TL;DR: Learn six effective ways to merge PDFs in C# using the Syncfusion PDF Library. Streamline your document workflow, batch process files, and combine multiple PDFs seamlessly.
In today’s digital age, PDFs have become the go-to format for sharing, archiving, and managing documents. Whether you’re handling business reports, legal paperwork, or personal files, merging multiple PDFs into one cohesive file is often essential. Doing so simplifies organization, enhances consistency, and makes it easier to share documents professionally.
With the Syncfusion PDF Library, merging PDF files is straightforward and can be automated for batch processes, saving time and effort.
This blog will cover the following six efficient methods to merge PDF files:
- Combine multiple PDF documents into a single file.
- Merge selected pages ranges from different PDF documents.
- Optimize resources during the PDF merging process.
- Extend the page margins while merging PDF documents.
- Preserve accessibility tags when merging PDF files.
- Add bookmarks to the merged PDF documents for easier navigation.
Let’s get started!

Say goodbye to tedious PDF tasks and hello to effortless document processing with Syncfusion's PDF Library.
Getting started with app creation
- First, create a .NET console app using Visual Studio.
- Then, open Visual Studio and navigate to Tools -> NuGet Package Manager -> Package Manager Console.
- Run the following command in the Package Manager Console to install the Syncfusion.Pdf.Net.Core NuGet package.
Install-Package Syncfusion.Pdf.Net.Core
Combine multiple PDF documents into a single file
Combining multiple PDF documents into a single file is a common task, especially when consolidating reports, forms, or other documents into one unified PDF. This process can be accomplished using the Syncfusion PDF Library with just a few lines of code.
In this example, we’ll merge the following two PDF files, file1.pdf, and file2.pdf, located in the project’s data folder.
file1.pdf
Steps to merge two PDF files
- Initialize a new instance of the PdfDocument class. This object will hold the merged PDF.
- Create two FileStream objects, each representing one of the input PDF files. These streams will allow you to read the contents of the PDF files.
- Call the PdfDocumentBase.Merge() method, passing the PdfDocument object and the array of FileStream objects. This will combine the contents of the two input PDF files into the PdfDocument object.
- Save the merged PdfDocument to a MemoryStream or FileStream object. In this step, the combined PDF is written into a file.
Refer to the following code example.
using Syncfusion.Pdf;
//Create a PDF document.
using (PdfDocument finalDocument = new PdfDocument())
{
//Get the stream from an existing PDF document.
using (FileStream firstStream = new FileStream("data/file1.pdf", FileMode.Open, FileAccess.Read))
using (FileStream secondStream = new FileStream("data/file2.pdf", FileMode.Open, FileAccess.Read))
{
//Create a stream array for merging.
Stream[] streams = { firstStream, secondStream };
//Merge PDF documents.
PdfDocumentBase.Merge(finalDocument, streams);
//Save the document.
using (FileStream outputStream = new FileStream("combine-multiple-pdf-files.pdf", FileMode.Create, FileAccess.ReadWrite))
{
finalDocument.Save(outputStream);
}
}
}
When you execute the above code example, you’ll obtain a single merged PDF document as the output.
