TL;DR: Let’s see how to export data from Syncfusion WPF DataGrid to a PDF. This blog covers the essential steps, such as installing Syncfusion NuGet packages, binding data to the grid, and adding an export button. The export functionality includes custom headers/footers, column exclusions, and styling options. For detailed instructions, follow the complete guide!
Exporting data to PDF format is a common requirement in modern desktop applications, especially when working with business and reporting features. In this blog, we’ll explore how to seamlessly export data from the Syncfusion WPF DataGrid to a PDF file.
The WPF DataGrid is a highly customizable control designed to handle large volumes of data with ease. Its built-in support for exporting data to various formats, including PDF, makes it an excellent choice for WPF developers.
Note: If you are new to the DataGrid control, refer to its getting started documentation.
Key features of PDF export feature in WPF DataGrid
Before diving into the implementation, let’s explore the key features of the PDF export in WPF DataGrid:
- Customizable content: Export columns selectively, include custom headers/footers, and format content.
- Styling options: Apply styles to rows and cells to match your requirements.
- AutoFit columns: Automatically adjust column widths to fit content.
- Embedded graphics: Include images, charts, or logos in the exported document.
Export a simple WPF DataGrid
Step 1: Include the required Syncfusion references
Install the Syncfusion.DataGridExcelExport.Wpf NuGet package.
Step 2: Add the DataGrid to your WPF app
Refer to the following code example to add the WPF DataGrid to the app.
<syncfusion:SfDataGrid x:Name="sfDataGrid" Grid.Column="0" Grid.Row="0" ItemsSource="{Binding Orders}" AutoGenerateColumns="True" ColumnSizer="Star" />
In the MainWindow.xaml.cs file, bind the DataGrid to a collection.
using System.Collections.ObjectModel; public class Order { public int OrderID { get; set; } public string CustomerName { get; set; } public int Quantity { get; set; } public double Price { get; set; } } public class ViewModel { public ObservableCollection<Order> Orders { get; set; } public ViewModel() { Orders = new ObservableCollection<Order> { new Order { OrderID = 1001, CustomerName = "Michael Johnson", Quantity = 10, Price = 250.00 }, new Order { OrderID = 1002, CustomerName = "Emily Davis", Quantity = 5, Price = 100.00 }, new Order { OrderID = 1003, CustomerName = "Christopher Brown", Quantity = 12, Price = 300.00 }, new Order { OrderID = 1004, CustomerName = "Sarah Taylor", Quantity = 8, Price = 200.00 }, new Order { OrderID = 1005, CustomerName = "James Miller", Quantity = 15, Price = 375.00 }, new Order { OrderID = 1006, CustomerName = "Olivia Wilson", Quantity = 20, Price = 500.00 }, new Order { OrderID = 1007, CustomerName = "Benjamin Moore", Quantity = 25, Price = 625.00 }, new Order { OrderID = 1008, CustomerName = "Sophia Anderson", Quantity = 30, Price = 750.00 }, new Order { OrderID = 1009, CustomerName = "Alexander Thomas", Quantity = 18, Price = 450.00 }, new Order { OrderID = 1010, CustomerName = "Isabella Martinez", Quantity = 7, Price = 175.00 }, new Order { OrderID = 1011, CustomerName = "Daniel Harris", Quantity = 14, Price = 350.00 }, new Order { OrderID = 1012, CustomerName = "Victoria Martin", Quantity = 22, Price = 550.00 }, new Order { OrderID = 1013, CustomerName = "Matthew White", Quantity = 16, Price = 400.00 }, new Order { OrderID = 1014, CustomerName = "Emma Thompson", Quantity = 24, Price = 600.00 }, new Order { OrderID = 1015, CustomerName = "Liam Garcia", Quantity = 9, Price = 225.00 }, new Order { OrderID = 1016, CustomerName = "Ava Clark", Quantity = 13, Price = 325.00 }, new Order { OrderID = 1017, CustomerName = "Noah Lewis", Quantity = 21, Price = 525.00 }, new Order { OrderID = 1018, CustomerName = "Charlotte Young", Quantity = 11, Price = 275.00 }, new Order { OrderID = 1019, CustomerName = "Ethan Walker", Quantity = 19, Price = 475.00 }, new Order { OrderID = 1020, CustomerName = "Amelia Hall", Quantity = 17, Price = 425.00 }, new Order { OrderID = 1021, CustomerName = "Lucas Allen", Quantity = 6, Price = 150.00 }, new Order { OrderID = 1022, CustomerName = "Mia Scott", Quantity = 28, Price = 700.00 }, new Order { OrderID = 1023, CustomerName = "William Wright", Quantity = 23, Price = 575.00 }, new Order { OrderID = 1024, CustomerName = "Harper Adams", Quantity = 4, Price = 100.00 }, new Order { OrderID = 1025, CustomerName = "Ella Baker", Quantity = 3, Price = 75.00 } }; } }
Step 3: Implement the export functionality
We need to add a button(Export to PDF) to the user interface to allow users to export data from the WPF DataGrid to a PDF file. This button will act as the trigger for export functionality.
