TL;DR: Let’s see how to integrate CEL and SpEL queries in the Angular Query Builder. This blog covers how to set up the Query Builder, leverage CEL and SpEL for complex database queries, and filter records effectively. You’ll also learn to switch between query types using utility methods and radio buttons, providing greater flexibility for query management.
Syncfusion Angular Query Builder is a graphical user interface component that creates queries. It supports data binding, templates, importing from JSON and SQL, exporting to JSON and SQL, and parsing queries to predicates for the data manager. This component can be populated using an array of JavaScript objects.
It is a powerful tool for crafting complex database queries. However, by default, it primarily supports SQL and Mongo queries.
In this blog, we’ll guide you on retrieving CEL and SpEL queries from the Angular Query Builder to filter records from tables using these advanced filters.
First, let’s briefly define these query types.
CEL query
CEL (Common Expression Language) is a language-agnostic tool used to define and evaluate expressions. It is designed to be simple and efficient, making it suitable for various apps such as policy evaluation, data validation, and other scenarios where safe evaluation of expressions is required.
Here’s an example of a CEL query:
FirstName.startsWith("Andre") && LastName in ["Davolio", "Buchanan"] && Age > 29
SpEL query
SpEL (Spring Expression Language) is a powerful expression language within the Spring Framework. It is mainly used for configuring and manipulating beans in the Spring IoC (Inversion of Control) container, defining conditions in Spring Security, and more.
Here’s an example of a SpEL query:
FirstName matches "^Andre" and (LastName == "Davolio" or LastName == "Buchanan") and Age > 29
Getting started with Angular Query Builder
Integrating a Query Builder into your Angular app enhances the user experience by simplifying the query creation process. To begin, follow the instructions in the getting started with Angular Query Builder documentation.
CEL and SpEL query building in Angular Query Builder
To obtain CEL and SpEL queries, utilize the utility methods getCELQuery and getSpELQuery. These methods can be imported from the utility (util.ts) file.
To display the CEL and SpEL queries, add a TextArea and two Radio Buttons to switch between the CEL and SpEL query types. Additionally, incorporate the created and ruleChange event to update the query whenever a rule is modified in the Query Builder.
Here’s the step-by-step procedure for preparing the project.
Step 1: Create a Query Builder component with the created and ruleChange events to update the CEL and SpEL queries in the TextArea.
app.component.html
<div class="parent-container"> <div class='top-content'> <ejs-querybuilder id="querybuilder" [dataSource]="dataSource" #querybuilder [columns]="filter" width="100%" [rule]="importRules" (created)="updateRule()" (ruleChange)="updateRule()"> </ejs-querybuilder> </div> </div>
app.component.ts
import { Component, ViewChild } from '@angular/core'; import { ColumnsModel, QueryBuilderComponent, RuleModel } from '@syncfusion/ej2-angular-querybuilder'; import { employeeData } from './data-source'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { @ViewChild('querybuilder') qryBldrObj: QueryBuilderComponent | any; dataSource: any = employeeData; filter: ColumnsModel[] = [ { field: "EmployeeID", label: "Employee ID", type: "number" }, { field: "FirstName", label: "First Name", type: "string" }, { field: "LastName", label: "Last Name", type: "string" }, { field: "Age", label: "Age", type: "number" }, { field: "IsDeveloper", label: "Is Developer", type: "boolean" }, { field: "PrimaryFramework", label: "Primary Framework", type: "string" }, { field: "HireDate", label: "Hire Date", type: "date", format: "MM/dd/yyyy" }, { field: "Country", label: "Country", type: "string" }, ]; importRules: RuleModel = { condition: "and", rules: [ { label: "First Name", field: "FirstName", type: "string", operator: "startswith", value: "Andre" }, { label: "Last Name", field: "LastName", type: "string", operator: "in", value: ['Davolio', 'Buchanan'] }, { label: "Age", field: "Age", type: "number", operator: "greaterthan", value: 29 }, { condition: "or", rules: [ { label: "Is Developer", field: "IsDeveloper", type: "boolean", operator: "equal", value: true }, { label: "Primary Framework", field: "PrimaryFramework", type: "string", operator: "equal", value: "React" } ] } ], }; updateRule(): void { // Update the text area with CEL and SpEL. } }
Step 2: Add two Radio Buttons and a TextArea component to display the CEL and SpEL queries by importing the utility methods.