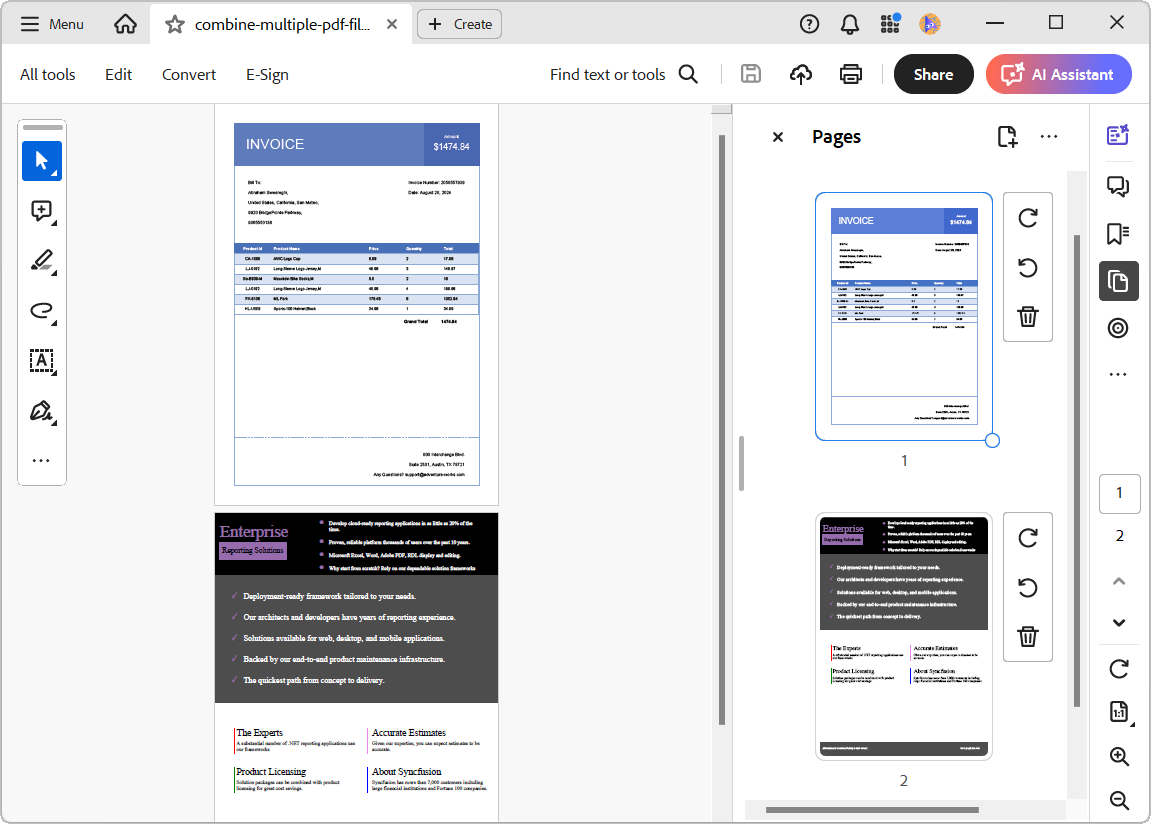
Merge the selected page ranges from different PDF documents
Merging selected page ranges from different PDF documents involves combining specific pages from multiple PDFs into one file. You can easily achieve this using the Syncfusion PDF library by loading each PDF, selecting the desired page ranges, and appending these pages to a new document. This allows precise control over the pages that need to be included in the final merged PDF.
In this example, we’ll take pages 2 to 5 from file1.pdf and pages 1 to 2 from file2.pdf to merge these specific pages into a single PDF file.
file1.pdf
Steps to merge selected PDF pages range from different PDF files
- First, initialize a new PdfDocument instance to store the final merged PDF file.
- Next, create two FileStream objects to represent the input PDF files. These streams will allow you to access and read the contents of the PDFs.
- Load the first input PDF file (file1.pdf) using a PdfLoadedDocument object, and then import the specified pages by calling the ImportPageRange method.
- Repeat the process for the second PDF file (file2.pdf), importing the desired pages.
- Finally, save the combined PDF document to a FileStream or MemoryStream. Refer to the following code example.
// Create a new PDF document.
using PdfDocument finalDocument = new PdfDocument();
// Open and load the first PDF file.
using FileStream firstStream = new FileStream("data/file1.pdf", FileMode.Open, FileAccess.Read);
PdfLoadedDocument loadedDocument1 = new PdfLoadedDocument(firstStream);
// Import pages 2 to 5 from the first PDF document.
finalDocument.ImportPageRange(loadedDocument1, 1, 5);
// Open and load the second PDF file.
using FileStream secondStream = new FileStream("data/file2.pdf", FileMode.Open, FileAccess.Read);
PdfLoadedDocument loadedDocument2 = new PdfLoadedDocument(secondStream);
// Import pages 1 to 2 from the second PDF document.
finalDocument.ImportPageRange(loadedDocument2, 0, 1);
// Save the final document.
using FileStream outputStream = new FileStream("merge-selected-page-ranges.pdf", FileMode.Create, FileAccess.ReadWrite);
finalDocument.Save(outputStream);
After running the program, you will get a PDF file containing the merged page ranges from both input documents.