Refer to the following code example to define the button on your XAML page.
<Button Content="Export to PDF" Width="120" Height="30" VerticalAlignment="Top" HorizontalAlignment="Right" Margin="10" Click=" OnExportToPDFClick _Click"/>
The button click event is where the core logic for exporting the SfDataGrid to a PDF file is implemented. Refer to the following code example for the OnExportToPDFClick event handler, which is triggered when the Export to PDF button is clicked.
private void OnExportToPDFClick(object sender, RoutedEventArgs e) { // Create a PDF document instance PdfDocument pdfDocument = new PdfDocument(); // Export SfDataGrid to PDF PdfExportingOptions exportingOptions = new PdfExportingOptions() { }; // Export data to the PDF document pdfDocument = sfDataGrid.ExportToPdf(exportingOptions); // Save the PDF to a file pdfDocument.Save("SfDataGridExport.pdf"); pdfDocument.Close(true); try { // Use Process.Start to open the PDF file in the default PDF viewer Process.Start(new ProcessStartInfo("SfDataGridExport.pdf") { UseShellExecute = true }); } catch (System.Exception ex) { // Handle other exceptions MessageBox.Show($"An error occurred: {ex.Message}", "Error", MessageBoxButton.OK, MessageBoxImage.Error); } }
Refer to the following image.
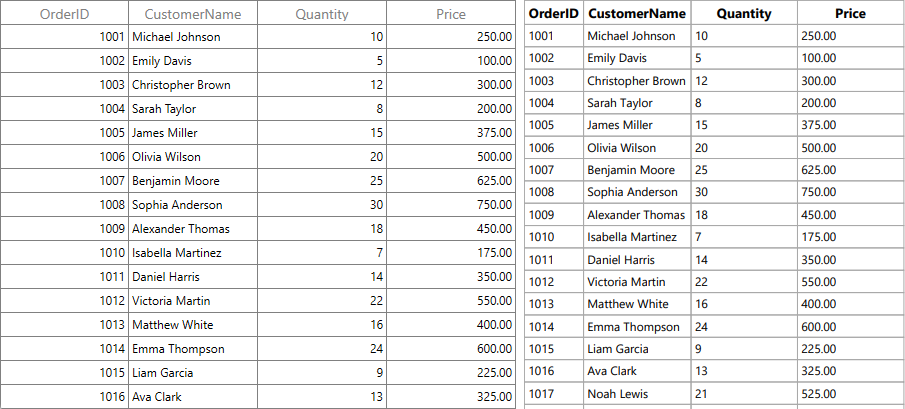
Advanced customization
Add custom headers and footers
When exporting data from the WPF DataGrid to a PDF, you can enhance the document by adding custom headers or footers. The headers and footers are an excellent way to include additional context, branding, or formatting to your exported documents. This functionality is enabled through the PageHeaderFooterEventHandler in the PdfExportingOptions.
