<IfModule mod_headers.c> Header set Content-Security-Policy "default-src 'self'; script-src 'self' https://writergate.com; style-src 'self' https://writergate.com;" </IfModule>
- Nginx:
add_header Content-Security-Policy "default-src 'self'; script-src 'self' https://writergate.com; style-src 'self' https://writergate.com;";
- Express.js:
app.use((req, res, next) => { res.setHeader("Content-Security-Policy", "default-src 'self'; script-src 'self' https://writergate.com; style-src 'self' https://writergate.com;"); next(); });
Step 4: Test your CSP
Use browser developer tools to test your CSP policy. Check the console for CSP violations and ensure that legitimate content is not being blocked:
- Google Chrome: Open DevTools -> Console to see CSP violation reports.
- Mozilla Firefox: Open DevTools -> Console for CSP violation logs.
Step 5: Monitor and refine your policy
Continuously monitor CSP reports to identify and address any blocked content that should be allowed. Use the report-uri directive to collect CSP violation reports for analysis.
Content-Security-Policy: default-src 'self'; script-src 'self' https://writergate.com; style-src 'self' https://writergate.com; report-uri /csp-violation-report-endpoint;
Although this process looks a bit complex, you can simplify it by using specialized tools like CSP Evaluator or Report URI’s CSP Generator to generate CSPs automatically.
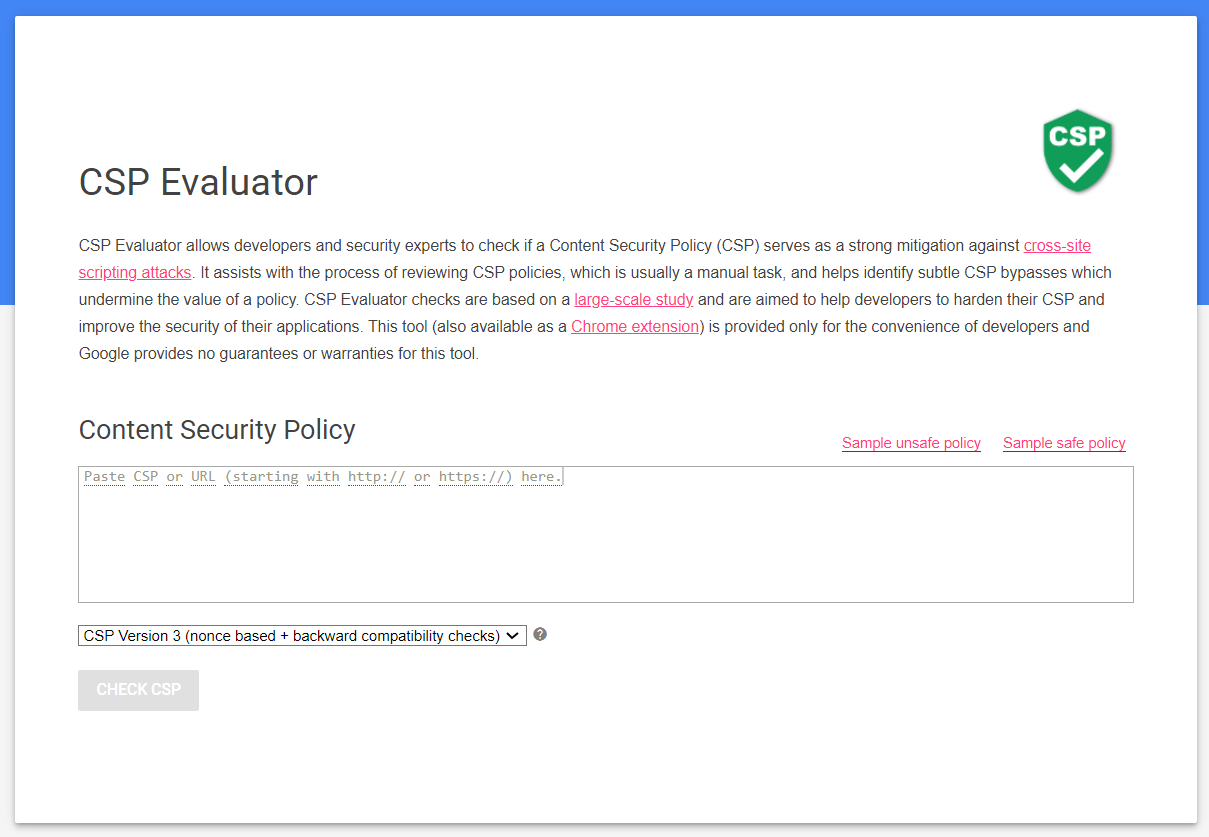
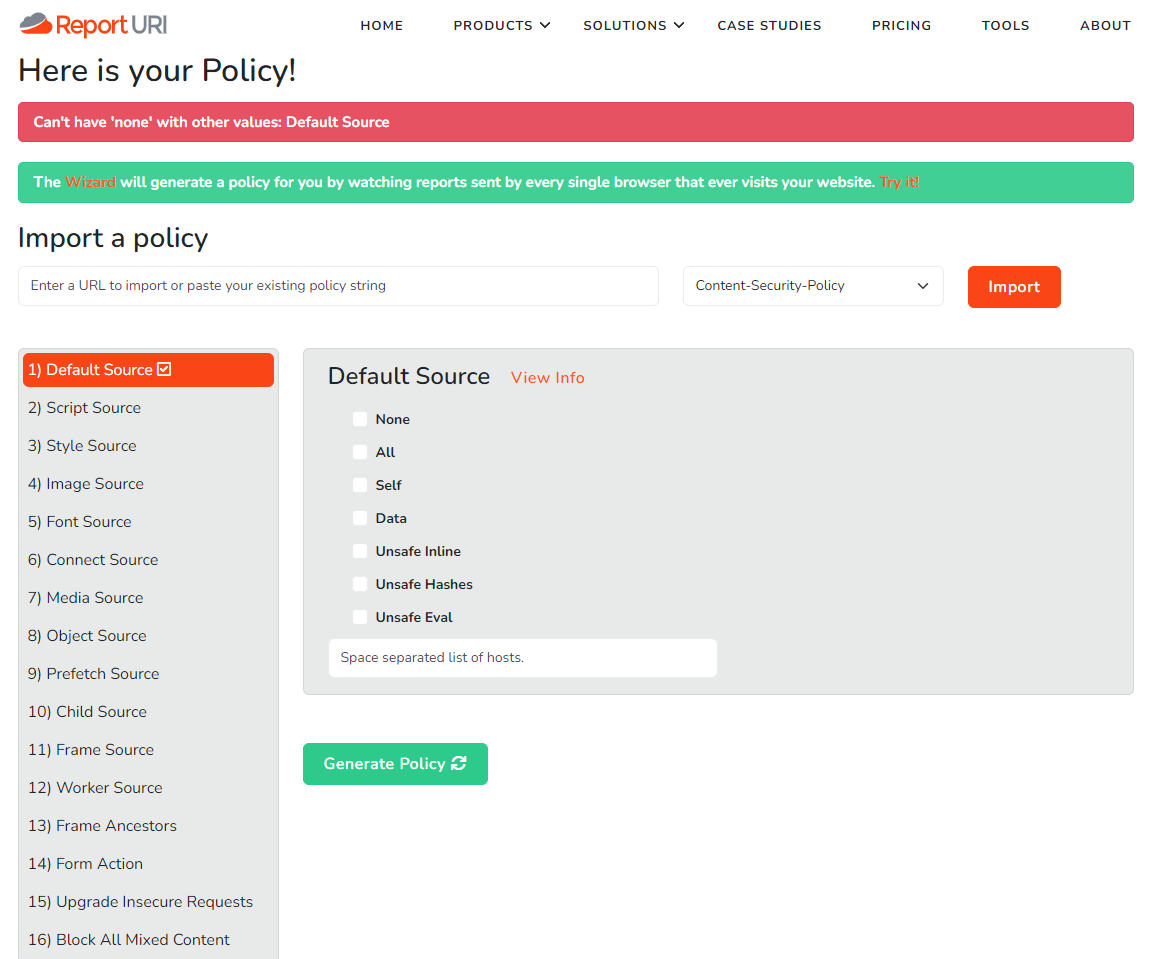
Common challenges and how to overcome them
Implementing a CSP can present several challenges. Following is how to address some common issues.
Balancing security with usability
Challenge:
Balancing security and user experience is a tough nut to crack. If the policy is too restrictive, it might block real data, which renders your web app useless.
Solution:
- At first, deploy your CSP in report-only mode to see what content gets blocked without affecting the users’ experience.
- Introduce stricter policies gradually over time. Start with a permissive policy, then tighten it up on feedback from report-only mode responses.
- Use nonces (numbers used once) and hashes for inline scripts and styles. This way, you can allow specified scripts to run while others are blocked.
// Using nonce <script nonce="random123"> ... </script> // Using hash <script src="..." integrity="sha256-abc123..."> ... </script>