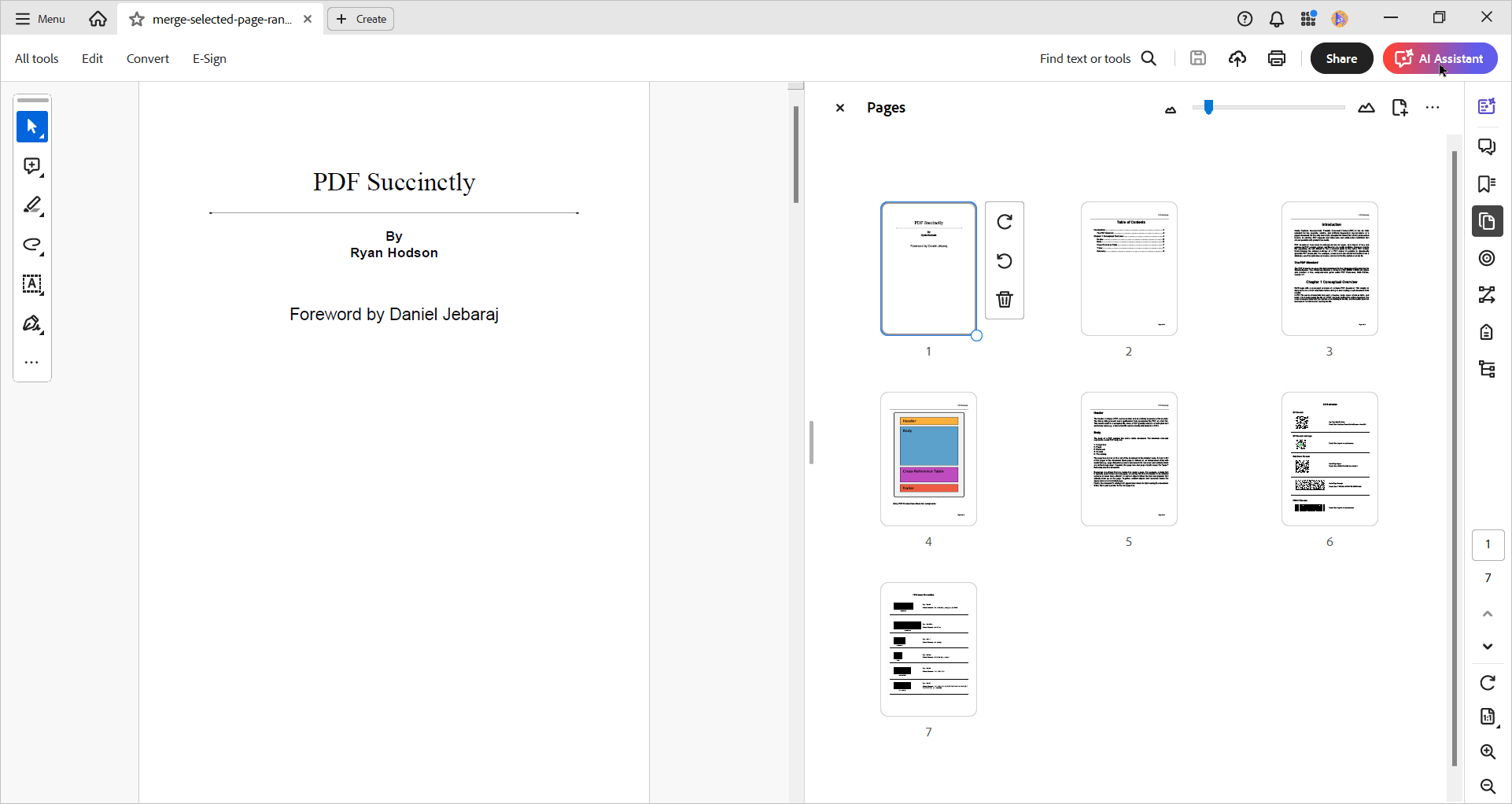

Unleash the full potential of Syncfusion's PDF Library! Explore our advanced resources and empower your apps with cutting-edge functionalities.