With SfDataGrid, you can include the following as headers or footers:
- Text: Titles, subtitles, or descriptions.
- Images: Logos or banners.
- Graphics: Lines, shapes, or custom designs.
The PdfHeaderFooterEventHandler is used to define the content of the header and footer. By setting the PdfPageTemplateElement to PdfHeaderFooterEventArgs.PdfDocumentTemplate.Top or Bottom, you can customize the appearance of the header or footer on every page.
// Export SfDataGrid to PDF PdfExportingOptions exportingOptions = new PdfExportingOptions() { PageHeaderFooterEventHandler = PdfHeaderFooterEventHandler }; // Export data to the PDF document pdfDocument = sfDataGrid.ExportToPdf(exportingOptions); // Save the PDF to a file pdfDocument.Save("SfDataGridExport.pdf"); pdfDocument.Close(true); // Define the header customization logic static void PdfHeaderFooterEventHandler(object sender, PdfHeaderFooterEventArgs e) { // Create a bold font for the header text PdfFont font = new PdfStandardFont(PdfFontFamily.TimesRoman, 20f, PdfFontStyle.Bold); // Get the page width to ensure proper alignment var width = e.PdfPage.GetClientSize().Width; // Create a template element for the header with a fixed height PdfPageTemplateElement header = new PdfPageTemplateElement(width, 38); // Add text to the header at a specific position header.Graphics.DrawString("Order Details", font, PdfPens.Black, 70, 3); // Assign the header content to the top of the page e.PdfDocumentTemplate.Top = header; }
Refer to the following image.
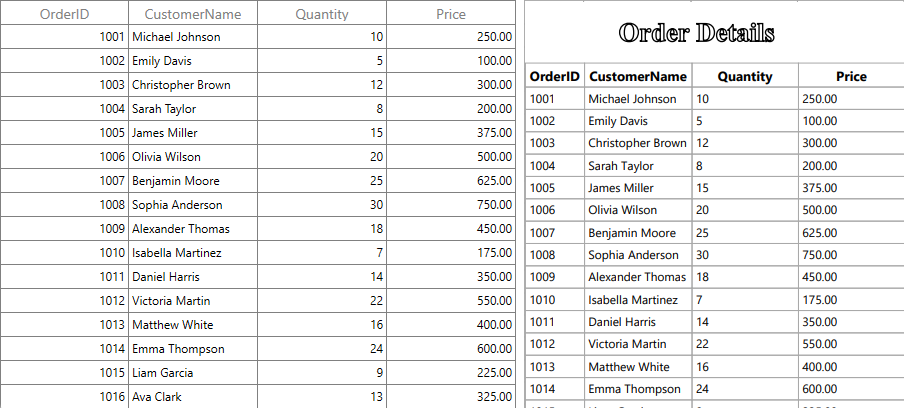
Exclude columns while exporting
You can easily export the WPF DataGrid to a PDF while excluding specific columns by utilizing the ExcludeColumns property.
This feature is particularly useful in scenarios where you need to generate professional reports or share grid data with clients while omitting certain columns (such as internal IDs, sensitive information, or calculated values) that are not relevant to the final output.
// Export SfDataGrid to PDF PdfExportingOptions exportingOptions = new PdfExportingOptions() { }; exportingOptions.ExcludeColumns.Add("Quantity"); // Export data to the PDF document pdfDocument = sfDataGrid.ExportToPdf(exportingOptions); // Save the PDF to a file pdfDocument.Save("SfDataGridExport.pdf"); pdfDocument.Close(true);Refer to the following image.