Handling third-party scripts and content
Challenge:
Many web apps use third-party scripts to implement custom requirements. These scripts may increase security vulnerabilities and make the CSP implementation more complex.
Solution:
- Set up your CSP policy in such a manner that it only allows trusted third-party resources.
Content-Security-Policy: script-src 'self' https://writergate.com;
- Subresource integrity (SRI) is a great way to confirm the authenticity of third-party resources. Using SRI, you can specify a cryptographic hash the resource must match.
<script src="https://writergate.com/script.js" integrity="sha384-abc123..."></script>
- Create fallback mechanisms for important features so that even if third-party scripts are not permitted, or they fail to load, your app can still function well with its core functionality in place.
Debugging issues with CSPs
Challenge:
Debugging a content security policy can be challenging because of the difficulty in identifying and rectifying blocked content or violations while not sacrificing the security of your app.
Solutions:
- Modern browsers now include developer tools that can help to debug CSP issues. In the console, you may see CSP violations that will assist you in determining what content is being blocked and why.
- Make sure your CSP contains a report-to directive. This directive allows you to receive violation reports and makes it easy to track down and resolve blocked content.
Content-Security-Policy: default-src 'self'; script-src 'self' https://writergate.com; report-uri /csp-violation-report-endpoint;
Conclusion
Implementing content security policies (CSPs) to guard your apps against cyber threats like data breaches and cross-site scripting (XSS) is important. Although implementing and debugging CSPs can be challenging, they are important to modern web apps because of their security advantages. So, use a CSP for your next project to improve its security against modern vulnerabilities.
If you have any questions, you can contact us through our support forums, support portal, or feedback portal. We are always happy to assist you!
Related blogs
- Log4j Vulnerability- A Giant Security Threat
- Top 5 Best Practices for Angular App Security
- Why Security Matters to Syncfusion and its Customers
- Shield Your ASP.NET MVC Web Apps with Content Security Policy (CSP)