Optimize resources during the PDF merging process
Optimizing resources during the PDF merging process involves reducing file size and memory usage while maintaining the quality of the merged document. With the Syncfusion PDF Library, you can streamline the merging process by efficiently managing resources such as images, fonts, and other assets, ensuring that the resulting PDF is compact and optimized for performance.
The OptimizeResources property in PdfMergeOptions is used to optimize PDF page resources during the merging process.
Steps to optimize resources during the PDF merging process
- Start by creating a new instance of the PdfDocument class, which will hold the merged and optimized PDF.
- Create FileStream objects for the input PDF files to allow reading their contents during the merge process.
- To optimize resources like images and fonts during the merging process, use the OptimizeResources property available in the PdfMergeOptions class. This helps reduce file size and memory usage while preserving document quality.
- After merging, save the optimized PDF document to a FileStream or MemoryStream. Refer to the following code example.
// Create a new PDF document.
using (PdfDocument outputDocument = new PdfDocument())
{
// Load the first PDF document.
using (FileStream firstPDFStream = new FileStream("data/file1.pdf", FileMode.Open, FileAccess.Read))
{
// Load the second PDF document
using (FileStream secondPDFStream = new FileStream("data/file2.pdf", FileMode.Open, FileAccess.Read))
{
// Create a list of streams to merge.
Stream[] streams = { firstPDFStream, secondPDFStream };
// Create a merge options object.
PdfMergeOptions mergeOptions = new PdfMergeOptions();
// Enable the optimize resources option.
mergeOptions.OptimizeResources = true;
// Merge the PDF documents with optimization.
PdfDocumentBase.Merge(outputDocument, mergeOptions, streams);
// Save the document.
using (FileStream outputMemoryStream = new FileStream("optimize-resources-merge-pdf.pdf", FileMode.Create, FileAccess.ReadWrite))
{
outputDocument.Save(outputMemoryStream);
}
}
}
}
After running the code, you will receive a merged PDF file optimized for size, ensuring efficient resource usage.

Note: The optimization process is most effective when the PDF documents being merged contain identical resources, such as fonts, images, and other embedded elements. The optimization may not significantly reduce the file size if the resources differ between the PDFs.
Extend the page margins while merging PDF documents
Extending the page margins while merging PDF documents allows you to add additional space around the content of each page in the final merged document. Using the Syncfusion PDF Library, you can adjust the page layout during the merging process, ensuring that the merged PDF has consistent and expanded margins as needed.
Steps to extend page margins during PDF merging
- First, initialize a new PdfDocument object and set the page margins to your desired size. In this example, we set the margins to 50 points on all sides.
- Create FileStream objects for the input PDF files to allow access to their contents for merging.
- To extend the margins during the merging process, allow the ExtendMargin option in the PdfMergeOptions object. This ensures the final merged document applies the specified margin settings to all pages.
- Use the PdfDocumentBase.Merge method to merge the input PDF documents, applying the margin settings from the PdfMergeOptions.
- Finally, save the merged document with extended margins to a FileStream or MemoryStream. Refer to the following code example.
// Create a new PDF document with 50-point margins.
using PdfDocument outputDocument = new PdfDocument
{
PageSettings = { Margins = { All = 50 } }
};
// Load the first and second PDF documents.
using FileStream firstPDFStream = new FileStream("data/file1.pdf", FileMode.Open, FileAccess.Read);
using FileStream secondPDFStream = new FileStream("data/file2.pdf", FileMode.Open, FileAccess.Read);
// Create a merge options object and enable the margin extension.
PdfMergeOptions mergeOptions = new PdfMergeOptions
{
ExtendMargin = true
};
// Merge the PDF documents with extended margins.
PdfDocumentBase.Merge(outputDocument, mergeOptions, new[] { firstPDFStream, secondPDFStream });
// Save the document with extended margins.
using FileStream outputMemoryStream = new FileStream("extend-margins-while-merging-pdfs.pdf", FileMode.Create, FileAccess.ReadWrite);
outputDocument.Save(outputMemoryStream);
After running the program, you will obtain a merged PDF with extended margins on each page, providing consistent extra space around the content.