app.component.html
<div class="parent-container"> // Render Query Builder component. <div class="bottom-content"> <div class="e-query-preview"> <ejs-radiobutton (change)="change($event)" label="CEL" checked="true" name="query" value="cel"></ejs-radiobutton> <ejs-radiobutton style="margin-left: 20px" label="SpEL" (change)="change($event)" name="query" value="spel"></ejs-radiobutton> <textarea class="content-area" id="content-area"></textarea> </div> </div> </div>
app.component.ts
import { getCELQuery, getSpELQuery } from './util'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { // Query Builder related codes. queryType: string = 'cel'; change(args: any){ this.queryType = args.value; this.updateRule(); } updateRule(): void { if (this.queryType === "cel") { this.convertCELQuery(); } else { this.convertSpELQuery(); } } convertCELQuery(): void { const allRules = this.qryBldrObj.getValidRules(); let celQuery: string = ''; celQuery = getCELQuery(allRules, celQuery); document.querySelector('.content-area')!.textContent = celQuery; } convertSpELQuery(): void { let spELQuery: string = ''; const allRules = this.qryBldrObj.getValidRules(); spELQuery = getSpELQuery(allRules, spELQuery); document.querySelector('.content-area')!.textContent = spELQuery; }
app.module.ts
import { QueryBuilderModule } from '@syncfusion/ej2-angular-querybuilder'; import { RadioButtonAllModule } from '@syncfusion/ej2-angular-buttons'; @NgModule({ imports: [ RadioButtonAllModule, QueryBuilderModule ] })
Refer to the following output image.
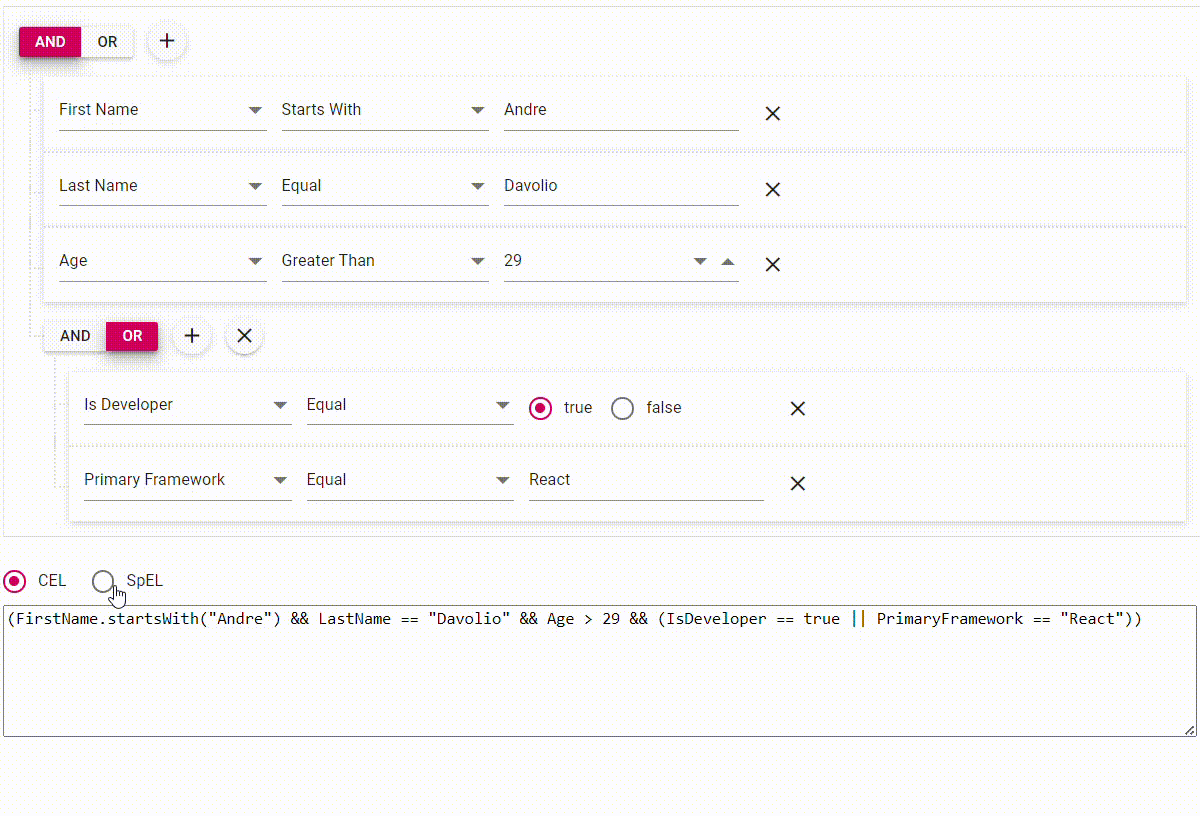
Reference
You can also download the complete code example for integrating CEL and SpEL queries in Angular Query Builder on StackBlitz.
Conclusion
Thanks for reading! In this blog, we’ve explored the CEL and SpEL queries and how to integrate them in the Syncfusion Angular Query Builder to filter the records from the tables.
Whether you’re already a valued Syncfusion user or new to our platform, we extend an invitation to explore our Angular components with the convenience of a free trial. This trial allows you to experience the full potential of our components to enhance your app’s user interface and functionality.
Our dedicated support system is readily available if you need guidance or have any inquiries. You can contact us through our support forum, support portal, or feedback portal. Your success is our priority, and we’re always delighted to assist you on your development journey!
Related blogs
- How to Share Angular Code Between Projects?
- Explore Advanced PDF Exporting in Angular Pivot Table
- Enhancing Angular Charts: Boosting Readability with Dynamic Colors
- Advanced Query Building Techniques in Angular: Queries with Different Connectors