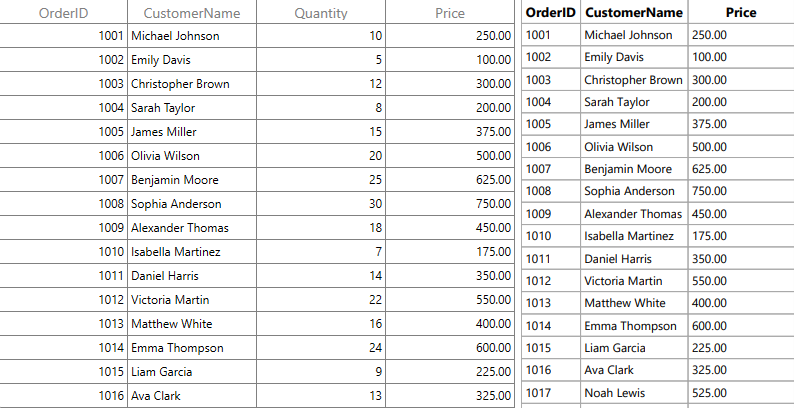
Exporting selected rows
By default, the entire content of WPF DataGrid is exported to a PDF document. However, if you need to export only specific rows, you can achieve this by passing the SelectedItems collection to the ExportToPdf or ExportToPdfGrid methods. This feature is particularly useful for generating reports or exporting only relevant data without including the entire data.
Refer to the following code example.
// Create a PDF document instance PdfDocument pdfDocument = new PdfDocument(); // Export SfDataGrid to PDF PdfExportingOptions exportingOptions = new PdfExportingOptions() { }; // Export data to the PDF document pdfDocument = sfDataGrid.ExportToPdf(sfDataGrid.SelectedItems, exportingOptions); // Save the PDF to a file pdfDocument.Save("SfDataGridExport.pdf"); pdfDocument.Close(true);
Refer to the following image.
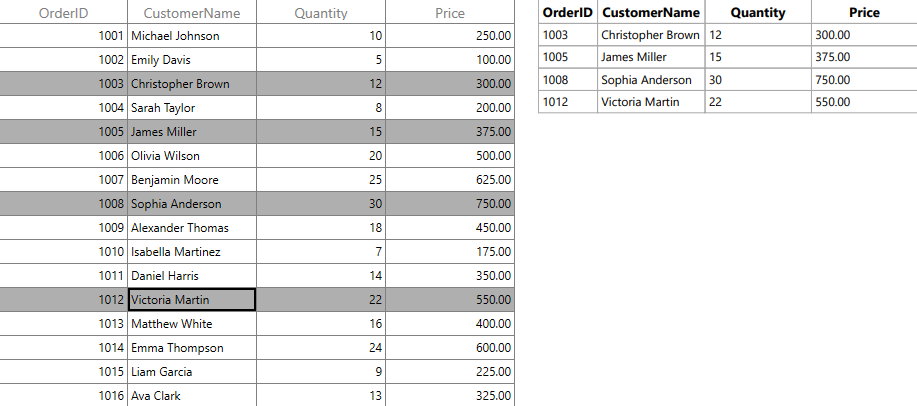
Exclude groups while exporting
By default, when exporting a DataGrid to a PDF, all the groups present in the DataGrid will be included in the exported file. However, if you prefer to export the data without including the groups, you can easily achieve this by setting the ExportGroups property to false.
This gives you complete control over how your data appears in the PDF, enabling a cleaner export when groupings are not required.
// Export SfDataGrid to PDF PdfExportingOptions exportingOptions = new PdfExportingOptions() { ExportGroups = false }; // Export data to the PDF document pdfDocument = sfDataGrid.ExportToPdf(exportingOptions); // Save the PDF to a file pdfDocument.Save("SfDataGridExport.pdf"); pdfDocument.Close(true);
Refer to the following image.