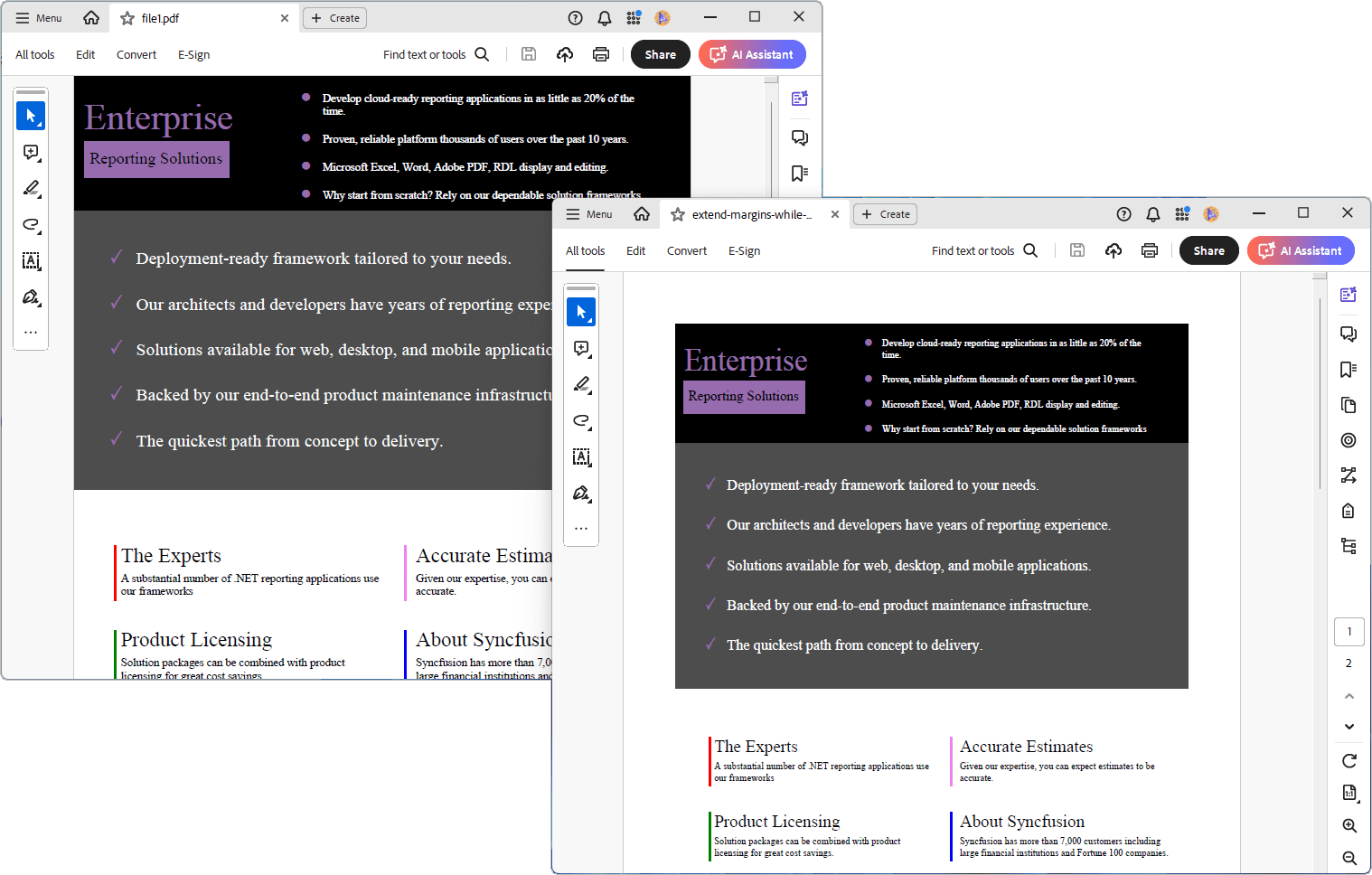

Embark on a virtual tour of Syncfusion's PDF Library through interactive demos.
Preserve accessibility tags when merging PDF documents
Preserving accessibility tags when merging PDF files ensures that the merged document retains important metadata and structure, such as headings, reading order, and alternative text for images. These tags are crucial for making PDFs accessible to users with disabilities, enabling screen readers and other assistive technologies to interpret the content correctly.
Using the Syncfusion PDF Library, you can merge PDF documents while maintaining these accessibility features, ensuring that the resulting file remains fully accessible.
Steps to preserve accessibility tags during PDF merging
- First, create a new PdfDocument instance to hold the merged and accessible PDF document.
- Set up FileStream objects to load the PDF files you want to merge, ensuring these files contain accessibility tags.
- Then, create a PdfMergeOptions object and enable the MergeAccessibilityTags property to ensure that accessibility tags (like reading order, headings, alt-text, etc.) are maintained during the merge process.
- Merge the loaded PDF documents using the PdfDocumentBase.Merge method while applying the merge options for accessibility.
- Finally, save the merged PDF to a FileStream to ensure the document is stored with all the accessibility features intact. Refer to the following code example.
// Create a new PDF document.
using (PdfDocument finalDoc = new PdfDocument())
{
// Load the first and second PDF documents.
using (FileStream stream1 = new FileStream("data/file1.pdf", FileMode.Open, FileAccess.Read))
using (FileStream stream2 = new FileStream("data/file2.pdf", FileMode.Open, FileAccess.Read))
{
// Create merge options and enable accessibility tag merging.
PdfMergeOptions mergeOptions = new PdfMergeOptions
{
MergeAccessibilityTags = true
};
// Merge PDF documents with accessibility tags preserved.
PdfDocumentBase.Merge(finalDoc, mergeOptions, new[] { stream1, stream2 });
// Save the merged document with preserved accessibility tags.
using (FileStream outputFileStream = new FileStream("merge-tags.pdf", FileMode.Create, FileAccess.ReadWrite))
{
finalDoc.Save(outputFileStream);
}
}
}
After running this program, the resulting merged PDF (merge-tags.pdf) will retain all accessibility features from the original files, ensuring that the document is fully accessible and can be navigated by assistive technologies such as screen readers.