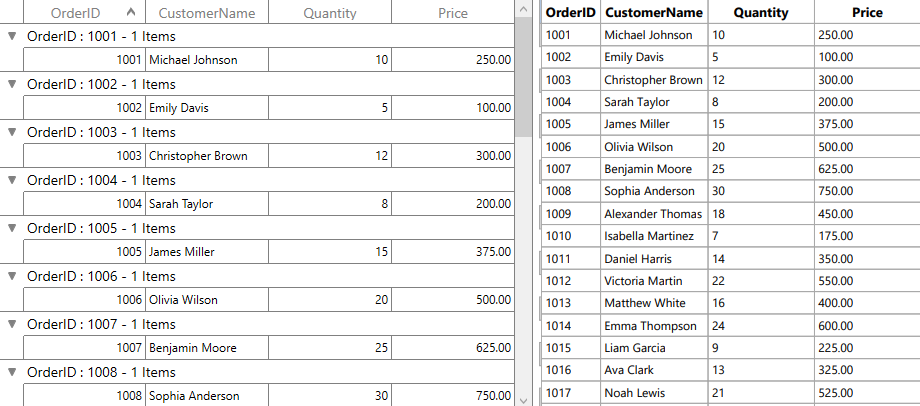
Apply styles to exported content
When exporting data from DataGrid to PDF, you can tailor the appearance of different cell types (e.g., header cells, group caption cells, and record cells) to enhance the document’s readability and professionalism. This customization is achieved by handling the ExportingEventHandler.
The following code demonstrates how to customize cell styles based on the cell type during export.
// Export SfDataGrid to PDF PdfExportingOptions exportingOptions = new PdfExportingOptions() { ExportingEventHandler = GridPdfExportingEventHandler }; // Export data to the PDF document pdfDocument = sfDataGrid.ExportToPdf(exportingOptions); // Save the PDF to a file pdfDocument.Save("SfDataGridExport.pdf"); pdfDocument.Close(true); void GridPdfExportingEventHandler(object sender, GridPdfExportingEventArgs e) { if (e.CellType == ExportCellType.HeaderCell) e.CellStyle.BackgroundBrush = PdfBrushes.LightSteelBlue; else if (e.CellType == ExportCellType.GroupCaptionCell) e.CellStyle.BackgroundBrush = PdfBrushes.LightGray; else if (e.CellType == ExportCellType.RecordCell) e.CellStyle.BackgroundBrush = PdfBrushes.Wheat; }
Refer to the following image.
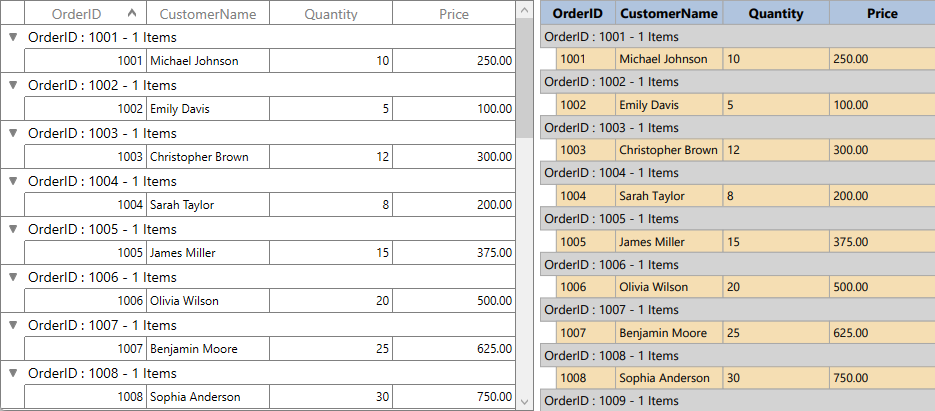
References
For more details, refer to Export WPF DataGrid to PDF documentation and GitHub demo.
Conclusion
Thanks for reading! Exporting data from Syncfusion WPF DataGrid to PDF is simple and flexible. It provides a comprehensive solution to meet diverse requirements with features like customizable headers, styling, and autofitting columns. Try it in your project to easily deliver polished reports and data presentations. We encourage you to try these steps and share your feedback in the comments below.
Our customers can access the latest version of Essential Studio for WPF from the License and Downloads page. If you are not a Syncfusion customer, you can download our free evaluation to explore all our controls.
For questions, you can contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!