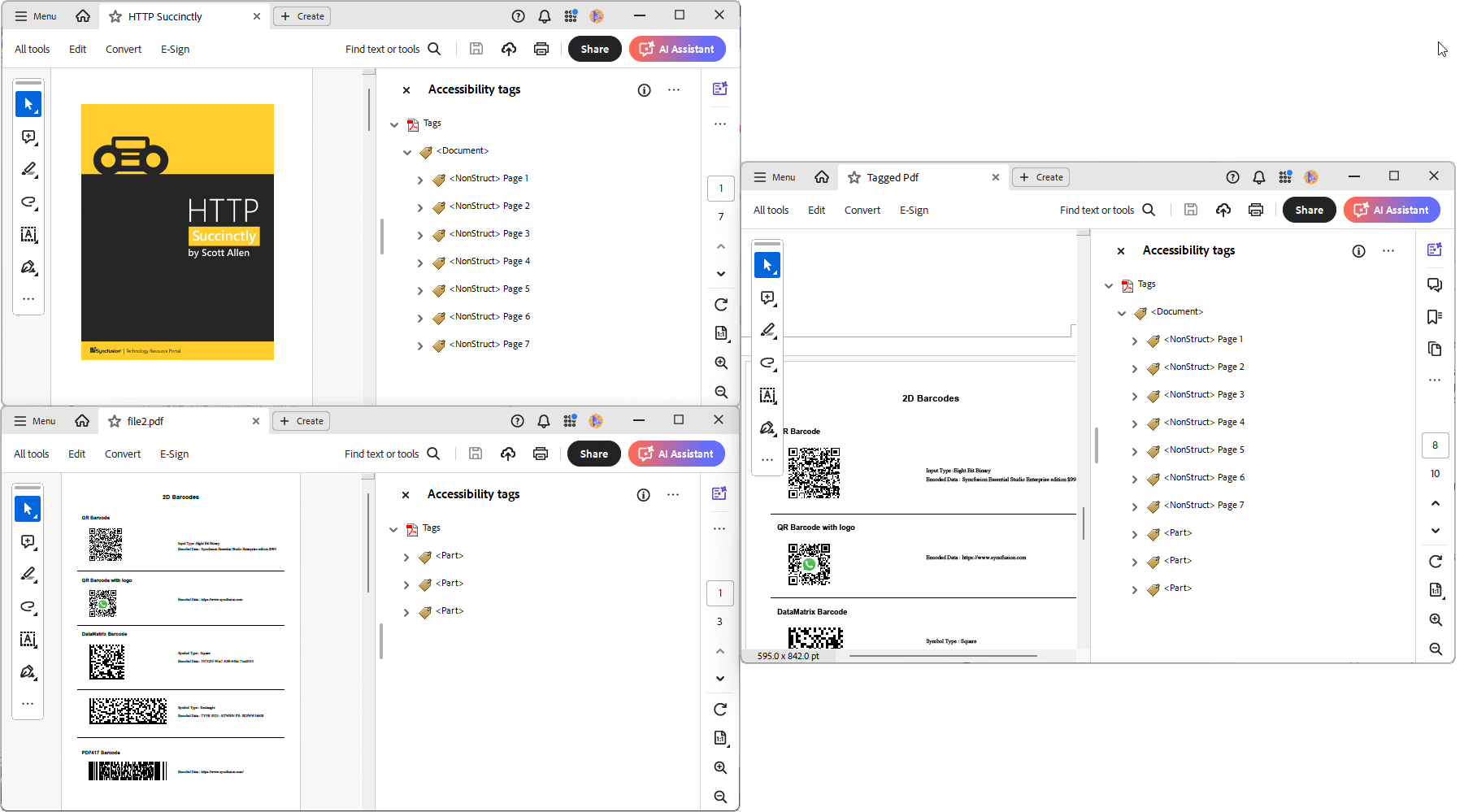
Add bookmarks to the merged PDF documents for easier navigation
Adding bookmarks to the merged PDF documents enhances navigation by allowing users to jump to specific sections or pages within the document quickly. Bookmarks act as an interactive table of contents, making it easier to locate key content.
Using the Syncfusion PDF Library, users can create bookmarks based on individual file titles. This will help quickly navigate to specific sections of the merged file.
Steps to add bookmarks to merged PDF documents
- First, create a new PdfDocument instance to hold the merged PDF documents with bookmarks.
- Use FileStream objects to load the PDF files you want to merge. The loaded documents will be merged, and bookmarks will be added at the beginning of each document.
- Merge the loaded PDF files using the PdfDocumentBase.Merge method to combine them into the final document.
- To improve navigation, add bookmarks for each merged PDF document. In this example, bookmarks are added to represent the start of each document in the merged file.
- Finally, save the merged document with bookmarks to a FileStream, which ensures that the bookmarks will appear in the output PDF. Refer to the following code example.
// Create a new PDF document.
using (PdfDocument finalDocument = new PdfDocument())
{
// Load the first and second PDF documents.
using (FileStream firstStream = new FileStream("data/file1.pdf", FileMode.Open, FileAccess.Read))
using (FileStream secondStream = new FileStream("data/file2.pdf", FileMode.Open, FileAccess.Read))
{
// Load the stream into PdfLoadedDocument class.
using PdfLoadedDocument loadedDocument1 = new PdfLoadedDocument(firstStream);
using PdfLoadedDocument loadedDocument2 = new PdfLoadedDocument(secondStream);
// Merge the PDF documents.
PdfDocumentBase.Merge(finalDocument, new[] { loadedDocument1, loadedDocument2 });
// Create a bookmark for the first PDF file.
PdfBookmark bookmark1 = finalDocument.Bookmarks.Add("Chapter 1 - Barcodes");
// Set the destination page for the first bookmark.
bookmark1.Destination = new PdfDestination(finalDocument.Pages[0]);
// Set the location for the first bookmark.
bookmark1.Destination.Location = new PointF(20, 20);
// Create a bookmark for the second PDF file.
PdfBookmark bookmark2 = finalDocument.Bookmarks.Add("Chapter 2 - HTTP Succinctly");
// Set the destination page for the second bookmark (after the pages of the first PDF)
bookmark2.Destination = new PdfDestination(finalDocument.Pages[loadedDocument1.PageCount]);
// Set the location for the second bookmark.
bookmark2.Destination.Location = new PointF(20, 20);
// Save the document with bookmarks into a stream.
using (FileStream outputFileStream = new FileStream("add-bookmarks.pdf", FileMode.Create, FileAccess.ReadWrite))
{
finalDocument.Save(outputFileStream);
}
}
}
When you execute this code, the output will be a merged PDF file named add-bookmarks.pdf. The document will include bookmarks representing each appended PDF, enabling easy navigation through the content using a bookmark panel in the PDF viewer.
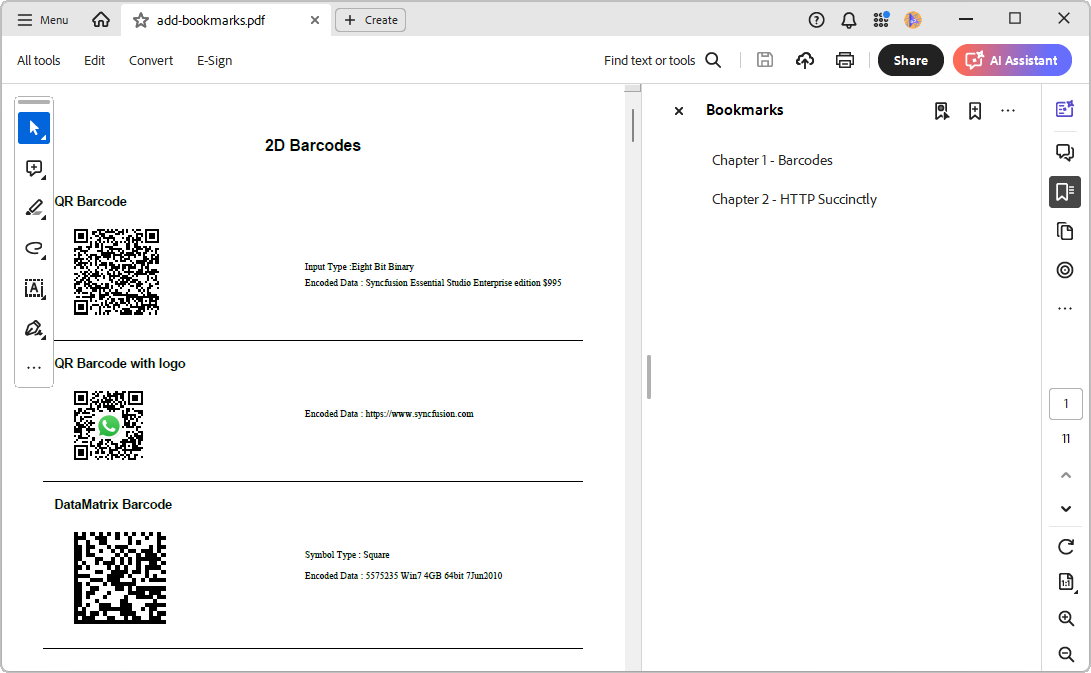
GitHub reference
For more details, refer to the 6 ways to merge PDF files using the .NET PDF Library and C# GitHub demo.

Syncfusion’s high-performance PDF Library allows you to create PDF documents from scratch without Adobe dependencies.
Conclusion
Thank you for reading! In this blog, we’ve explored how to merge PDF files using the Syncfusion .NET PDF Library in C#. We encourage you to dive deeper into the documentation, where you’ll find even more options and features, complete with code examples.
If you have any queries regarding these functionalities, kindly let us know by leaving a comment below. You can also reach out to us through our support forum, support portal, or feedback portal. We are always delighted to help you!
Related blogs
- How to Split PDF in C#
- 9 Types of Useful Data You Can Extract from a PDF Using C#
- Add, Remove, Extract, and Replace Images in PDF using C#
- Create Accessible PDF Documents Using C#
- Easily Create, Update, and Remove Bookmarks in PDFs Using C#
- Create, Fill, and Edit Fillable PDF Forms Using C# – A Complete Guide